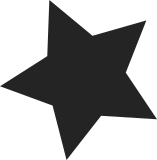
Bridge will OOPS on removal if other application has the SAP open. The bridge SAP might be shared with other usages, so need to do reference counting on module removal rather than explicit close/delete. Since packet might arrive after or during removal, need to clear the receive function handle, so LLC only hands it to user (if any). Signed-off-by: Stephen Hemminger <shemminger@osdl.org> Signed-off-by: David S. Miller <davem@davemloft.net>
84 lines
1.7 KiB
C
84 lines
1.7 KiB
C
/*
|
|
* Generic parts
|
|
* Linux ethernet bridge
|
|
*
|
|
* Authors:
|
|
* Lennert Buytenhek <buytenh@gnu.org>
|
|
*
|
|
* $Id: br.c,v 1.47 2001/12/24 00:56:41 davem Exp $
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/config.h>
|
|
#include <linux/module.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/etherdevice.h>
|
|
#include <linux/init.h>
|
|
#include <linux/llc.h>
|
|
#include <net/llc.h>
|
|
|
|
#include "br_private.h"
|
|
|
|
int (*br_should_route_hook) (struct sk_buff **pskb) = NULL;
|
|
|
|
static struct llc_sap *br_stp_sap;
|
|
|
|
static int __init br_init(void)
|
|
{
|
|
br_stp_sap = llc_sap_open(LLC_SAP_BSPAN, br_stp_rcv);
|
|
if (!br_stp_sap) {
|
|
printk(KERN_ERR "bridge: can't register sap for STP\n");
|
|
return -EBUSY;
|
|
}
|
|
|
|
br_fdb_init();
|
|
|
|
#ifdef CONFIG_BRIDGE_NETFILTER
|
|
if (br_netfilter_init())
|
|
return 1;
|
|
#endif
|
|
brioctl_set(br_ioctl_deviceless_stub);
|
|
br_handle_frame_hook = br_handle_frame;
|
|
|
|
br_fdb_get_hook = br_fdb_get;
|
|
br_fdb_put_hook = br_fdb_put;
|
|
|
|
register_netdevice_notifier(&br_device_notifier);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void __exit br_deinit(void)
|
|
{
|
|
rcu_assign_pointer(br_stp_sap->rcv_func, NULL);
|
|
|
|
#ifdef CONFIG_BRIDGE_NETFILTER
|
|
br_netfilter_fini();
|
|
#endif
|
|
unregister_netdevice_notifier(&br_device_notifier);
|
|
brioctl_set(NULL);
|
|
|
|
br_cleanup_bridges();
|
|
|
|
synchronize_net();
|
|
|
|
llc_sap_put(br_stp_sap);
|
|
br_fdb_get_hook = NULL;
|
|
br_fdb_put_hook = NULL;
|
|
|
|
br_handle_frame_hook = NULL;
|
|
br_fdb_fini();
|
|
}
|
|
|
|
EXPORT_SYMBOL(br_should_route_hook);
|
|
|
|
module_init(br_init)
|
|
module_exit(br_deinit)
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_VERSION(BR_VERSION);
|