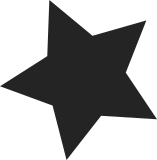
Currently struct names for sysfs are generated only based on the attribute names. This means that attribute names cannot be reused in multiple places throughout the complete btrfs sysfs hierarchy. E.g. allocation/data/total_bytes and allocation/data/single/total_bytes result in the same struct name btrfs_attr_total_bytes. A workaround for this case was made in the past by ad hoc creating an extra macro wrapper, BTRFS_RAID_ATTR, that inserts some extra text in the struct name. Instead of polluting sysfs.h with such kind of extra macro definitions, and only doing so when there are collisions, use a prefix which gets inserted in the struct name, so we keep everything nicely grouped together by default. Current collections of attributes are: * (the toplevel, empty prefix) * allocation * space_info * raid * features Signed-off-by: Hans van Kranenburg <hans.van.kranenburg@mendix.com> Reviewed-by: David Sterba <dsterba@suse.com> Signed-off-by: David Sterba <dsterba@suse.com>
93 lines
3 KiB
C
93 lines
3 KiB
C
#ifndef _BTRFS_SYSFS_H_
|
|
#define _BTRFS_SYSFS_H_
|
|
|
|
/*
|
|
* Data exported through sysfs
|
|
*/
|
|
extern u64 btrfs_debugfs_test;
|
|
|
|
enum btrfs_feature_set {
|
|
FEAT_COMPAT,
|
|
FEAT_COMPAT_RO,
|
|
FEAT_INCOMPAT,
|
|
FEAT_MAX
|
|
};
|
|
|
|
#define __INIT_KOBJ_ATTR(_name, _mode, _show, _store) \
|
|
{ \
|
|
.attr = { .name = __stringify(_name), .mode = _mode }, \
|
|
.show = _show, \
|
|
.store = _store, \
|
|
}
|
|
|
|
#define BTRFS_ATTR_RW(_prefix, _name, _show, _store) \
|
|
static struct kobj_attribute btrfs_attr_##_prefix##_##_name = \
|
|
__INIT_KOBJ_ATTR(_name, 0644, _show, _store)
|
|
|
|
#define BTRFS_ATTR(_prefix, _name, _show) \
|
|
static struct kobj_attribute btrfs_attr_##_prefix##_##_name = \
|
|
__INIT_KOBJ_ATTR(_name, 0444, _show, NULL)
|
|
|
|
#define BTRFS_ATTR_PTR(_prefix, _name) \
|
|
(&btrfs_attr_##_prefix##_##_name.attr)
|
|
|
|
|
|
struct btrfs_feature_attr {
|
|
struct kobj_attribute kobj_attr;
|
|
enum btrfs_feature_set feature_set;
|
|
u64 feature_bit;
|
|
};
|
|
|
|
#define BTRFS_FEAT_ATTR(_name, _feature_set, _feature_prefix, _feature_bit) \
|
|
static struct btrfs_feature_attr btrfs_attr_features_##_name = { \
|
|
.kobj_attr = __INIT_KOBJ_ATTR(_name, S_IRUGO, \
|
|
btrfs_feature_attr_show, \
|
|
btrfs_feature_attr_store), \
|
|
.feature_set = _feature_set, \
|
|
.feature_bit = _feature_prefix ##_## _feature_bit, \
|
|
}
|
|
#define BTRFS_FEAT_ATTR_PTR(_name) \
|
|
(&btrfs_attr_features_##_name.kobj_attr.attr)
|
|
|
|
#define BTRFS_FEAT_ATTR_COMPAT(name, feature) \
|
|
BTRFS_FEAT_ATTR(name, FEAT_COMPAT, BTRFS_FEATURE_COMPAT, feature)
|
|
#define BTRFS_FEAT_ATTR_COMPAT_RO(name, feature) \
|
|
BTRFS_FEAT_ATTR(name, FEAT_COMPAT_RO, BTRFS_FEATURE_COMPAT_RO, feature)
|
|
#define BTRFS_FEAT_ATTR_INCOMPAT(name, feature) \
|
|
BTRFS_FEAT_ATTR(name, FEAT_INCOMPAT, BTRFS_FEATURE_INCOMPAT, feature)
|
|
|
|
/* convert from attribute */
|
|
static inline struct btrfs_feature_attr *
|
|
to_btrfs_feature_attr(struct kobj_attribute *a)
|
|
{
|
|
return container_of(a, struct btrfs_feature_attr, kobj_attr);
|
|
}
|
|
|
|
static inline struct kobj_attribute *attr_to_btrfs_attr(struct attribute *attr)
|
|
{
|
|
return container_of(attr, struct kobj_attribute, attr);
|
|
}
|
|
|
|
static inline struct btrfs_feature_attr *
|
|
attr_to_btrfs_feature_attr(struct attribute *attr)
|
|
{
|
|
return to_btrfs_feature_attr(attr_to_btrfs_attr(attr));
|
|
}
|
|
|
|
char *btrfs_printable_features(enum btrfs_feature_set set, u64 flags);
|
|
extern const char * const btrfs_feature_set_names[3];
|
|
extern struct kobj_type space_info_ktype;
|
|
extern struct kobj_type btrfs_raid_ktype;
|
|
int btrfs_sysfs_add_device_link(struct btrfs_fs_devices *fs_devices,
|
|
struct btrfs_device *one_device);
|
|
int btrfs_sysfs_rm_device_link(struct btrfs_fs_devices *fs_devices,
|
|
struct btrfs_device *one_device);
|
|
int btrfs_sysfs_add_fsid(struct btrfs_fs_devices *fs_devs,
|
|
struct kobject *parent);
|
|
int btrfs_sysfs_add_device(struct btrfs_fs_devices *fs_devs);
|
|
void btrfs_sysfs_remove_fsid(struct btrfs_fs_devices *fs_devs);
|
|
void btrfs_sysfs_feature_update(struct btrfs_fs_info *fs_info,
|
|
u64 bit, enum btrfs_feature_set set);
|
|
|
|
#endif /* _BTRFS_SYSFS_H_ */
|