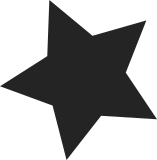
Now that we don't pass double skb pointers to nf_hook_slow anymore, gcc can generate tail calls for some of the netfilter hook okfn invocations, so there is no need to inline the functions anymore. This caused huge code bloat since we ended up with one inlined version and one out-of-line version since we pass the address to nf_hook_slow. Before: text data bss dec hex filename 8997385 1016524 524652 10538561 a0ce41 vmlinux After: text data bss dec hex filename 8994009 1016524 524652 10535185 a0c111 vmlinux ------------------------------------------------------- -3376 All cases have been verified to generate tail-calls with and without netfilter. The okfns in ipmr and xfrm4_input still remain inline because gcc can't generate tail-calls for them. Signed-off-by: Patrick McHardy <kaber@trash.net> Signed-off-by: David S. Miller <davem@davemloft.net>
131 lines
3.2 KiB
C
131 lines
3.2 KiB
C
/*
|
|
* INET An implementation of the TCP/IP protocol suite for the LINUX
|
|
* operating system. INET is implemented using the BSD Socket
|
|
* interface as the means of communication with the user level.
|
|
*
|
|
* The IP forwarding functionality.
|
|
*
|
|
* Version: $Id: ip_forward.c,v 1.48 2000/12/13 18:31:48 davem Exp $
|
|
*
|
|
* Authors: see ip.c
|
|
*
|
|
* Fixes:
|
|
* Many : Split from ip.c , see ip_input.c for
|
|
* history.
|
|
* Dave Gregorich : NULL ip_rt_put fix for multicast
|
|
* routing.
|
|
* Jos Vos : Add call_out_firewall before sending,
|
|
* use output device for accounting.
|
|
* Jos Vos : Call forward firewall after routing
|
|
* (always use output device).
|
|
* Mike McLagan : Routing by source
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/ip.h>
|
|
#include <linux/icmp.h>
|
|
#include <linux/netdevice.h>
|
|
#include <net/sock.h>
|
|
#include <net/ip.h>
|
|
#include <net/tcp.h>
|
|
#include <net/udp.h>
|
|
#include <net/icmp.h>
|
|
#include <linux/tcp.h>
|
|
#include <linux/udp.h>
|
|
#include <linux/netfilter_ipv4.h>
|
|
#include <net/checksum.h>
|
|
#include <linux/route.h>
|
|
#include <net/route.h>
|
|
#include <net/xfrm.h>
|
|
|
|
static int ip_forward_finish(struct sk_buff *skb)
|
|
{
|
|
struct ip_options * opt = &(IPCB(skb)->opt);
|
|
|
|
IP_INC_STATS_BH(IPSTATS_MIB_OUTFORWDATAGRAMS);
|
|
|
|
if (unlikely(opt->optlen))
|
|
ip_forward_options(skb);
|
|
|
|
return dst_output(skb);
|
|
}
|
|
|
|
int ip_forward(struct sk_buff *skb)
|
|
{
|
|
struct iphdr *iph; /* Our header */
|
|
struct rtable *rt; /* Route we use */
|
|
struct ip_options * opt = &(IPCB(skb)->opt);
|
|
|
|
if (!xfrm4_policy_check(NULL, XFRM_POLICY_FWD, skb))
|
|
goto drop;
|
|
|
|
if (IPCB(skb)->opt.router_alert && ip_call_ra_chain(skb))
|
|
return NET_RX_SUCCESS;
|
|
|
|
if (skb->pkt_type != PACKET_HOST)
|
|
goto drop;
|
|
|
|
skb_forward_csum(skb);
|
|
|
|
/*
|
|
* According to the RFC, we must first decrease the TTL field. If
|
|
* that reaches zero, we must reply an ICMP control message telling
|
|
* that the packet's lifetime expired.
|
|
*/
|
|
if (ip_hdr(skb)->ttl <= 1)
|
|
goto too_many_hops;
|
|
|
|
if (!xfrm4_route_forward(skb))
|
|
goto drop;
|
|
|
|
rt = (struct rtable*)skb->dst;
|
|
|
|
if (opt->is_strictroute && rt->rt_dst != rt->rt_gateway)
|
|
goto sr_failed;
|
|
|
|
if (unlikely(skb->len > dst_mtu(&rt->u.dst) &&
|
|
(ip_hdr(skb)->frag_off & htons(IP_DF))) && !skb->local_df) {
|
|
IP_INC_STATS(IPSTATS_MIB_FRAGFAILS);
|
|
icmp_send(skb, ICMP_DEST_UNREACH, ICMP_FRAG_NEEDED,
|
|
htonl(dst_mtu(&rt->u.dst)));
|
|
goto drop;
|
|
}
|
|
|
|
/* We are about to mangle packet. Copy it! */
|
|
if (skb_cow(skb, LL_RESERVED_SPACE(rt->u.dst.dev)+rt->u.dst.header_len))
|
|
goto drop;
|
|
iph = ip_hdr(skb);
|
|
|
|
/* Decrease ttl after skb cow done */
|
|
ip_decrease_ttl(iph);
|
|
|
|
/*
|
|
* We now generate an ICMP HOST REDIRECT giving the route
|
|
* we calculated.
|
|
*/
|
|
if (rt->rt_flags&RTCF_DOREDIRECT && !opt->srr && !skb->sp)
|
|
ip_rt_send_redirect(skb);
|
|
|
|
skb->priority = rt_tos2priority(iph->tos);
|
|
|
|
return NF_HOOK(PF_INET, NF_IP_FORWARD, skb, skb->dev, rt->u.dst.dev,
|
|
ip_forward_finish);
|
|
|
|
sr_failed:
|
|
/*
|
|
* Strict routing permits no gatewaying
|
|
*/
|
|
icmp_send(skb, ICMP_DEST_UNREACH, ICMP_SR_FAILED, 0);
|
|
goto drop;
|
|
|
|
too_many_hops:
|
|
/* Tell the sender its packet died... */
|
|
IP_INC_STATS_BH(IPSTATS_MIB_INHDRERRORS);
|
|
icmp_send(skb, ICMP_TIME_EXCEEDED, ICMP_EXC_TTL, 0);
|
|
drop:
|
|
kfree_skb(skb);
|
|
return NET_RX_DROP;
|
|
}
|