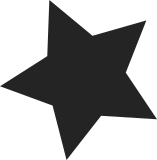
Based on 1 normalized pattern(s): this program is free software you can redistribute it and or modify it under the terms of the gnu general public license as published by the free software foundation either version 2 of the license or at your option any later version this program is distributed in the hope that it will be useful but without any warranty without even the implied warranty of merchantability or fitness for a particular purpose see the gnu general public license for more details you should have received a copy of the gnu general public license along with this program if not write to the free software foundation inc 59 temple place suite 330 boston ma 02111 1307 usa extracted by the scancode license scanner the SPDX license identifier GPL-2.0-or-later has been chosen to replace the boilerplate/reference in 1334 file(s). Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Reviewed-by: Allison Randal <allison@lohutok.net> Reviewed-by: Richard Fontana <rfontana@redhat.com> Cc: linux-spdx@vger.kernel.org Link: https://lkml.kernel.org/r/20190527070033.113240726@linutronix.de Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
148 lines
3.4 KiB
C
148 lines
3.4 KiB
C
// SPDX-License-Identifier: GPL-2.0-or-later
|
|
/*
|
|
* Misc and compatibility things
|
|
* Copyright (c) by Jaroslav Kysela <perex@perex.cz>
|
|
*/
|
|
|
|
#include <linux/init.h>
|
|
#include <linux/export.h>
|
|
#include <linux/moduleparam.h>
|
|
#include <linux/time.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/ioport.h>
|
|
#include <sound/core.h>
|
|
|
|
#ifdef CONFIG_SND_DEBUG
|
|
|
|
#ifdef CONFIG_SND_DEBUG_VERBOSE
|
|
#define DEFAULT_DEBUG_LEVEL 2
|
|
#else
|
|
#define DEFAULT_DEBUG_LEVEL 1
|
|
#endif
|
|
|
|
static int debug = DEFAULT_DEBUG_LEVEL;
|
|
module_param(debug, int, 0644);
|
|
MODULE_PARM_DESC(debug, "Debug level (0 = disable)");
|
|
|
|
#endif /* CONFIG_SND_DEBUG */
|
|
|
|
void release_and_free_resource(struct resource *res)
|
|
{
|
|
if (res) {
|
|
release_resource(res);
|
|
kfree(res);
|
|
}
|
|
}
|
|
EXPORT_SYMBOL(release_and_free_resource);
|
|
|
|
#ifdef CONFIG_SND_VERBOSE_PRINTK
|
|
/* strip the leading path if the given path is absolute */
|
|
static const char *sanity_file_name(const char *path)
|
|
{
|
|
if (*path == '/')
|
|
return strrchr(path, '/') + 1;
|
|
else
|
|
return path;
|
|
}
|
|
#endif
|
|
|
|
#if defined(CONFIG_SND_DEBUG) || defined(CONFIG_SND_VERBOSE_PRINTK)
|
|
void __snd_printk(unsigned int level, const char *path, int line,
|
|
const char *format, ...)
|
|
{
|
|
va_list args;
|
|
#ifdef CONFIG_SND_VERBOSE_PRINTK
|
|
int kern_level;
|
|
struct va_format vaf;
|
|
char verbose_fmt[] = KERN_DEFAULT "ALSA %s:%d %pV";
|
|
bool level_found = false;
|
|
#endif
|
|
|
|
#ifdef CONFIG_SND_DEBUG
|
|
if (debug < level)
|
|
return;
|
|
#endif
|
|
|
|
va_start(args, format);
|
|
#ifdef CONFIG_SND_VERBOSE_PRINTK
|
|
vaf.fmt = format;
|
|
vaf.va = &args;
|
|
|
|
while ((kern_level = printk_get_level(vaf.fmt)) != 0) {
|
|
const char *end_of_header = printk_skip_level(vaf.fmt);
|
|
|
|
/* Ignore KERN_CONT. We print filename:line for each piece. */
|
|
if (kern_level >= '0' && kern_level <= '7') {
|
|
memcpy(verbose_fmt, vaf.fmt, end_of_header - vaf.fmt);
|
|
level_found = true;
|
|
}
|
|
|
|
vaf.fmt = end_of_header;
|
|
}
|
|
|
|
if (!level_found && level)
|
|
memcpy(verbose_fmt, KERN_DEBUG, sizeof(KERN_DEBUG) - 1);
|
|
|
|
printk(verbose_fmt, sanity_file_name(path), line, &vaf);
|
|
#else
|
|
vprintk(format, args);
|
|
#endif
|
|
va_end(args);
|
|
}
|
|
EXPORT_SYMBOL_GPL(__snd_printk);
|
|
#endif
|
|
|
|
#ifdef CONFIG_PCI
|
|
#include <linux/pci.h>
|
|
/**
|
|
* snd_pci_quirk_lookup_id - look up a PCI SSID quirk list
|
|
* @vendor: PCI SSV id
|
|
* @device: PCI SSD id
|
|
* @list: quirk list, terminated by a null entry
|
|
*
|
|
* Look through the given quirk list and finds a matching entry
|
|
* with the same PCI SSID. When subdevice is 0, all subdevice
|
|
* values may match.
|
|
*
|
|
* Returns the matched entry pointer, or NULL if nothing matched.
|
|
*/
|
|
const struct snd_pci_quirk *
|
|
snd_pci_quirk_lookup_id(u16 vendor, u16 device,
|
|
const struct snd_pci_quirk *list)
|
|
{
|
|
const struct snd_pci_quirk *q;
|
|
|
|
for (q = list; q->subvendor; q++) {
|
|
if (q->subvendor != vendor)
|
|
continue;
|
|
if (!q->subdevice ||
|
|
(device & q->subdevice_mask) == q->subdevice)
|
|
return q;
|
|
}
|
|
return NULL;
|
|
}
|
|
EXPORT_SYMBOL(snd_pci_quirk_lookup_id);
|
|
|
|
/**
|
|
* snd_pci_quirk_lookup - look up a PCI SSID quirk list
|
|
* @pci: pci_dev handle
|
|
* @list: quirk list, terminated by a null entry
|
|
*
|
|
* Look through the given quirk list and finds a matching entry
|
|
* with the same PCI SSID. When subdevice is 0, all subdevice
|
|
* values may match.
|
|
*
|
|
* Returns the matched entry pointer, or NULL if nothing matched.
|
|
*/
|
|
const struct snd_pci_quirk *
|
|
snd_pci_quirk_lookup(struct pci_dev *pci, const struct snd_pci_quirk *list)
|
|
{
|
|
if (!pci)
|
|
return NULL;
|
|
return snd_pci_quirk_lookup_id(pci->subsystem_vendor,
|
|
pci->subsystem_device,
|
|
list);
|
|
}
|
|
EXPORT_SYMBOL(snd_pci_quirk_lookup);
|
|
#endif
|