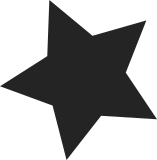
This defines a new platform_driver_probe() method allowing the driver's probe() method, and its support code+data, to safely live in __init sections for typical system configurations. Many system-on-chip processors could benefit from this API, to the tune of recovering hundreds to thousands of bytes per driver. That's memory which is currently wasted holding code which can never be called after system startup, yet can not be removed. It can't be removed because of the linkage requirement that pointers to init section code (like, ideally, probe support) must not live in other sections (like driver method tables) after those pointers would be invalid. Signed-off-by: David Brownell <dbrownell@users.sourceforge.net> Signed-off-by: Greg Kroah-Hartman <gregkh@suse.de>
71 lines
2.6 KiB
C
71 lines
2.6 KiB
C
/*
|
|
* platform_device.h - generic, centralized driver model
|
|
*
|
|
* Copyright (c) 2001-2003 Patrick Mochel <mochel@osdl.org>
|
|
*
|
|
* This file is released under the GPLv2
|
|
*
|
|
* See Documentation/driver-model/ for more information.
|
|
*/
|
|
|
|
#ifndef _PLATFORM_DEVICE_H_
|
|
#define _PLATFORM_DEVICE_H_
|
|
|
|
#include <linux/device.h>
|
|
|
|
struct platform_device {
|
|
const char * name;
|
|
u32 id;
|
|
struct device dev;
|
|
u32 num_resources;
|
|
struct resource * resource;
|
|
};
|
|
|
|
#define to_platform_device(x) container_of((x), struct platform_device, dev)
|
|
|
|
extern int platform_device_register(struct platform_device *);
|
|
extern void platform_device_unregister(struct platform_device *);
|
|
|
|
extern struct bus_type platform_bus_type;
|
|
extern struct device platform_bus;
|
|
|
|
extern struct resource *platform_get_resource(struct platform_device *, unsigned int, unsigned int);
|
|
extern int platform_get_irq(struct platform_device *, unsigned int);
|
|
extern struct resource *platform_get_resource_byname(struct platform_device *, unsigned int, char *);
|
|
extern int platform_get_irq_byname(struct platform_device *, char *);
|
|
extern int platform_add_devices(struct platform_device **, int);
|
|
|
|
extern struct platform_device *platform_device_register_simple(char *, unsigned int, struct resource *, unsigned int);
|
|
|
|
extern struct platform_device *platform_device_alloc(const char *name, unsigned int id);
|
|
extern int platform_device_add_resources(struct platform_device *pdev, struct resource *res, unsigned int num);
|
|
extern int platform_device_add_data(struct platform_device *pdev, void *data, size_t size);
|
|
extern int platform_device_add(struct platform_device *pdev);
|
|
extern void platform_device_del(struct platform_device *pdev);
|
|
extern void platform_device_put(struct platform_device *pdev);
|
|
|
|
struct platform_driver {
|
|
int (*probe)(struct platform_device *);
|
|
int (*remove)(struct platform_device *);
|
|
void (*shutdown)(struct platform_device *);
|
|
int (*suspend)(struct platform_device *, pm_message_t state);
|
|
int (*suspend_late)(struct platform_device *, pm_message_t state);
|
|
int (*resume_early)(struct platform_device *);
|
|
int (*resume)(struct platform_device *);
|
|
struct device_driver driver;
|
|
};
|
|
|
|
extern int platform_driver_register(struct platform_driver *);
|
|
extern void platform_driver_unregister(struct platform_driver *);
|
|
|
|
/* non-hotpluggable platform devices may use this so that probe() and
|
|
* its support may live in __init sections, conserving runtime memory.
|
|
*/
|
|
extern int platform_driver_probe(struct platform_driver *driver,
|
|
int (*probe)(struct platform_device *));
|
|
|
|
#define platform_get_drvdata(_dev) dev_get_drvdata(&(_dev)->dev)
|
|
#define platform_set_drvdata(_dev,data) dev_set_drvdata(&(_dev)->dev, (data))
|
|
|
|
#endif /* _PLATFORM_DEVICE_H_ */
|