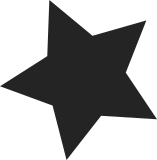
- Stolen ticks and PV wallclock support for arm/arm64. - Add grant copy ioctl to gntdev device. -----BEGIN PGP SIGNATURE----- Version: GnuPG v1 iQEcBAABAgAGBQJWk5IUAAoJEFxbo/MsZsTRLxwH/1BDcrbQDRc5hxUOG9JEYSUt H/lMjvZRShPkzweijdNon95ywAXhcSbkS9IV2Mp0+CZV7VyeymW7QIW/g4+G6iRg +LnoV77PAhPv/cmsr1pENXqRCclvemlxQOf7UyWLezuKhB71LC+oNaEnpk/tPIZS et/qef+m/SgSP5R91nO0Esv2KfP7za0UrgJf3Ee4GzjSeDkya0Hko06Cy3yc1/RT 082kHpQ1/KFcHHh2qhdCQwyzhq/cwFkuDA6ksKYJoxC6YAVC2mvvkuIOZYbloHDL c/dzuP9qjjxOZ7Gblv2cmg+RE4UqRfBhxmMycxSCcwW/Mt5LaftCpAxpBQKq2/8= =6F/q -----END PGP SIGNATURE----- Merge tag 'for-linus-4.5-rc0-tag' of git://git.kernel.org/pub/scm/linux/kernel/git/xen/tip Pull xen updates from David Vrabel: "Xen features and fixes for 4.5-rc0: - Stolen ticks and PV wallclock support for arm/arm64 - Add grant copy ioctl to gntdev device" * tag 'for-linus-4.5-rc0-tag' of git://git.kernel.org/pub/scm/linux/kernel/git/xen/tip: xen/gntdev: add ioctl for grant copy x86/xen: don't reset vcpu_info on a cancelled suspend xen/gntdev: constify mmu_notifier_ops structures xen/grant-table: constify gnttab_ops structure xen/time: use READ_ONCE xen/x86: convert remaining timespec to timespec64 in xen_pvclock_gtod_notify xen/x86: support XENPF_settime64 xen/arm: set the system time in Xen via the XENPF_settime64 hypercall xen/arm: introduce xen_read_wallclock arm: extend pvclock_wall_clock with sec_hi xen: introduce XENPF_settime64 xen/arm: introduce HYPERVISOR_platform_op on arm and arm64 xen: rename dom0_op to platform_op xen/arm: account for stolen ticks arm64: introduce CONFIG_PARAVIRT, PARAVIRT_TIME_ACCOUNTING and pv_time_ops arm: introduce CONFIG_PARAVIRT, PARAVIRT_TIME_ACCOUNTING and pv_time_ops missing include asm/paravirt.h in cputime.c xen: move xen_setup_runstate_info and get_runstate_snapshot to drivers/xen/time.c
118 lines
2.2 KiB
C
118 lines
2.2 KiB
C
#include <linux/types.h>
|
|
#include <linux/tick.h>
|
|
|
|
#include <xen/xen.h>
|
|
#include <xen/interface/xen.h>
|
|
#include <xen/grant_table.h>
|
|
#include <xen/events.h>
|
|
|
|
#include <asm/xen/hypercall.h>
|
|
#include <asm/xen/page.h>
|
|
#include <asm/fixmap.h>
|
|
|
|
#include "xen-ops.h"
|
|
#include "mmu.h"
|
|
#include "pmu.h"
|
|
|
|
static void xen_pv_pre_suspend(void)
|
|
{
|
|
xen_mm_pin_all();
|
|
|
|
xen_start_info->store_mfn = mfn_to_pfn(xen_start_info->store_mfn);
|
|
xen_start_info->console.domU.mfn =
|
|
mfn_to_pfn(xen_start_info->console.domU.mfn);
|
|
|
|
BUG_ON(!irqs_disabled());
|
|
|
|
HYPERVISOR_shared_info = &xen_dummy_shared_info;
|
|
if (HYPERVISOR_update_va_mapping(fix_to_virt(FIX_PARAVIRT_BOOTMAP),
|
|
__pte_ma(0), 0))
|
|
BUG();
|
|
}
|
|
|
|
static void xen_hvm_post_suspend(int suspend_cancelled)
|
|
{
|
|
#ifdef CONFIG_XEN_PVHVM
|
|
int cpu;
|
|
if (!suspend_cancelled)
|
|
xen_hvm_init_shared_info();
|
|
xen_callback_vector();
|
|
xen_unplug_emulated_devices();
|
|
if (xen_feature(XENFEAT_hvm_safe_pvclock)) {
|
|
for_each_online_cpu(cpu) {
|
|
xen_setup_runstate_info(cpu);
|
|
}
|
|
}
|
|
#endif
|
|
}
|
|
|
|
static void xen_pv_post_suspend(int suspend_cancelled)
|
|
{
|
|
xen_build_mfn_list_list();
|
|
|
|
xen_setup_shared_info();
|
|
|
|
if (suspend_cancelled) {
|
|
xen_start_info->store_mfn =
|
|
pfn_to_mfn(xen_start_info->store_mfn);
|
|
xen_start_info->console.domU.mfn =
|
|
pfn_to_mfn(xen_start_info->console.domU.mfn);
|
|
} else {
|
|
#ifdef CONFIG_SMP
|
|
BUG_ON(xen_cpu_initialized_map == NULL);
|
|
cpumask_copy(xen_cpu_initialized_map, cpu_online_mask);
|
|
#endif
|
|
xen_vcpu_restore();
|
|
}
|
|
|
|
xen_mm_unpin_all();
|
|
}
|
|
|
|
void xen_arch_pre_suspend(void)
|
|
{
|
|
if (xen_pv_domain())
|
|
xen_pv_pre_suspend();
|
|
}
|
|
|
|
void xen_arch_post_suspend(int cancelled)
|
|
{
|
|
if (xen_pv_domain())
|
|
xen_pv_post_suspend(cancelled);
|
|
else
|
|
xen_hvm_post_suspend(cancelled);
|
|
}
|
|
|
|
static void xen_vcpu_notify_restore(void *data)
|
|
{
|
|
/* Boot processor notified via generic timekeeping_resume() */
|
|
if (smp_processor_id() == 0)
|
|
return;
|
|
|
|
tick_resume_local();
|
|
}
|
|
|
|
static void xen_vcpu_notify_suspend(void *data)
|
|
{
|
|
tick_suspend_local();
|
|
}
|
|
|
|
void xen_arch_resume(void)
|
|
{
|
|
int cpu;
|
|
|
|
on_each_cpu(xen_vcpu_notify_restore, NULL, 1);
|
|
|
|
for_each_online_cpu(cpu)
|
|
xen_pmu_init(cpu);
|
|
}
|
|
|
|
void xen_arch_suspend(void)
|
|
{
|
|
int cpu;
|
|
|
|
for_each_online_cpu(cpu)
|
|
xen_pmu_finish(cpu);
|
|
|
|
on_each_cpu(xen_vcpu_notify_suspend, NULL, 1);
|
|
}
|