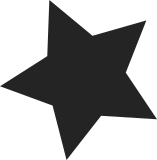
When EEH error happens to one specific PE, the device drivers of its attached EEH devices (PCI devices) are checked to see the further action: reset with complete hotplug, or reset without hotplug. However, that's not enough for those PCI devices whose drivers can't support EEH, or those PCI devices without driver. So we need do so-called "partial hotplug" on basis of PCI devices. In the situation, part of PCI devices of the specific PE are unplugged and plugged again after PE reset. The patch changes pcibios_add_pci_devices() so that it can support full hotplug and so-called "partial" hotplug based on device-tree or real hardware. It's notable that pci_of_scan.c has been changed for a bit in order to support the "partial" hotplug based on dev-tree. Most of the generic code already supports that, we just need to plumb it properly on our side. Signed-off-by: Gavin Shan <shangw@linux.vnet.ibm.com> Signed-off-by: Benjamin Herrenschmidt <benh@kernel.crashing.org>
111 lines
3.3 KiB
C
111 lines
3.3 KiB
C
/*
|
|
* Derived from "arch/powerpc/platforms/pseries/pci_dlpar.c"
|
|
*
|
|
* Copyright (C) 2003 Linda Xie <lxie@us.ibm.com>
|
|
* Copyright (C) 2005 International Business Machines
|
|
*
|
|
* Updates, 2005, John Rose <johnrose@austin.ibm.com>
|
|
* Updates, 2005, Linas Vepstas <linas@austin.ibm.com>
|
|
* Updates, 2013, Gavin Shan <shangw@linux.vnet.ibm.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/pci.h>
|
|
#include <linux/export.h>
|
|
#include <asm/pci-bridge.h>
|
|
#include <asm/ppc-pci.h>
|
|
#include <asm/firmware.h>
|
|
#include <asm/eeh.h>
|
|
|
|
/**
|
|
* pcibios_release_device - release PCI device
|
|
* @dev: PCI device
|
|
*
|
|
* The function is called before releasing the indicated PCI device.
|
|
*/
|
|
void pcibios_release_device(struct pci_dev *dev)
|
|
{
|
|
eeh_remove_device(dev);
|
|
}
|
|
|
|
/**
|
|
* pcibios_remove_pci_devices - remove all devices under this bus
|
|
* @bus: the indicated PCI bus
|
|
*
|
|
* Remove all of the PCI devices under this bus both from the
|
|
* linux pci device tree, and from the powerpc EEH address cache.
|
|
*/
|
|
void pcibios_remove_pci_devices(struct pci_bus *bus)
|
|
{
|
|
struct pci_dev *dev, *tmp;
|
|
struct pci_bus *child_bus;
|
|
|
|
/* First go down child busses */
|
|
list_for_each_entry(child_bus, &bus->children, node)
|
|
pcibios_remove_pci_devices(child_bus);
|
|
|
|
pr_debug("PCI: Removing devices on bus %04x:%02x\n",
|
|
pci_domain_nr(bus), bus->number);
|
|
list_for_each_entry_safe(dev, tmp, &bus->devices, bus_list) {
|
|
pr_debug(" Removing %s...\n", pci_name(dev));
|
|
pci_stop_and_remove_bus_device(dev);
|
|
}
|
|
}
|
|
|
|
EXPORT_SYMBOL_GPL(pcibios_remove_pci_devices);
|
|
|
|
/**
|
|
* pcibios_add_pci_devices - adds new pci devices to bus
|
|
* @bus: the indicated PCI bus
|
|
*
|
|
* This routine will find and fixup new pci devices under
|
|
* the indicated bus. This routine presumes that there
|
|
* might already be some devices under this bridge, so
|
|
* it carefully tries to add only new devices. (And that
|
|
* is how this routine differs from other, similar pcibios
|
|
* routines.)
|
|
*/
|
|
void pcibios_add_pci_devices(struct pci_bus * bus)
|
|
{
|
|
int slotno, mode, pass, max;
|
|
struct pci_dev *dev;
|
|
struct device_node *dn = pci_bus_to_OF_node(bus);
|
|
|
|
eeh_add_device_tree_early(dn);
|
|
|
|
mode = PCI_PROBE_NORMAL;
|
|
if (ppc_md.pci_probe_mode)
|
|
mode = ppc_md.pci_probe_mode(bus);
|
|
|
|
if (mode == PCI_PROBE_DEVTREE) {
|
|
/* use ofdt-based probe */
|
|
of_rescan_bus(dn, bus);
|
|
} else if (mode == PCI_PROBE_NORMAL) {
|
|
/*
|
|
* Use legacy probe. In the partial hotplug case, we
|
|
* probably have grandchildren devices unplugged. So
|
|
* we don't check the return value from pci_scan_slot() in
|
|
* order for fully rescan all the way down to pick them up.
|
|
* They can have been removed during partial hotplug.
|
|
*/
|
|
slotno = PCI_SLOT(PCI_DN(dn->child)->devfn);
|
|
pci_scan_slot(bus, PCI_DEVFN(slotno, 0));
|
|
pcibios_setup_bus_devices(bus);
|
|
max = bus->busn_res.start;
|
|
for (pass = 0; pass < 2; pass++) {
|
|
list_for_each_entry(dev, &bus->devices, bus_list) {
|
|
if (dev->hdr_type == PCI_HEADER_TYPE_BRIDGE ||
|
|
dev->hdr_type == PCI_HEADER_TYPE_CARDBUS)
|
|
max = pci_scan_bridge(bus, dev,
|
|
max, pass);
|
|
}
|
|
}
|
|
}
|
|
pcibios_finish_adding_to_bus(bus);
|
|
}
|
|
EXPORT_SYMBOL_GPL(pcibios_add_pci_devices);
|