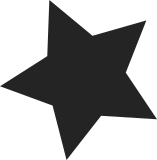
With all the infrastructure in place, we can now have slabinfo_show done from slab_common.c. A cache-specific function is called to grab information about the cache itself, since that is still heavily dependent on the implementation. But with the values produced by it, all the printing and handling is done from common code. Signed-off-by: Glauber Costa <glommer@parallels.com> CC: Christoph Lameter <cl@linux.com> CC: David Rientjes <rientjes@google.com> Signed-off-by: Pekka Enberg <penberg@kernel.org>
71 lines
2 KiB
C
71 lines
2 KiB
C
#ifndef MM_SLAB_H
|
|
#define MM_SLAB_H
|
|
/*
|
|
* Internal slab definitions
|
|
*/
|
|
|
|
/*
|
|
* State of the slab allocator.
|
|
*
|
|
* This is used to describe the states of the allocator during bootup.
|
|
* Allocators use this to gradually bootstrap themselves. Most allocators
|
|
* have the problem that the structures used for managing slab caches are
|
|
* allocated from slab caches themselves.
|
|
*/
|
|
enum slab_state {
|
|
DOWN, /* No slab functionality yet */
|
|
PARTIAL, /* SLUB: kmem_cache_node available */
|
|
PARTIAL_ARRAYCACHE, /* SLAB: kmalloc size for arraycache available */
|
|
PARTIAL_L3, /* SLAB: kmalloc size for l3 struct available */
|
|
UP, /* Slab caches usable but not all extras yet */
|
|
FULL /* Everything is working */
|
|
};
|
|
|
|
extern enum slab_state slab_state;
|
|
|
|
/* The slab cache mutex protects the management structures during changes */
|
|
extern struct mutex slab_mutex;
|
|
|
|
/* The list of all slab caches on the system */
|
|
extern struct list_head slab_caches;
|
|
|
|
/* The slab cache that manages slab cache information */
|
|
extern struct kmem_cache *kmem_cache;
|
|
|
|
/* Functions provided by the slab allocators */
|
|
extern int __kmem_cache_create(struct kmem_cache *, unsigned long flags);
|
|
|
|
#ifdef CONFIG_SLUB
|
|
struct kmem_cache *__kmem_cache_alias(const char *name, size_t size,
|
|
size_t align, unsigned long flags, void (*ctor)(void *));
|
|
#else
|
|
static inline struct kmem_cache *__kmem_cache_alias(const char *name, size_t size,
|
|
size_t align, unsigned long flags, void (*ctor)(void *))
|
|
{ return NULL; }
|
|
#endif
|
|
|
|
|
|
int __kmem_cache_shutdown(struct kmem_cache *);
|
|
|
|
struct seq_file;
|
|
struct file;
|
|
|
|
struct slabinfo {
|
|
unsigned long active_objs;
|
|
unsigned long num_objs;
|
|
unsigned long active_slabs;
|
|
unsigned long num_slabs;
|
|
unsigned long shared_avail;
|
|
unsigned int limit;
|
|
unsigned int batchcount;
|
|
unsigned int shared;
|
|
unsigned int objects_per_slab;
|
|
unsigned int cache_order;
|
|
};
|
|
|
|
void get_slabinfo(struct kmem_cache *s, struct slabinfo *sinfo);
|
|
void slabinfo_show_stats(struct seq_file *m, struct kmem_cache *s);
|
|
ssize_t slabinfo_write(struct file *file, const char __user *buffer,
|
|
size_t count, loff_t *ppos);
|
|
#endif
|