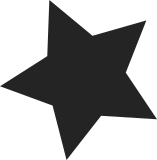
loop block device handles IO in a separate thread. The actual IO dispatched isn't cloned from the IO loop device received, so the dispatched IO loses the cgroup context. I'm ignoring buffer IO case now, which is quite complicated. Making the loop thread aware cgroup context doesn't really help. The loop device only writes to a single file. In current writeback cgroup implementation, the file can only belong to one cgroup. For direct IO case, we could workaround the issue in theory. For example, say we assign cgroup1 5M/s BW for loop device and cgroup2 10M/s. We can create a special cgroup for loop thread and assign at least 15M/s for the underlayer disk. In this way, we correctly throttle the two cgroups. But this is tricky to setup. This patch tries to address the issue. We record bio's css in loop command. When loop thread is handling the command, we then use the API provided in patch 1 to set the css for current task. The bio layer will use the css for new IO (from patch 3). Acked-by: Tejun Heo <tj@kernel.org> Signed-off-by: Shaohua Li <shli@fb.com> Signed-off-by: Jens Axboe <axboe@kernel.dk>
96 lines
2.3 KiB
C
96 lines
2.3 KiB
C
/*
|
|
* loop.h
|
|
*
|
|
* Written by Theodore Ts'o, 3/29/93.
|
|
*
|
|
* Copyright 1993 by Theodore Ts'o. Redistribution of this file is
|
|
* permitted under the GNU General Public License.
|
|
*/
|
|
#ifndef _LINUX_LOOP_H
|
|
#define _LINUX_LOOP_H
|
|
|
|
#include <linux/bio.h>
|
|
#include <linux/blkdev.h>
|
|
#include <linux/blk-mq.h>
|
|
#include <linux/spinlock.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/kthread.h>
|
|
#include <uapi/linux/loop.h>
|
|
|
|
/* Possible states of device */
|
|
enum {
|
|
Lo_unbound,
|
|
Lo_bound,
|
|
Lo_rundown,
|
|
};
|
|
|
|
struct loop_func_table;
|
|
|
|
struct loop_device {
|
|
int lo_number;
|
|
atomic_t lo_refcnt;
|
|
loff_t lo_offset;
|
|
loff_t lo_sizelimit;
|
|
int lo_flags;
|
|
int (*transfer)(struct loop_device *, int cmd,
|
|
struct page *raw_page, unsigned raw_off,
|
|
struct page *loop_page, unsigned loop_off,
|
|
int size, sector_t real_block);
|
|
char lo_file_name[LO_NAME_SIZE];
|
|
char lo_crypt_name[LO_NAME_SIZE];
|
|
char lo_encrypt_key[LO_KEY_SIZE];
|
|
int lo_encrypt_key_size;
|
|
struct loop_func_table *lo_encryption;
|
|
__u32 lo_init[2];
|
|
kuid_t lo_key_owner; /* Who set the key */
|
|
int (*ioctl)(struct loop_device *, int cmd,
|
|
unsigned long arg);
|
|
|
|
struct file * lo_backing_file;
|
|
struct block_device *lo_device;
|
|
void *key_data;
|
|
|
|
gfp_t old_gfp_mask;
|
|
|
|
spinlock_t lo_lock;
|
|
int lo_state;
|
|
struct mutex lo_ctl_mutex;
|
|
struct kthread_worker worker;
|
|
struct task_struct *worker_task;
|
|
bool use_dio;
|
|
|
|
struct request_queue *lo_queue;
|
|
struct blk_mq_tag_set tag_set;
|
|
struct gendisk *lo_disk;
|
|
};
|
|
|
|
struct loop_cmd {
|
|
struct kthread_work work;
|
|
struct request *rq;
|
|
bool use_aio; /* use AIO interface to handle I/O */
|
|
atomic_t ref; /* only for aio */
|
|
long ret;
|
|
struct kiocb iocb;
|
|
struct bio_vec *bvec;
|
|
struct cgroup_subsys_state *css;
|
|
};
|
|
|
|
/* Support for loadable transfer modules */
|
|
struct loop_func_table {
|
|
int number; /* filter type */
|
|
int (*transfer)(struct loop_device *lo, int cmd,
|
|
struct page *raw_page, unsigned raw_off,
|
|
struct page *loop_page, unsigned loop_off,
|
|
int size, sector_t real_block);
|
|
int (*init)(struct loop_device *, const struct loop_info64 *);
|
|
/* release is called from loop_unregister_transfer or clr_fd */
|
|
int (*release)(struct loop_device *);
|
|
int (*ioctl)(struct loop_device *, int cmd, unsigned long arg);
|
|
struct module *owner;
|
|
};
|
|
|
|
int loop_register_transfer(struct loop_func_table *funcs);
|
|
int loop_unregister_transfer(int number);
|
|
|
|
#endif
|