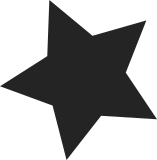
To make ICMPv6 closer to ICMPv4, add ratemask parameter. Since the ICMP message types use larger numeric values, a simple bitmask doesn't fit. I use large bitmap. The input and output are the in form of list of ranges. Set the default to rate limit all error messages but Packet Too Big. For Packet Too Big, use ratemask instead of hard-coded. There are functions where icmpv6_xrlim_allow() and icmpv6_global_allow() aren't called. This patch only adds them to icmpv6_echo_reply(). Rate limiting error messages is mandated by RFC 4443 but RFC 4890 says that it is also acceptable to rate limit informational messages. Thus, I removed the current hard-coded behavior of icmpv6_mask_allow() that doesn't rate limit informational messages. v2: Add dummy function proc_do_large_bitmap() if CONFIG_PROC_SYSCTL isn't defined, expand the description in ip-sysctl.txt and remove unnecessary conditional before kfree(). v3: Inline the bitmap instead of dynamically allocated. Still is a pointer to it is needed because of the way proc_do_large_bitmap work. Signed-off-by: Stephen Suryaputra <ssuryaextr@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
124 lines
3.1 KiB
C
124 lines
3.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* ipv6 in net namespaces
|
|
*/
|
|
|
|
#include <net/inet_frag.h>
|
|
|
|
#ifndef __NETNS_IPV6_H__
|
|
#define __NETNS_IPV6_H__
|
|
#include <net/dst_ops.h>
|
|
#include <uapi/linux/icmpv6.h>
|
|
|
|
struct ctl_table_header;
|
|
|
|
struct netns_sysctl_ipv6 {
|
|
#ifdef CONFIG_SYSCTL
|
|
struct ctl_table_header *hdr;
|
|
struct ctl_table_header *route_hdr;
|
|
struct ctl_table_header *icmp_hdr;
|
|
struct ctl_table_header *frags_hdr;
|
|
struct ctl_table_header *xfrm6_hdr;
|
|
#endif
|
|
int bindv6only;
|
|
int flush_delay;
|
|
int ip6_rt_max_size;
|
|
int ip6_rt_gc_min_interval;
|
|
int ip6_rt_gc_timeout;
|
|
int ip6_rt_gc_interval;
|
|
int ip6_rt_gc_elasticity;
|
|
int ip6_rt_mtu_expires;
|
|
int ip6_rt_min_advmss;
|
|
int multipath_hash_policy;
|
|
int flowlabel_consistency;
|
|
int auto_flowlabels;
|
|
int icmpv6_time;
|
|
int icmpv6_echo_ignore_all;
|
|
int icmpv6_echo_ignore_multicast;
|
|
int icmpv6_echo_ignore_anycast;
|
|
DECLARE_BITMAP(icmpv6_ratemask, ICMPV6_MSG_MAX + 1);
|
|
unsigned long *icmpv6_ratemask_ptr;
|
|
int anycast_src_echo_reply;
|
|
int ip_nonlocal_bind;
|
|
int fwmark_reflect;
|
|
int idgen_retries;
|
|
int idgen_delay;
|
|
int flowlabel_state_ranges;
|
|
int flowlabel_reflect;
|
|
int max_dst_opts_cnt;
|
|
int max_hbh_opts_cnt;
|
|
int max_dst_opts_len;
|
|
int max_hbh_opts_len;
|
|
int seg6_flowlabel;
|
|
bool skip_notify_on_dev_down;
|
|
};
|
|
|
|
struct netns_ipv6 {
|
|
struct netns_sysctl_ipv6 sysctl;
|
|
struct ipv6_devconf *devconf_all;
|
|
struct ipv6_devconf *devconf_dflt;
|
|
struct inet_peer_base *peers;
|
|
struct netns_frags frags;
|
|
#ifdef CONFIG_NETFILTER
|
|
struct xt_table *ip6table_filter;
|
|
struct xt_table *ip6table_mangle;
|
|
struct xt_table *ip6table_raw;
|
|
#ifdef CONFIG_SECURITY
|
|
struct xt_table *ip6table_security;
|
|
#endif
|
|
struct xt_table *ip6table_nat;
|
|
#endif
|
|
struct fib6_info *fib6_null_entry;
|
|
struct rt6_info *ip6_null_entry;
|
|
struct rt6_statistics *rt6_stats;
|
|
struct timer_list ip6_fib_timer;
|
|
struct hlist_head *fib_table_hash;
|
|
struct fib6_table *fib6_main_tbl;
|
|
struct list_head fib6_walkers;
|
|
struct dst_ops ip6_dst_ops;
|
|
rwlock_t fib6_walker_lock;
|
|
spinlock_t fib6_gc_lock;
|
|
unsigned int ip6_rt_gc_expire;
|
|
unsigned long ip6_rt_last_gc;
|
|
#ifdef CONFIG_IPV6_MULTIPLE_TABLES
|
|
unsigned int fib6_rules_require_fldissect;
|
|
bool fib6_has_custom_rules;
|
|
struct rt6_info *ip6_prohibit_entry;
|
|
struct rt6_info *ip6_blk_hole_entry;
|
|
struct fib6_table *fib6_local_tbl;
|
|
struct fib_rules_ops *fib6_rules_ops;
|
|
#endif
|
|
struct sock * __percpu *icmp_sk;
|
|
struct sock *ndisc_sk;
|
|
struct sock *tcp_sk;
|
|
struct sock *igmp_sk;
|
|
struct sock *mc_autojoin_sk;
|
|
#ifdef CONFIG_IPV6_MROUTE
|
|
#ifndef CONFIG_IPV6_MROUTE_MULTIPLE_TABLES
|
|
struct mr_table *mrt6;
|
|
#else
|
|
struct list_head mr6_tables;
|
|
struct fib_rules_ops *mr6_rules_ops;
|
|
#endif
|
|
#endif
|
|
atomic_t dev_addr_genid;
|
|
atomic_t fib6_sernum;
|
|
struct seg6_pernet_data *seg6_data;
|
|
struct fib_notifier_ops *notifier_ops;
|
|
struct fib_notifier_ops *ip6mr_notifier_ops;
|
|
unsigned int ipmr_seq; /* protected by rtnl_mutex */
|
|
struct {
|
|
struct hlist_head head;
|
|
spinlock_t lock;
|
|
u32 seq;
|
|
} ip6addrlbl_table;
|
|
};
|
|
|
|
#if IS_ENABLED(CONFIG_NF_DEFRAG_IPV6)
|
|
struct netns_nf_frag {
|
|
struct netns_frags frags;
|
|
};
|
|
#endif
|
|
|
|
#endif
|