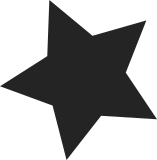
This patch refactors MIPS branch emulation code so as to allow skipping delay slot instruction in case of branch likely instructions when branch is not taken. This is useful for keeping the code common for use cases like kprobes where one would like to handle the branch instructions keeping the delay slot instuction also in picture for branch likely instructions. Also allow emulation when instruction to be decoded is not at pt_regs->cp0_epc as in case of kprobes where pt_regs->cp0_epc points to the breakpoint instruction. The patch also exports the function for modules. Signed-off-by: Maneesh Soni <manesoni@cisco.com> Signed-off-by: Victor Kamensky <kamensky@cisco.com> Cc: David Daney <david.daney@cavium.com> Cc: ananth@in.ibm.com Cc: linux-kernel@vger.kernel.org Cc: linux-mips@linux-mips.org Patchwork: https://patchwork.linux-mips.org/patch/2913/ Signed-off-by: Ralf Baechle <ralf@linux-mips.org>
44 lines
951 B
C
44 lines
951 B
C
/*
|
|
* This file is subject to the terms and conditions of the GNU General Public
|
|
* License. See the file "COPYING" in the main directory of this archive
|
|
* for more details.
|
|
*
|
|
* Copyright (C) 1996, 1997, 1998, 2001 by Ralf Baechle
|
|
*/
|
|
#ifndef _ASM_BRANCH_H
|
|
#define _ASM_BRANCH_H
|
|
|
|
#include <asm/ptrace.h>
|
|
#include <asm/inst.h>
|
|
|
|
static inline int delay_slot(struct pt_regs *regs)
|
|
{
|
|
return regs->cp0_cause & CAUSEF_BD;
|
|
}
|
|
|
|
static inline unsigned long exception_epc(struct pt_regs *regs)
|
|
{
|
|
if (!delay_slot(regs))
|
|
return regs->cp0_epc;
|
|
|
|
return regs->cp0_epc + 4;
|
|
}
|
|
|
|
#define BRANCH_LIKELY_TAKEN 0x0001
|
|
|
|
extern int __compute_return_epc(struct pt_regs *regs);
|
|
extern int __compute_return_epc_for_insn(struct pt_regs *regs,
|
|
union mips_instruction insn);
|
|
|
|
static inline int compute_return_epc(struct pt_regs *regs)
|
|
{
|
|
if (!delay_slot(regs)) {
|
|
regs->cp0_epc += 4;
|
|
return 0;
|
|
}
|
|
|
|
return __compute_return_epc(regs);
|
|
}
|
|
|
|
#endif /* _ASM_BRANCH_H */
|