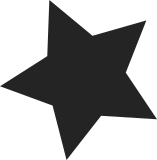
All the current architecture specific defines for these are the same. Refactor these common defines to a common header file. The new common linux/compat_time.h is also useful as it will eventually be used to hold all the defines that are needed for compat time types that support non y2038 safe types. New architectures need not have to define these new types as they will only use new y2038 safe syscalls. This file can be deleted after y2038 when we stop supporting non y2038 safe syscalls. The patch also requires an operation similar to: git grep "asm/compat\.h" | cut -d ":" -f 1 | xargs -n 1 sed -i -e "s%asm/compat.h%linux/compat.h%g" Cc: acme@kernel.org Cc: benh@kernel.crashing.org Cc: borntraeger@de.ibm.com Cc: catalin.marinas@arm.com Cc: cmetcalf@mellanox.com Cc: cohuck@redhat.com Cc: davem@davemloft.net Cc: deller@gmx.de Cc: devel@driverdev.osuosl.org Cc: gerald.schaefer@de.ibm.com Cc: gregkh@linuxfoundation.org Cc: heiko.carstens@de.ibm.com Cc: hoeppner@linux.vnet.ibm.com Cc: hpa@zytor.com Cc: jejb@parisc-linux.org Cc: jwi@linux.vnet.ibm.com Cc: linux-kernel@vger.kernel.org Cc: linux-mips@linux-mips.org Cc: linux-parisc@vger.kernel.org Cc: linuxppc-dev@lists.ozlabs.org Cc: linux-s390@vger.kernel.org Cc: mark.rutland@arm.com Cc: mingo@redhat.com Cc: mpe@ellerman.id.au Cc: oberpar@linux.vnet.ibm.com Cc: oprofile-list@lists.sf.net Cc: paulus@samba.org Cc: peterz@infradead.org Cc: ralf@linux-mips.org Cc: rostedt@goodmis.org Cc: rric@kernel.org Cc: schwidefsky@de.ibm.com Cc: sebott@linux.vnet.ibm.com Cc: sparclinux@vger.kernel.org Cc: sth@linux.vnet.ibm.com Cc: ubraun@linux.vnet.ibm.com Cc: will.deacon@arm.com Cc: x86@kernel.org Signed-off-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Deepa Dinamani <deepa.kernel@gmail.com> Acked-by: Steven Rostedt (VMware) <rostedt@goodmis.org> Acked-by: Catalin Marinas <catalin.marinas@arm.com> Acked-by: James Hogan <jhogan@kernel.org> Acked-by: Helge Deller <deller@gmx.de> Signed-off-by: Arnd Bergmann <arnd@arndb.de>
130 lines
2.5 KiB
C
130 lines
2.5 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* IOCTL interface for SCLP
|
|
*
|
|
* Copyright IBM Corp. 2012
|
|
*
|
|
* Author: Michael Holzheu <holzheu@linux.vnet.ibm.com>
|
|
*/
|
|
|
|
#include <linux/compat.h>
|
|
#include <linux/uaccess.h>
|
|
#include <linux/miscdevice.h>
|
|
#include <linux/gfp.h>
|
|
#include <linux/init.h>
|
|
#include <linux/ioctl.h>
|
|
#include <linux/fs.h>
|
|
#include <asm/sclp_ctl.h>
|
|
#include <asm/sclp.h>
|
|
|
|
#include "sclp.h"
|
|
|
|
/*
|
|
* Supported command words
|
|
*/
|
|
static unsigned int sclp_ctl_sccb_wlist[] = {
|
|
0x00400002,
|
|
0x00410002,
|
|
};
|
|
|
|
/*
|
|
* Check if command word is supported
|
|
*/
|
|
static int sclp_ctl_cmdw_supported(unsigned int cmdw)
|
|
{
|
|
int i;
|
|
|
|
for (i = 0; i < ARRAY_SIZE(sclp_ctl_sccb_wlist); i++) {
|
|
if (cmdw == sclp_ctl_sccb_wlist[i])
|
|
return 1;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
static void __user *u64_to_uptr(u64 value)
|
|
{
|
|
if (is_compat_task())
|
|
return compat_ptr(value);
|
|
else
|
|
return (void __user *)(unsigned long)value;
|
|
}
|
|
|
|
/*
|
|
* Start SCLP request
|
|
*/
|
|
static int sclp_ctl_ioctl_sccb(void __user *user_area)
|
|
{
|
|
struct sclp_ctl_sccb ctl_sccb;
|
|
struct sccb_header *sccb;
|
|
unsigned long copied;
|
|
int rc;
|
|
|
|
if (copy_from_user(&ctl_sccb, user_area, sizeof(ctl_sccb)))
|
|
return -EFAULT;
|
|
if (!sclp_ctl_cmdw_supported(ctl_sccb.cmdw))
|
|
return -EOPNOTSUPP;
|
|
sccb = (void *) get_zeroed_page(GFP_KERNEL | GFP_DMA);
|
|
if (!sccb)
|
|
return -ENOMEM;
|
|
copied = PAGE_SIZE -
|
|
copy_from_user(sccb, u64_to_uptr(ctl_sccb.sccb), PAGE_SIZE);
|
|
if (offsetof(struct sccb_header, length) +
|
|
sizeof(sccb->length) > copied || sccb->length > copied) {
|
|
rc = -EFAULT;
|
|
goto out_free;
|
|
}
|
|
if (sccb->length < 8) {
|
|
rc = -EINVAL;
|
|
goto out_free;
|
|
}
|
|
rc = sclp_sync_request(ctl_sccb.cmdw, sccb);
|
|
if (rc)
|
|
goto out_free;
|
|
if (copy_to_user(u64_to_uptr(ctl_sccb.sccb), sccb, sccb->length))
|
|
rc = -EFAULT;
|
|
out_free:
|
|
free_page((unsigned long) sccb);
|
|
return rc;
|
|
}
|
|
|
|
/*
|
|
* SCLP SCCB ioctl function
|
|
*/
|
|
static long sclp_ctl_ioctl(struct file *filp, unsigned int cmd,
|
|
unsigned long arg)
|
|
{
|
|
void __user *argp;
|
|
|
|
if (is_compat_task())
|
|
argp = compat_ptr(arg);
|
|
else
|
|
argp = (void __user *) arg;
|
|
switch (cmd) {
|
|
case SCLP_CTL_SCCB:
|
|
return sclp_ctl_ioctl_sccb(argp);
|
|
default: /* unknown ioctl number */
|
|
return -ENOTTY;
|
|
}
|
|
}
|
|
|
|
/*
|
|
* File operations
|
|
*/
|
|
static const struct file_operations sclp_ctl_fops = {
|
|
.owner = THIS_MODULE,
|
|
.open = nonseekable_open,
|
|
.unlocked_ioctl = sclp_ctl_ioctl,
|
|
.compat_ioctl = sclp_ctl_ioctl,
|
|
.llseek = no_llseek,
|
|
};
|
|
|
|
/*
|
|
* Misc device definition
|
|
*/
|
|
static struct miscdevice sclp_ctl_device = {
|
|
.minor = MISC_DYNAMIC_MINOR,
|
|
.name = "sclp",
|
|
.fops = &sclp_ctl_fops,
|
|
};
|
|
builtin_misc_device(sclp_ctl_device);
|