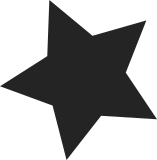
If a display controller is not attached to an explicit IOMMU domain, which usually means that it's connected to an IOMMU domain controlled by the DMA API, make sure to map the framebuffer to the display controller address space. This allows us to transparently handle setups where the display controller is attached to an IOMMU or setups where it isn't. It also allows the driver to work with a DMA API that is backed by an IOMMU. Signed-off-by: Thierry Reding <treding@nvidia.com>
81 lines
1.7 KiB
C
81 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* Copyright (C) 2017 NVIDIA CORPORATION. All rights reserved.
|
|
*/
|
|
|
|
#ifndef TEGRA_PLANE_H
|
|
#define TEGRA_PLANE_H 1
|
|
|
|
#include <drm/drm_plane.h>
|
|
|
|
struct tegra_bo;
|
|
struct tegra_dc;
|
|
|
|
struct tegra_plane {
|
|
struct drm_plane base;
|
|
struct tegra_dc *dc;
|
|
unsigned int offset;
|
|
unsigned int index;
|
|
};
|
|
|
|
struct tegra_cursor {
|
|
struct tegra_plane base;
|
|
|
|
struct tegra_bo *bo;
|
|
unsigned int width;
|
|
unsigned int height;
|
|
};
|
|
|
|
static inline struct tegra_plane *to_tegra_plane(struct drm_plane *plane)
|
|
{
|
|
return container_of(plane, struct tegra_plane, base);
|
|
}
|
|
|
|
struct tegra_plane_legacy_blending_state {
|
|
bool alpha;
|
|
bool top;
|
|
};
|
|
|
|
struct tegra_plane_state {
|
|
struct drm_plane_state base;
|
|
|
|
struct sg_table *sgt[3];
|
|
dma_addr_t iova[3];
|
|
|
|
struct tegra_bo_tiling tiling;
|
|
u32 format;
|
|
u32 swap;
|
|
|
|
bool bottom_up;
|
|
|
|
/* used for legacy blending support only */
|
|
struct tegra_plane_legacy_blending_state blending[2];
|
|
bool opaque;
|
|
};
|
|
|
|
static inline struct tegra_plane_state *
|
|
to_tegra_plane_state(struct drm_plane_state *state)
|
|
{
|
|
if (state)
|
|
return container_of(state, struct tegra_plane_state, base);
|
|
|
|
return NULL;
|
|
}
|
|
|
|
extern const struct drm_plane_funcs tegra_plane_funcs;
|
|
|
|
int tegra_plane_prepare_fb(struct drm_plane *plane,
|
|
struct drm_plane_state *state);
|
|
void tegra_plane_cleanup_fb(struct drm_plane *plane,
|
|
struct drm_plane_state *state);
|
|
|
|
int tegra_plane_state_add(struct tegra_plane *plane,
|
|
struct drm_plane_state *state);
|
|
|
|
int tegra_plane_format(u32 fourcc, u32 *format, u32 *swap);
|
|
bool tegra_plane_format_is_yuv(unsigned int format, bool *planar);
|
|
int tegra_plane_setup_legacy_state(struct tegra_plane *tegra,
|
|
struct tegra_plane_state *state);
|
|
|
|
#endif /* TEGRA_PLANE_H */
|