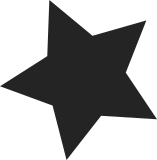
Reading /proc/net/snmp on a machine with a lot of cpus is very expensive (can be ~88000 us). This is because ICMPMSG MIB uses 4096 bytes per cpu, and folding values for all possible cpus can read 16 Mbytes of memory. ICMP messages are not considered as fast path on a typical server, and eventually few cpus handle them anyway. We can afford an atomic operation instead of using percpu data. This saves 4096 bytes per cpu and per network namespace. Signed-off-by: Eric Dumazet <eric.dumazet@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
49 lines
1.6 KiB
C
49 lines
1.6 KiB
C
/*
|
|
* INET An implementation of the TCP/IP protocol suite for the LINUX
|
|
* operating system. INET is implemented using the BSD Socket
|
|
* interface as the means of communication with the user level.
|
|
*
|
|
* Definitions for the ICMP module.
|
|
*
|
|
* Version: @(#)icmp.h 1.0.4 05/13/93
|
|
*
|
|
* Authors: Ross Biro
|
|
* Fred N. van Kempen, <waltje@uWalt.NL.Mugnet.ORG>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
#ifndef _ICMP_H
|
|
#define _ICMP_H
|
|
|
|
#include <linux/icmp.h>
|
|
|
|
#include <net/inet_sock.h>
|
|
#include <net/snmp.h>
|
|
|
|
struct icmp_err {
|
|
int errno;
|
|
unsigned fatal:1;
|
|
};
|
|
|
|
extern const struct icmp_err icmp_err_convert[];
|
|
#define ICMP_INC_STATS(net, field) SNMP_INC_STATS((net)->mib.icmp_statistics, field)
|
|
#define ICMP_INC_STATS_BH(net, field) SNMP_INC_STATS_BH((net)->mib.icmp_statistics, field)
|
|
#define ICMPMSGOUT_INC_STATS(net, field) SNMP_INC_STATS_ATOMIC_LONG((net)->mib.icmpmsg_statistics, field+256)
|
|
#define ICMPMSGIN_INC_STATS_BH(net, field) SNMP_INC_STATS_ATOMIC_LONG((net)->mib.icmpmsg_statistics, field)
|
|
|
|
struct dst_entry;
|
|
struct net_proto_family;
|
|
struct sk_buff;
|
|
struct net;
|
|
|
|
extern void icmp_send(struct sk_buff *skb_in, int type, int code, __be32 info);
|
|
extern int icmp_rcv(struct sk_buff *skb);
|
|
extern int icmp_ioctl(struct sock *sk, int cmd, unsigned long arg);
|
|
extern int icmp_init(void);
|
|
extern void icmp_out_count(struct net *net, unsigned char type);
|
|
|
|
#endif /* _ICMP_H */
|