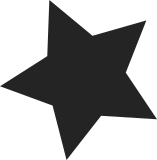
The input argument to rtc_time_to_tm() is unsigned as well as are members of the output structure. However signed arithmetic is used within for calculations leading to incorrect results for input values outside the signed positive range. If this happens the time of day returned is out of range. Found the problem when fiddling with the RTC and the driver where year was set to an unexpectedly large value like 2070, e.g.: rtc0: setting system clock to 2070-01-01 1193046:71582832:26 UTC (3155760954) while it should be: rtc0: setting system clock to 2070-01-01 00:15:54 UTC (3155760954) Changing types to unsigned fixes the problem. [akpm@linux-foundation.org: remove old-fashioned `register' keyword] Signed-off-by: Maciej W. Rozycki <macro@linux-mips.org> Cc: Alessandro Zummo <a.zummo@towertech.it> Cc: David Brownell <david-b@pacbell.net> Cc: Dmitri Vorobiev <dmitri.vorobiev@gmail.com> Cc: <stable@kernel.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
121 lines
2.9 KiB
C
121 lines
2.9 KiB
C
/*
|
|
* rtc and date/time utility functions
|
|
*
|
|
* Copyright (C) 2005-06 Tower Technologies
|
|
* Author: Alessandro Zummo <a.zummo@towertech.it>
|
|
*
|
|
* based on arch/arm/common/rtctime.c and other bits
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/rtc.h>
|
|
|
|
static const unsigned char rtc_days_in_month[] = {
|
|
31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31
|
|
};
|
|
|
|
static const unsigned short rtc_ydays[2][13] = {
|
|
/* Normal years */
|
|
{ 0, 31, 59, 90, 120, 151, 181, 212, 243, 273, 304, 334, 365 },
|
|
/* Leap years */
|
|
{ 0, 31, 60, 91, 121, 152, 182, 213, 244, 274, 305, 335, 366 }
|
|
};
|
|
|
|
#define LEAPS_THRU_END_OF(y) ((y)/4 - (y)/100 + (y)/400)
|
|
#define LEAP_YEAR(year) ((!(year % 4) && (year % 100)) || !(year % 400))
|
|
|
|
/*
|
|
* The number of days in the month.
|
|
*/
|
|
int rtc_month_days(unsigned int month, unsigned int year)
|
|
{
|
|
return rtc_days_in_month[month] + (LEAP_YEAR(year) && month == 1);
|
|
}
|
|
EXPORT_SYMBOL(rtc_month_days);
|
|
|
|
/*
|
|
* The number of days since January 1. (0 to 365)
|
|
*/
|
|
int rtc_year_days(unsigned int day, unsigned int month, unsigned int year)
|
|
{
|
|
return rtc_ydays[LEAP_YEAR(year)][month] + day-1;
|
|
}
|
|
EXPORT_SYMBOL(rtc_year_days);
|
|
|
|
/*
|
|
* Convert seconds since 01-01-1970 00:00:00 to Gregorian date.
|
|
*/
|
|
void rtc_time_to_tm(unsigned long time, struct rtc_time *tm)
|
|
{
|
|
unsigned int days, month, year;
|
|
|
|
days = time / 86400;
|
|
time -= days * 86400;
|
|
|
|
/* day of the week, 1970-01-01 was a Thursday */
|
|
tm->tm_wday = (days + 4) % 7;
|
|
|
|
year = 1970 + days / 365;
|
|
days -= (year - 1970) * 365
|
|
+ LEAPS_THRU_END_OF(year - 1)
|
|
- LEAPS_THRU_END_OF(1970 - 1);
|
|
if (days < 0) {
|
|
year -= 1;
|
|
days += 365 + LEAP_YEAR(year);
|
|
}
|
|
tm->tm_year = year - 1900;
|
|
tm->tm_yday = days + 1;
|
|
|
|
for (month = 0; month < 11; month++) {
|
|
int newdays;
|
|
|
|
newdays = days - rtc_month_days(month, year);
|
|
if (newdays < 0)
|
|
break;
|
|
days = newdays;
|
|
}
|
|
tm->tm_mon = month;
|
|
tm->tm_mday = days + 1;
|
|
|
|
tm->tm_hour = time / 3600;
|
|
time -= tm->tm_hour * 3600;
|
|
tm->tm_min = time / 60;
|
|
tm->tm_sec = time - tm->tm_min * 60;
|
|
}
|
|
EXPORT_SYMBOL(rtc_time_to_tm);
|
|
|
|
/*
|
|
* Does the rtc_time represent a valid date/time?
|
|
*/
|
|
int rtc_valid_tm(struct rtc_time *tm)
|
|
{
|
|
if (tm->tm_year < 70
|
|
|| ((unsigned)tm->tm_mon) >= 12
|
|
|| tm->tm_mday < 1
|
|
|| tm->tm_mday > rtc_month_days(tm->tm_mon, tm->tm_year + 1900)
|
|
|| ((unsigned)tm->tm_hour) >= 24
|
|
|| ((unsigned)tm->tm_min) >= 60
|
|
|| ((unsigned)tm->tm_sec) >= 60)
|
|
return -EINVAL;
|
|
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(rtc_valid_tm);
|
|
|
|
/*
|
|
* Convert Gregorian date to seconds since 01-01-1970 00:00:00.
|
|
*/
|
|
int rtc_tm_to_time(struct rtc_time *tm, unsigned long *time)
|
|
{
|
|
*time = mktime(tm->tm_year + 1900, tm->tm_mon + 1, tm->tm_mday,
|
|
tm->tm_hour, tm->tm_min, tm->tm_sec);
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(rtc_tm_to_time);
|
|
|
|
MODULE_LICENSE("GPL");
|