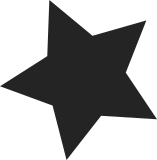
With the tegra3 and the big.LITTLE [1] new architectures, several cpus with different characteristics (latencies and states) can co-exists on the system. The cpuidle framework has the limitation of handling only identical cpus. This patch removes this limitation by introducing the multiple driver support for cpuidle. This option is configurable at compile time and should be enabled for the architectures mentioned above. So there is no impact for the other platforms if the option is disabled. The option defaults to 'n'. Note the multiple drivers support is also compatible with the existing drivers, even if just one driver is needed, all the cpu will be tied to this driver using an extra small chunk of processor memory. The multiple driver support use a per-cpu driver pointer instead of a global variable and the accessor to this variable are done from a cpu context. In order to keep the compatibility with the existing drivers, the function 'cpuidle_register_driver' and 'cpuidle_unregister_driver' will register the specified driver for all the cpus. The semantic for the output of /sys/devices/system/cpu/cpuidle/current_driver remains the same except the driver name will be related to the current cpu. The /sys/devices/system/cpu/cpu[0-9]/cpuidle/driver/name files are added allowing to read the per cpu driver name. [1] http://lwn.net/Articles/481055/ Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org> Acked-by: Peter De Schrijver <pdeschrijver@nvidia.com> Signed-off-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com>
67 lines
1.9 KiB
C
67 lines
1.9 KiB
C
/*
|
|
* cpuidle.h - The internal header file
|
|
*/
|
|
|
|
#ifndef __DRIVER_CPUIDLE_H
|
|
#define __DRIVER_CPUIDLE_H
|
|
|
|
/* For internal use only */
|
|
extern struct cpuidle_governor *cpuidle_curr_governor;
|
|
extern struct list_head cpuidle_governors;
|
|
extern struct list_head cpuidle_detected_devices;
|
|
extern struct mutex cpuidle_lock;
|
|
extern spinlock_t cpuidle_driver_lock;
|
|
extern int cpuidle_disabled(void);
|
|
extern int cpuidle_enter_state(struct cpuidle_device *dev,
|
|
struct cpuidle_driver *drv, int next_state);
|
|
|
|
/* idle loop */
|
|
extern void cpuidle_install_idle_handler(void);
|
|
extern void cpuidle_uninstall_idle_handler(void);
|
|
|
|
/* governors */
|
|
extern int cpuidle_switch_governor(struct cpuidle_governor *gov);
|
|
|
|
/* sysfs */
|
|
|
|
struct device;
|
|
|
|
extern int cpuidle_add_interface(struct device *dev);
|
|
extern void cpuidle_remove_interface(struct device *dev);
|
|
extern int cpuidle_add_device_sysfs(struct cpuidle_device *device);
|
|
extern void cpuidle_remove_device_sysfs(struct cpuidle_device *device);
|
|
extern int cpuidle_add_sysfs(struct cpuidle_device *dev);
|
|
extern void cpuidle_remove_sysfs(struct cpuidle_device *dev);
|
|
|
|
#ifdef CONFIG_ARCH_NEEDS_CPU_IDLE_COUPLED
|
|
bool cpuidle_state_is_coupled(struct cpuidle_device *dev,
|
|
struct cpuidle_driver *drv, int state);
|
|
int cpuidle_enter_state_coupled(struct cpuidle_device *dev,
|
|
struct cpuidle_driver *drv, int next_state);
|
|
int cpuidle_coupled_register_device(struct cpuidle_device *dev);
|
|
void cpuidle_coupled_unregister_device(struct cpuidle_device *dev);
|
|
#else
|
|
static inline bool cpuidle_state_is_coupled(struct cpuidle_device *dev,
|
|
struct cpuidle_driver *drv, int state)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
static inline int cpuidle_enter_state_coupled(struct cpuidle_device *dev,
|
|
struct cpuidle_driver *drv, int next_state)
|
|
{
|
|
return -1;
|
|
}
|
|
|
|
static inline int cpuidle_coupled_register_device(struct cpuidle_device *dev)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline void cpuidle_coupled_unregister_device(struct cpuidle_device *dev)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
#endif /* __DRIVER_CPUIDLE_H */
|