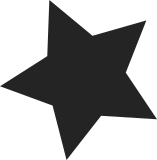
This adds two types of btree defrag, a run time form that tries to defrag recently allocated blocks in the btree when they are still in ram, and an ioctl that forces defrag of all btree blocks. File data blocks are not defragged yet, but this can make a huge difference in sequential btree reads. Signed-off-by: Chris Mason <chris.mason@oracle.com>
76 lines
2.3 KiB
C
76 lines
2.3 KiB
C
/*
|
|
* Copyright (C) 2007 Oracle. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public
|
|
* License v2 as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public
|
|
* License along with this program; if not, write to the
|
|
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
* Boston, MA 021110-1307, USA.
|
|
*/
|
|
|
|
#ifndef __TRANSACTION__
|
|
#define __TRANSACTION__
|
|
#include "btrfs_inode.h"
|
|
|
|
struct btrfs_transaction {
|
|
u64 transid;
|
|
unsigned long num_writers;
|
|
int in_commit;
|
|
int use_count;
|
|
int commit_done;
|
|
struct list_head list;
|
|
struct radix_tree_root dirty_pages;
|
|
unsigned long start_time;
|
|
wait_queue_head_t writer_wait;
|
|
wait_queue_head_t commit_wait;
|
|
};
|
|
|
|
struct btrfs_trans_handle {
|
|
u64 transid;
|
|
unsigned long blocks_reserved;
|
|
unsigned long blocks_used;
|
|
struct btrfs_transaction *transaction;
|
|
struct btrfs_block_group_cache *block_group;
|
|
};
|
|
|
|
|
|
static inline void btrfs_set_trans_block_group(struct btrfs_trans_handle *trans,
|
|
struct inode *inode)
|
|
{
|
|
trans->block_group = BTRFS_I(inode)->block_group;
|
|
}
|
|
|
|
static inline void btrfs_update_inode_block_group(struct
|
|
btrfs_trans_handle *trans,
|
|
struct inode *inode)
|
|
{
|
|
BTRFS_I(inode)->block_group = trans->block_group;
|
|
}
|
|
|
|
int btrfs_end_transaction(struct btrfs_trans_handle *trans,
|
|
struct btrfs_root *root);
|
|
struct btrfs_trans_handle *btrfs_start_transaction(struct btrfs_root *root,
|
|
int num_blocks);
|
|
int btrfs_write_and_wait_transaction(struct btrfs_trans_handle *trans,
|
|
struct btrfs_root *root);
|
|
int btrfs_commit_tree_roots(struct btrfs_trans_handle *trans,
|
|
struct btrfs_root *root);
|
|
|
|
void btrfs_transaction_cleaner(struct work_struct *work);
|
|
void btrfs_transaction_flush_work(struct btrfs_root *root);
|
|
void btrfs_transaction_queue_work(struct btrfs_root *root, int delay);
|
|
void btrfs_init_transaction_sys(void);
|
|
void btrfs_exit_transaction_sys(void);
|
|
int btrfs_add_dead_root(struct btrfs_root *root, struct list_head *dead_list);
|
|
int btrfs_defrag_dirty_roots(struct btrfs_fs_info *info);
|
|
|
|
#endif
|