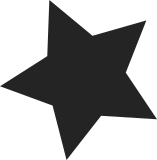
1/ Add support for the ACPI 6.0 NFIT hot add mechanism to process updates of the NFIT at runtime. 2/ Teach the coredump implementation how to filter out DAX mappings. 3/ Introduce NUMA hints for allocations made by the pmem driver, and as a side effect all devm allocations now hint their NUMA node by default. -----BEGIN PGP SIGNATURE----- Version: GnuPG v1 iQIcBAABAgAGBQJWQX2sAAoJEB7SkWpmfYgCWsEQAK7w/xM9zClVY/DDlFJxFtYq DZJ4faPj+E3FMTiJIEDzjtRgQvOFE+wmJtntYsCqKH/QZmpnyk9jeT/CbJzEEL2k WsAk+qHGLcVUlSb36blwN1RFzYqC+IDYThewJqUvxDbOwL1AbiibbX7gplzZHLhW +rj3ScVlSNOPRDgGGpkAeLNNsttuKvsGo7nB/JZopm0tV6g14rSK09wQbVhv6S6T Lu7VGYqnJlkteL9YlzRiROf9hW2ZFCMGJz1YZydPTy3aX3hGTBX4w2qvmsPwBIKP kW/gCNisVJGk1cZCk4joSJ8i/b3x3fE0zdZ5waivJ5jDvYbUUfyk0KtJkfw207Rl 14yWitUC6aeVuCeOqXHgsjRi+1QVN9Pg7i49xgGiUN1igQiUYRTgQPWZxDv6Zo/s USrLFQBaRd+hJw+dl7A47lJ3mUF96tPCoQb4LCQ7DVsg5U4J2TvqXLH9Gek/CCZ4 QsMkZDTQlZw4+JEDlzBgg/L7xVty8DadplTADMdjaRhFU3y8zKNJ85Ileokt7KVt IsBT4+S5HeZLvinZY95932DwAmFp1DtsyENd1BUXL06ddyvlQrFJ6NQaXji4fuDc EVQmMoTAqDujZFupMAux9vkUBDFj/hmaVD5F7j3+MWP87OCritw/IZn+2LgTaKoX EmttaYrDr2jJwIaGyw+H =a2/L -----END PGP SIGNATURE----- Merge tag 'libnvdimm-for-4.4' of git://git.kernel.org/pub/scm/linux/kernel/git/nvdimm/nvdimm Pull libnvdimm updates from Dan Williams: "Outside of the new ACPI-NFIT hot-add support this pull request is more notable for what it does not contain, than what it does. There were a handful of development topics this cycle, dax get_user_pages, dax fsync, and raw block dax, that need more more iteration and will wait for 4.5. The patches to make devm and the pmem driver NUMA aware have been in -next for several weeks. The hot-add support has not, but is contained to the NFIT driver and is passing unit tests. The coredump support is straightforward and was looked over by Jeff. All of it has received a 0day build success notification across 107 configs. Summary: - Add support for the ACPI 6.0 NFIT hot add mechanism to process updates of the NFIT at runtime. - Teach the coredump implementation how to filter out DAX mappings. - Introduce NUMA hints for allocations made by the pmem driver, and as a side effect all devm allocations now hint their NUMA node by default" * tag 'libnvdimm-for-4.4' of git://git.kernel.org/pub/scm/linux/kernel/git/nvdimm/nvdimm: coredump: add DAX filtering for FDPIC ELF coredumps coredump: add DAX filtering for ELF coredumps acpi: nfit: Add support for hot-add nfit: in acpi_nfit_init, break on a 0-length table pmem, memremap: convert to numa aware allocations devm_memremap_pages: use numa_mem_id devm: make allocations numa aware by default devm_memremap: convert to return ERR_PTR devm_memunmap: use devres_release() pmem: kill memremap_pmem() x86, mm: quiet arch_add_memory()
201 lines
5.4 KiB
C
201 lines
5.4 KiB
C
/*
|
|
* Copyright(c) 2015 Intel Corporation. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of version 2 of the GNU General Public License as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*/
|
|
#include <linux/device.h>
|
|
#include <linux/types.h>
|
|
#include <linux/io.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/memory_hotplug.h>
|
|
|
|
#ifndef ioremap_cache
|
|
/* temporary while we convert existing ioremap_cache users to memremap */
|
|
__weak void __iomem *ioremap_cache(resource_size_t offset, unsigned long size)
|
|
{
|
|
return ioremap(offset, size);
|
|
}
|
|
#endif
|
|
|
|
static void *try_ram_remap(resource_size_t offset, size_t size)
|
|
{
|
|
struct page *page = pfn_to_page(offset >> PAGE_SHIFT);
|
|
|
|
/* In the simple case just return the existing linear address */
|
|
if (!PageHighMem(page))
|
|
return __va(offset);
|
|
return NULL; /* fallback to ioremap_cache */
|
|
}
|
|
|
|
/**
|
|
* memremap() - remap an iomem_resource as cacheable memory
|
|
* @offset: iomem resource start address
|
|
* @size: size of remap
|
|
* @flags: either MEMREMAP_WB or MEMREMAP_WT
|
|
*
|
|
* memremap() is "ioremap" for cases where it is known that the resource
|
|
* being mapped does not have i/o side effects and the __iomem
|
|
* annotation is not applicable.
|
|
*
|
|
* MEMREMAP_WB - matches the default mapping for "System RAM" on
|
|
* the architecture. This is usually a read-allocate write-back cache.
|
|
* Morever, if MEMREMAP_WB is specified and the requested remap region is RAM
|
|
* memremap() will bypass establishing a new mapping and instead return
|
|
* a pointer into the direct map.
|
|
*
|
|
* MEMREMAP_WT - establish a mapping whereby writes either bypass the
|
|
* cache or are written through to memory and never exist in a
|
|
* cache-dirty state with respect to program visibility. Attempts to
|
|
* map "System RAM" with this mapping type will fail.
|
|
*/
|
|
void *memremap(resource_size_t offset, size_t size, unsigned long flags)
|
|
{
|
|
int is_ram = region_intersects(offset, size, "System RAM");
|
|
void *addr = NULL;
|
|
|
|
if (is_ram == REGION_MIXED) {
|
|
WARN_ONCE(1, "memremap attempted on mixed range %pa size: %#lx\n",
|
|
&offset, (unsigned long) size);
|
|
return NULL;
|
|
}
|
|
|
|
/* Try all mapping types requested until one returns non-NULL */
|
|
if (flags & MEMREMAP_WB) {
|
|
flags &= ~MEMREMAP_WB;
|
|
/*
|
|
* MEMREMAP_WB is special in that it can be satisifed
|
|
* from the direct map. Some archs depend on the
|
|
* capability of memremap() to autodetect cases where
|
|
* the requested range is potentially in "System RAM"
|
|
*/
|
|
if (is_ram == REGION_INTERSECTS)
|
|
addr = try_ram_remap(offset, size);
|
|
if (!addr)
|
|
addr = ioremap_cache(offset, size);
|
|
}
|
|
|
|
/*
|
|
* If we don't have a mapping yet and more request flags are
|
|
* pending then we will be attempting to establish a new virtual
|
|
* address mapping. Enforce that this mapping is not aliasing
|
|
* "System RAM"
|
|
*/
|
|
if (!addr && is_ram == REGION_INTERSECTS && flags) {
|
|
WARN_ONCE(1, "memremap attempted on ram %pa size: %#lx\n",
|
|
&offset, (unsigned long) size);
|
|
return NULL;
|
|
}
|
|
|
|
if (!addr && (flags & MEMREMAP_WT)) {
|
|
flags &= ~MEMREMAP_WT;
|
|
addr = ioremap_wt(offset, size);
|
|
}
|
|
|
|
return addr;
|
|
}
|
|
EXPORT_SYMBOL(memremap);
|
|
|
|
void memunmap(void *addr)
|
|
{
|
|
if (is_vmalloc_addr(addr))
|
|
iounmap((void __iomem *) addr);
|
|
}
|
|
EXPORT_SYMBOL(memunmap);
|
|
|
|
static void devm_memremap_release(struct device *dev, void *res)
|
|
{
|
|
memunmap(res);
|
|
}
|
|
|
|
static int devm_memremap_match(struct device *dev, void *res, void *match_data)
|
|
{
|
|
return *(void **)res == match_data;
|
|
}
|
|
|
|
void *devm_memremap(struct device *dev, resource_size_t offset,
|
|
size_t size, unsigned long flags)
|
|
{
|
|
void **ptr, *addr;
|
|
|
|
ptr = devres_alloc_node(devm_memremap_release, sizeof(*ptr), GFP_KERNEL,
|
|
dev_to_node(dev));
|
|
if (!ptr)
|
|
return ERR_PTR(-ENOMEM);
|
|
|
|
addr = memremap(offset, size, flags);
|
|
if (addr) {
|
|
*ptr = addr;
|
|
devres_add(dev, ptr);
|
|
} else
|
|
devres_free(ptr);
|
|
|
|
return addr;
|
|
}
|
|
EXPORT_SYMBOL(devm_memremap);
|
|
|
|
void devm_memunmap(struct device *dev, void *addr)
|
|
{
|
|
WARN_ON(devres_release(dev, devm_memremap_release,
|
|
devm_memremap_match, addr));
|
|
}
|
|
EXPORT_SYMBOL(devm_memunmap);
|
|
|
|
#ifdef CONFIG_ZONE_DEVICE
|
|
struct page_map {
|
|
struct resource res;
|
|
};
|
|
|
|
static void devm_memremap_pages_release(struct device *dev, void *res)
|
|
{
|
|
struct page_map *page_map = res;
|
|
|
|
/* pages are dead and unused, undo the arch mapping */
|
|
arch_remove_memory(page_map->res.start, resource_size(&page_map->res));
|
|
}
|
|
|
|
void *devm_memremap_pages(struct device *dev, struct resource *res)
|
|
{
|
|
int is_ram = region_intersects(res->start, resource_size(res),
|
|
"System RAM");
|
|
struct page_map *page_map;
|
|
int error, nid;
|
|
|
|
if (is_ram == REGION_MIXED) {
|
|
WARN_ONCE(1, "%s attempted on mixed region %pr\n",
|
|
__func__, res);
|
|
return ERR_PTR(-ENXIO);
|
|
}
|
|
|
|
if (is_ram == REGION_INTERSECTS)
|
|
return __va(res->start);
|
|
|
|
page_map = devres_alloc_node(devm_memremap_pages_release,
|
|
sizeof(*page_map), GFP_KERNEL, dev_to_node(dev));
|
|
if (!page_map)
|
|
return ERR_PTR(-ENOMEM);
|
|
|
|
memcpy(&page_map->res, res, sizeof(*res));
|
|
|
|
nid = dev_to_node(dev);
|
|
if (nid < 0)
|
|
nid = numa_mem_id();
|
|
|
|
error = arch_add_memory(nid, res->start, resource_size(res), true);
|
|
if (error) {
|
|
devres_free(page_map);
|
|
return ERR_PTR(error);
|
|
}
|
|
|
|
devres_add(dev, page_map);
|
|
return __va(res->start);
|
|
}
|
|
EXPORT_SYMBOL(devm_memremap_pages);
|
|
#endif /* CONFIG_ZONE_DEVICE */
|