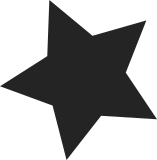
On powerpc64 machines running 32-bit userspace, we can get garbage bits in the stack pointer passed into the kernel. Most places handle this correctly, but the signal handling code uses the passed value directly for allocating signal stack frames. This fixes the issue by introducing a get_clean_sp function that returns a sanitized stack pointer. For 32-bit tasks on a 64-bit kernel, the stack pointer is masked correctly. In all other cases, the stack pointer is simply returned. Additionally, we pass an 'is_32' parameter to get_sigframe now in order to get the properly sanitized stack. The callers are know to be 32 or 64-bit statically. Signed-off-by: Josh Boyer <jwboyer@linux.vnet.ibm.com> Signed-off-by: Benjamin Herrenschmidt <benh@kernel.crashing.org>
58 lines
1.7 KiB
C
58 lines
1.7 KiB
C
/*
|
|
* Copyright (c) 2007 Benjamin Herrenschmidt, IBM Coproration
|
|
* Extracted from signal_32.c and signal_64.c
|
|
*
|
|
* This file is subject to the terms and conditions of the GNU General
|
|
* Public License. See the file README.legal in the main directory of
|
|
* this archive for more details.
|
|
*/
|
|
|
|
#ifndef _POWERPC_ARCH_SIGNAL_H
|
|
#define _POWERPC_ARCH_SIGNAL_H
|
|
|
|
#define _BLOCKABLE (~(sigmask(SIGKILL) | sigmask(SIGSTOP)))
|
|
|
|
extern void do_signal(struct pt_regs *regs, unsigned long thread_info_flags);
|
|
|
|
extern void __user * get_sigframe(struct k_sigaction *ka, struct pt_regs *regs,
|
|
size_t frame_size, int is_32);
|
|
extern void restore_sigmask(sigset_t *set);
|
|
|
|
extern int handle_signal32(unsigned long sig, struct k_sigaction *ka,
|
|
siginfo_t *info, sigset_t *oldset,
|
|
struct pt_regs *regs);
|
|
|
|
extern int handle_rt_signal32(unsigned long sig, struct k_sigaction *ka,
|
|
siginfo_t *info, sigset_t *oldset,
|
|
struct pt_regs *regs);
|
|
|
|
extern unsigned long copy_fpr_to_user(void __user *to,
|
|
struct task_struct *task);
|
|
extern unsigned long copy_fpr_from_user(struct task_struct *task,
|
|
void __user *from);
|
|
#ifdef CONFIG_VSX
|
|
extern unsigned long copy_vsx_to_user(void __user *to,
|
|
struct task_struct *task);
|
|
extern unsigned long copy_vsx_from_user(struct task_struct *task,
|
|
void __user *from);
|
|
#endif
|
|
|
|
#ifdef CONFIG_PPC64
|
|
|
|
extern int handle_rt_signal64(int signr, struct k_sigaction *ka,
|
|
siginfo_t *info, sigset_t *set,
|
|
struct pt_regs *regs);
|
|
|
|
#else /* CONFIG_PPC64 */
|
|
|
|
static inline int handle_rt_signal64(int signr, struct k_sigaction *ka,
|
|
siginfo_t *info, sigset_t *set,
|
|
struct pt_regs *regs)
|
|
{
|
|
return -EFAULT;
|
|
}
|
|
|
|
#endif /* !defined(CONFIG_PPC64) */
|
|
|
|
#endif /* _POWERPC_ARCH_SIGNAL_H */
|