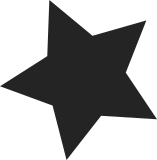
This patch is part of a larger patch series which will remove the "char bus_id[20]" name string from struct device. The device name is managed in the kobject anyway, and without any size limitation, and just needlessly copied into "struct device". To set and read the device name dev_name(dev) and dev_set_name(dev) must be used. If your code uses static kobjects, which it shouldn't do, "const char *init_name" can be used to statically provide the name the registered device should have. At registration time, the init_name field is cleared, to enforce the use of dev_name(dev) to access the device name at a later time. We need to get rid of all occurrences of bus_id in the entire tree to be able to enable the new interface. Please apply this patch, and possibly convert any remaining remaining occurrences of bus_id. Acked-by: Greg Kroah-Hartman <gregkh@suse.de> Signed-Off-By: Kay Sievers <kay.sievers@vrfy.org> Signed-off-by: Jesse Barnes <jbarnes@virtuousgeek.org>
61 lines
1.8 KiB
C
61 lines
1.8 KiB
C
/*
|
|
* PCI IRQ failure handing code
|
|
*
|
|
* Copyright (c) 2008 James Bottomley <James.Bottomley@HansenPartnership.com>
|
|
*/
|
|
|
|
#include <linux/acpi.h>
|
|
#include <linux/device.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/pci.h>
|
|
|
|
static void pci_note_irq_problem(struct pci_dev *pdev, const char *reason)
|
|
{
|
|
struct pci_dev *parent = to_pci_dev(pdev->dev.parent);
|
|
|
|
dev_printk(KERN_ERR, &pdev->dev,
|
|
"Potentially misrouted IRQ (Bridge %s %04x:%04x)\n",
|
|
dev_name(&parent->dev), parent->vendor, parent->device);
|
|
dev_printk(KERN_ERR, &pdev->dev, "%s\n", reason);
|
|
dev_printk(KERN_ERR, &pdev->dev, "Please report to linux-kernel@vger.kernel.org\n");
|
|
WARN_ON(1);
|
|
}
|
|
|
|
/**
|
|
* pci_lost_interrupt - reports a lost PCI interrupt
|
|
* @pdev: device whose interrupt is lost
|
|
*
|
|
* The primary function of this routine is to report a lost interrupt
|
|
* in a standard way which users can recognise (instead of blaming the
|
|
* driver).
|
|
*
|
|
* Returns:
|
|
* a suggestion for fixing it (although the driver is not required to
|
|
* act on this).
|
|
*/
|
|
enum pci_lost_interrupt_reason pci_lost_interrupt(struct pci_dev *pdev)
|
|
{
|
|
if (pdev->msi_enabled || pdev->msix_enabled) {
|
|
enum pci_lost_interrupt_reason ret;
|
|
|
|
if (pdev->msix_enabled) {
|
|
pci_note_irq_problem(pdev, "MSIX routing failure");
|
|
ret = PCI_LOST_IRQ_DISABLE_MSIX;
|
|
} else {
|
|
pci_note_irq_problem(pdev, "MSI routing failure");
|
|
ret = PCI_LOST_IRQ_DISABLE_MSI;
|
|
}
|
|
return ret;
|
|
}
|
|
#ifdef CONFIG_ACPI
|
|
if (!(acpi_disabled || acpi_noirq)) {
|
|
pci_note_irq_problem(pdev, "Potential ACPI misrouting please reboot with acpi=noirq");
|
|
/* currently no way to fix acpi on the fly */
|
|
return PCI_LOST_IRQ_DISABLE_ACPI;
|
|
}
|
|
#endif
|
|
pci_note_irq_problem(pdev, "unknown cause (not MSI or ACPI)");
|
|
return PCI_LOST_IRQ_NO_INFORMATION;
|
|
}
|
|
EXPORT_SYMBOL(pci_lost_interrupt);
|