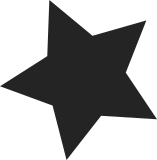
The SLAB kmalloc with a constant value isn't consistent with the other implementations because it bails out with __you_cannot_kmalloc_that_much rather than returning NULL and properly allowing the caller to fall back to vmalloc or take other action. This doesn't happen with a non-constant value or with SLOB or SLUB. Starting with 2.6.28, I've been seeing build failures on s390x. This is due to init_section_page_cgroup trying to allocate 2.5MB when the max size for a kmalloc on s390x is 2MB. It's failing because the value is constant. The workarounds at the call size are ugly and the caller shouldn't have to change behavior depending on what the backend of the API is. So, this patch eliminates the link failure and returns NULL like the other implementations. Signed-off-by: Jeff Mahoney <jeffm@suse.com> Cc: Martin Schwidefsky <schwidefsky@de.ibm.com> Cc: Heiko Carstens <heiko.carstens@de.ibm.com> Cc: Christoph Lameter <cl@linux-foundation.org> Cc: Pekka Enberg <penberg@cs.helsinki.fi> Cc: Matt Mackall <mpm@selenic.com> Cc: Nick Piggin <nickpiggin@yahoo.com.au> Cc: <stable@kernel.org> [2.6.28.x] Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Pekka Enberg <penberg@cs.helsinki.fi>
93 lines
2.1 KiB
C
93 lines
2.1 KiB
C
#ifndef _LINUX_SLAB_DEF_H
|
|
#define _LINUX_SLAB_DEF_H
|
|
|
|
/*
|
|
* Definitions unique to the original Linux SLAB allocator.
|
|
*
|
|
* What we provide here is a way to optimize the frequent kmalloc
|
|
* calls in the kernel by selecting the appropriate general cache
|
|
* if kmalloc was called with a size that can be established at
|
|
* compile time.
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <asm/page.h> /* kmalloc_sizes.h needs PAGE_SIZE */
|
|
#include <asm/cache.h> /* kmalloc_sizes.h needs L1_CACHE_BYTES */
|
|
#include <linux/compiler.h>
|
|
|
|
/* Size description struct for general caches. */
|
|
struct cache_sizes {
|
|
size_t cs_size;
|
|
struct kmem_cache *cs_cachep;
|
|
#ifdef CONFIG_ZONE_DMA
|
|
struct kmem_cache *cs_dmacachep;
|
|
#endif
|
|
};
|
|
extern struct cache_sizes malloc_sizes[];
|
|
|
|
void *kmem_cache_alloc(struct kmem_cache *, gfp_t);
|
|
void *__kmalloc(size_t size, gfp_t flags);
|
|
|
|
static inline void *kmalloc(size_t size, gfp_t flags)
|
|
{
|
|
if (__builtin_constant_p(size)) {
|
|
int i = 0;
|
|
|
|
if (!size)
|
|
return ZERO_SIZE_PTR;
|
|
|
|
#define CACHE(x) \
|
|
if (size <= x) \
|
|
goto found; \
|
|
else \
|
|
i++;
|
|
#include <linux/kmalloc_sizes.h>
|
|
#undef CACHE
|
|
return NULL;
|
|
found:
|
|
#ifdef CONFIG_ZONE_DMA
|
|
if (flags & GFP_DMA)
|
|
return kmem_cache_alloc(malloc_sizes[i].cs_dmacachep,
|
|
flags);
|
|
#endif
|
|
return kmem_cache_alloc(malloc_sizes[i].cs_cachep, flags);
|
|
}
|
|
return __kmalloc(size, flags);
|
|
}
|
|
|
|
#ifdef CONFIG_NUMA
|
|
extern void *__kmalloc_node(size_t size, gfp_t flags, int node);
|
|
extern void *kmem_cache_alloc_node(struct kmem_cache *, gfp_t flags, int node);
|
|
|
|
static inline void *kmalloc_node(size_t size, gfp_t flags, int node)
|
|
{
|
|
if (__builtin_constant_p(size)) {
|
|
int i = 0;
|
|
|
|
if (!size)
|
|
return ZERO_SIZE_PTR;
|
|
|
|
#define CACHE(x) \
|
|
if (size <= x) \
|
|
goto found; \
|
|
else \
|
|
i++;
|
|
#include <linux/kmalloc_sizes.h>
|
|
#undef CACHE
|
|
return NULL;
|
|
found:
|
|
#ifdef CONFIG_ZONE_DMA
|
|
if (flags & GFP_DMA)
|
|
return kmem_cache_alloc_node(malloc_sizes[i].cs_dmacachep,
|
|
flags, node);
|
|
#endif
|
|
return kmem_cache_alloc_node(malloc_sizes[i].cs_cachep,
|
|
flags, node);
|
|
}
|
|
return __kmalloc_node(size, flags, node);
|
|
}
|
|
|
|
#endif /* CONFIG_NUMA */
|
|
|
|
#endif /* _LINUX_SLAB_DEF_H */
|