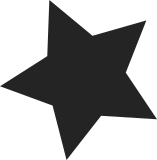
If we are soft disabled and receive a doorbell exception we don't process it immediately. This means we need to check on the way out of irq restore if there are any doorbell exceptions to process. The problem is at that point we don't know what our regs are, and that in turn makes xmon unhappy. To workaround the problem, instead of checking for and processing doorbells, we check for any doorbells and if there were any we send ourselves another. Signed-off-by: Michael Ellerman <michael@ellerman.id.au> Signed-off-by: Benjamin Herrenschmidt <benh@kernel.crashing.org>
44 lines
1.3 KiB
C
44 lines
1.3 KiB
C
/*
|
|
* Copyright 2009 Freescale Semicondutor, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*
|
|
* provides masks and opcode images for use by code generation, emulation
|
|
* and for instructions that older assemblers might not know about
|
|
*/
|
|
#ifndef _ASM_POWERPC_DBELL_H
|
|
#define _ASM_POWERPC_DBELL_H
|
|
|
|
#include <linux/smp.h>
|
|
#include <linux/threads.h>
|
|
|
|
#include <asm/ppc-opcode.h>
|
|
|
|
#define PPC_DBELL_MSG_BRDCAST (0x04000000)
|
|
#define PPC_DBELL_TYPE(x) (((x) & 0xf) << 28)
|
|
enum ppc_dbell {
|
|
PPC_DBELL = 0, /* doorbell */
|
|
PPC_DBELL_CRIT = 1, /* critical doorbell */
|
|
PPC_G_DBELL = 2, /* guest doorbell */
|
|
PPC_G_DBELL_CRIT = 3, /* guest critical doorbell */
|
|
PPC_G_DBELL_MC = 4, /* guest mcheck doorbell */
|
|
};
|
|
|
|
extern void doorbell_message_pass(int target, int msg);
|
|
extern void doorbell_exception(struct pt_regs *regs);
|
|
extern void doorbell_check_self(void);
|
|
extern void doorbell_setup_this_cpu(void);
|
|
|
|
static inline void ppc_msgsnd(enum ppc_dbell type, u32 flags, u32 tag)
|
|
{
|
|
u32 msg = PPC_DBELL_TYPE(type) | (flags & PPC_DBELL_MSG_BRDCAST) |
|
|
(tag & 0x07ffffff);
|
|
|
|
__asm__ __volatile__ (PPC_MSGSND(%0) : : "r" (msg));
|
|
}
|
|
|
|
#endif /* _ASM_POWERPC_DBELL_H */
|