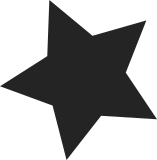
When XIP_KERNEL is enabled, some functions are defined in the .data ELF section because we require them to be in RAM whenever we communicate with the flash chip. However this causes problems when FTRACE is enabled and gcc emits calls to __gnu_mcount_nc in the function prolog: drivers/built-in.o: In function `cfi_chip_setup': :(.data+0x272fc): relocation truncated to fit: R_ARM_CALL against symbol `__gnu_mcount_nc' defined in .text section in arch/arm/kernel/built-in.o drivers/built-in.o: In function `cfi_probe_chip': :(.data+0x27de8): relocation truncated to fit: R_ARM_CALL against symbol `__gnu_mcount_nc' defined in .text section in arch/arm/kernel/built-in.o /tmp/ccY172rP.s: Assembler messages: /tmp/ccY172rP.s:70: Warning: ignoring changed section attributes for .data /tmp/ccY172rP.s: Error: 1 warning, treating warnings as errors make[5]: *** [drivers/mtd/chips/cfi_probe.o] Error 1 /tmp/ccK4rjeO.s: Assembler messages: /tmp/ccK4rjeO.s:421: Warning: ignoring changed section attributes for .data /tmp/ccK4rjeO.s: Error: 1 warning, treating warnings as errors make[5]: *** [drivers/mtd/chips/cfi_util.o] Error 1 /tmp/ccUvhCYR.s: Assembler messages: /tmp/ccUvhCYR.s:1895: Warning: ignoring changed section attributes for .data /tmp/ccUvhCYR.s: Error: 1 warning, treating warnings as errors Specifically, this does not work because the .data section is not marked executable, which leads LD to not generate trampolines for long calls. This moves the __xipram functions into their own .xiptext section instead. The section is still placed next to .data and located in RAM but is marked executable, which avoids the build errors. Also, we only need to place the XIP functions into a separate section if both CONFIG_XIP_KERNEL and CONFIG_MTD_XIP are set: When only MTD_XIP is used, the whole kernel is still in RAM and we do not need to worry about pulling out the rug under it. When only XIP_KERNEL but not MTD_XIP is set, the kernel is in some form of ROM, but we never write to it. Note that MTD_XIP has been broken on ARM since around 2011 or 2012. I have sent another patch[2] to fix compilation, which I plan to merge through arm-soc unless there are objections. The obvious alternative to that would be to completely rip out the MTD_XIP support from the kernel, since obviously nobody has been using it in a long while. Link: [1] https://patchwork.kernel.org/patch/8109771/ Link: [2] https://patchwork.kernel.org/patch/9855225/ Signed-off-by: Arnd Bergmann <arnd@arndb.de> Signed-off-by: Boris Brezillon <boris.brezillon@free-electrons.com>
102 lines
2.7 KiB
C
102 lines
2.7 KiB
C
/*
|
|
* MTD primitives for XIP support
|
|
*
|
|
* Author: Nicolas Pitre
|
|
* Created: Nov 2, 2004
|
|
* Copyright: (C) 2004 MontaVista Software, Inc.
|
|
*
|
|
* This XIP support for MTD has been loosely inspired
|
|
* by an earlier patch authored by David Woodhouse.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#ifndef __LINUX_MTD_XIP_H__
|
|
#define __LINUX_MTD_XIP_H__
|
|
|
|
|
|
#ifdef CONFIG_MTD_XIP
|
|
|
|
/*
|
|
* We really don't want gcc to guess anything.
|
|
* We absolutely _need_ proper inlining.
|
|
*/
|
|
#include <linux/compiler.h>
|
|
|
|
/*
|
|
* Function that are modifying the flash state away from array mode must
|
|
* obviously not be running from flash. The __xipram is therefore marking
|
|
* those functions so they get relocated to ram.
|
|
*/
|
|
#ifdef CONFIG_XIP_KERNEL
|
|
#define __xipram noinline __attribute__ ((__section__ (".xiptext")))
|
|
#endif
|
|
|
|
/*
|
|
* Each architecture has to provide the following macros. They must access
|
|
* the hardware directly and not rely on any other (XIP) functions since they
|
|
* won't be available when used (flash not in array mode).
|
|
*
|
|
* xip_irqpending()
|
|
*
|
|
* return non zero when any hardware interrupt is pending.
|
|
*
|
|
* xip_currtime()
|
|
*
|
|
* return a platform specific time reference to be used with
|
|
* xip_elapsed_since().
|
|
*
|
|
* xip_elapsed_since(x)
|
|
*
|
|
* return in usecs the elapsed timebetween now and the reference x as
|
|
* returned by xip_currtime().
|
|
*
|
|
* note 1: conversion to usec can be approximated, as long as the
|
|
* returned value is <= the real elapsed time.
|
|
* note 2: this should be able to cope with a few seconds without
|
|
* overflowing.
|
|
*
|
|
* xip_iprefetch()
|
|
*
|
|
* Macro to fill instruction prefetch
|
|
* e.g. a series of nops: asm volatile (".rep 8; nop; .endr");
|
|
*/
|
|
|
|
#include <asm/mtd-xip.h>
|
|
|
|
#ifndef xip_irqpending
|
|
|
|
#warning "missing IRQ and timer primitives for XIP MTD support"
|
|
#warning "some of the XIP MTD support code will be disabled"
|
|
#warning "your system will therefore be unresponsive when writing or erasing flash"
|
|
|
|
#define xip_irqpending() (0)
|
|
#define xip_currtime() (0)
|
|
#define xip_elapsed_since(x) (0)
|
|
|
|
#endif
|
|
|
|
#ifndef xip_iprefetch
|
|
#define xip_iprefetch() do { } while (0)
|
|
#endif
|
|
|
|
/*
|
|
* xip_cpu_idle() is used when waiting for a delay equal or larger than
|
|
* the system timer tick period. This should put the CPU into idle mode
|
|
* to save power and to be woken up only when some interrupts are pending.
|
|
* This should not rely upon standard kernel code.
|
|
*/
|
|
#ifndef xip_cpu_idle
|
|
#define xip_cpu_idle() do { } while (0)
|
|
#endif
|
|
|
|
#endif /* CONFIG_MTD_XIP */
|
|
|
|
#ifndef __xipram
|
|
#define __xipram
|
|
#endif
|
|
|
|
#endif /* __LINUX_MTD_XIP_H__ */
|