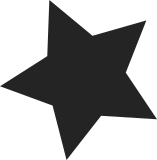
buffer.h supplies everything needed for devices using buffers. buffer_impl.h supplies access to the internals as needed to write a buffer implementation. This was really motivated by the mess that turned up in the kernel-doc documentation pulled in by the new sphinx docs. It made it clear that our logical separations in headers were generally terrible. The buffer case was easy to sort out without greatly effecting drivers so here it is. Signed-off-by: Jonathan Cameron <jic23@kernel.org> Reviewed-by: Lars-Peter Clausen <lars@metafoo.de>
54 lines
1.7 KiB
C
54 lines
1.7 KiB
C
/* The industrial I/O core - generic buffer interfaces.
|
|
*
|
|
* Copyright (c) 2008 Jonathan Cameron
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published by
|
|
* the Free Software Foundation.
|
|
*/
|
|
|
|
#ifndef _IIO_BUFFER_GENERIC_H_
|
|
#define _IIO_BUFFER_GENERIC_H_
|
|
#include <linux/sysfs.h>
|
|
#include <linux/iio/iio.h>
|
|
|
|
struct iio_buffer;
|
|
|
|
void iio_buffer_set_attrs(struct iio_buffer *buffer,
|
|
const struct attribute **attrs);
|
|
|
|
int iio_push_to_buffers(struct iio_dev *indio_dev, const void *data);
|
|
|
|
/**
|
|
* iio_push_to_buffers_with_timestamp() - push data and timestamp to buffers
|
|
* @indio_dev: iio_dev structure for device.
|
|
* @data: sample data
|
|
* @timestamp: timestamp for the sample data
|
|
*
|
|
* Pushes data to the IIO device's buffers. If timestamps are enabled for the
|
|
* device the function will store the supplied timestamp as the last element in
|
|
* the sample data buffer before pushing it to the device buffers. The sample
|
|
* data buffer needs to be large enough to hold the additional timestamp
|
|
* (usually the buffer should be indio->scan_bytes bytes large).
|
|
*
|
|
* Returns 0 on success, a negative error code otherwise.
|
|
*/
|
|
static inline int iio_push_to_buffers_with_timestamp(struct iio_dev *indio_dev,
|
|
void *data, int64_t timestamp)
|
|
{
|
|
if (indio_dev->scan_timestamp) {
|
|
size_t ts_offset = indio_dev->scan_bytes / sizeof(int64_t) - 1;
|
|
((int64_t *)data)[ts_offset] = timestamp;
|
|
}
|
|
|
|
return iio_push_to_buffers(indio_dev, data);
|
|
}
|
|
|
|
bool iio_validate_scan_mask_onehot(struct iio_dev *indio_dev,
|
|
const unsigned long *mask);
|
|
|
|
void iio_device_attach_buffer(struct iio_dev *indio_dev,
|
|
struct iio_buffer *buffer);
|
|
|
|
#endif /* _IIO_BUFFER_GENERIC_H_ */
|