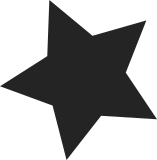
This marks many critical kernel structures for randomization. These are structures that have been targeted in the past in security exploits, or contain functions pointers, pointers to function pointer tables, lists, workqueues, ref-counters, credentials, permissions, or are otherwise sensitive. This initial list was extracted from Brad Spengler/PaX Team's code in the last public patch of grsecurity/PaX based on my understanding of the code. Changes or omissions from the original code are mine and don't reflect the original grsecurity/PaX code. Left out of this list is task_struct, which requires special handling and will be covered in a subsequent patch. Signed-off-by: Kees Cook <keescook@chromium.org>
111 lines
2.5 KiB
C
111 lines
2.5 KiB
C
#ifndef _LINUX_PID_NS_H
|
|
#define _LINUX_PID_NS_H
|
|
|
|
#include <linux/sched.h>
|
|
#include <linux/bug.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/workqueue.h>
|
|
#include <linux/threads.h>
|
|
#include <linux/nsproxy.h>
|
|
#include <linux/kref.h>
|
|
#include <linux/ns_common.h>
|
|
|
|
struct pidmap {
|
|
atomic_t nr_free;
|
|
void *page;
|
|
};
|
|
|
|
#define BITS_PER_PAGE (PAGE_SIZE * 8)
|
|
#define BITS_PER_PAGE_MASK (BITS_PER_PAGE-1)
|
|
#define PIDMAP_ENTRIES ((PID_MAX_LIMIT+BITS_PER_PAGE-1)/BITS_PER_PAGE)
|
|
|
|
struct fs_pin;
|
|
|
|
enum { /* definitions for pid_namespace's hide_pid field */
|
|
HIDEPID_OFF = 0,
|
|
HIDEPID_NO_ACCESS = 1,
|
|
HIDEPID_INVISIBLE = 2,
|
|
};
|
|
|
|
struct pid_namespace {
|
|
struct kref kref;
|
|
struct pidmap pidmap[PIDMAP_ENTRIES];
|
|
struct rcu_head rcu;
|
|
int last_pid;
|
|
unsigned int nr_hashed;
|
|
struct task_struct *child_reaper;
|
|
struct kmem_cache *pid_cachep;
|
|
unsigned int level;
|
|
struct pid_namespace *parent;
|
|
#ifdef CONFIG_PROC_FS
|
|
struct vfsmount *proc_mnt;
|
|
struct dentry *proc_self;
|
|
struct dentry *proc_thread_self;
|
|
#endif
|
|
#ifdef CONFIG_BSD_PROCESS_ACCT
|
|
struct fs_pin *bacct;
|
|
#endif
|
|
struct user_namespace *user_ns;
|
|
struct ucounts *ucounts;
|
|
struct work_struct proc_work;
|
|
kgid_t pid_gid;
|
|
int hide_pid;
|
|
int reboot; /* group exit code if this pidns was rebooted */
|
|
struct ns_common ns;
|
|
} __randomize_layout;
|
|
|
|
extern struct pid_namespace init_pid_ns;
|
|
|
|
#define PIDNS_HASH_ADDING (1U << 31)
|
|
|
|
#ifdef CONFIG_PID_NS
|
|
static inline struct pid_namespace *get_pid_ns(struct pid_namespace *ns)
|
|
{
|
|
if (ns != &init_pid_ns)
|
|
kref_get(&ns->kref);
|
|
return ns;
|
|
}
|
|
|
|
extern struct pid_namespace *copy_pid_ns(unsigned long flags,
|
|
struct user_namespace *user_ns, struct pid_namespace *ns);
|
|
extern void zap_pid_ns_processes(struct pid_namespace *pid_ns);
|
|
extern int reboot_pid_ns(struct pid_namespace *pid_ns, int cmd);
|
|
extern void put_pid_ns(struct pid_namespace *ns);
|
|
|
|
#else /* !CONFIG_PID_NS */
|
|
#include <linux/err.h>
|
|
|
|
static inline struct pid_namespace *get_pid_ns(struct pid_namespace *ns)
|
|
{
|
|
return ns;
|
|
}
|
|
|
|
static inline struct pid_namespace *copy_pid_ns(unsigned long flags,
|
|
struct user_namespace *user_ns, struct pid_namespace *ns)
|
|
{
|
|
if (flags & CLONE_NEWPID)
|
|
ns = ERR_PTR(-EINVAL);
|
|
return ns;
|
|
}
|
|
|
|
static inline void put_pid_ns(struct pid_namespace *ns)
|
|
{
|
|
}
|
|
|
|
static inline void zap_pid_ns_processes(struct pid_namespace *ns)
|
|
{
|
|
BUG();
|
|
}
|
|
|
|
static inline int reboot_pid_ns(struct pid_namespace *pid_ns, int cmd)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif /* CONFIG_PID_NS */
|
|
|
|
extern struct pid_namespace *task_active_pid_ns(struct task_struct *tsk);
|
|
void pidhash_init(void);
|
|
void pidmap_init(void);
|
|
|
|
#endif /* _LINUX_PID_NS_H */
|