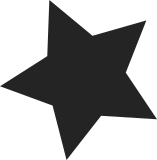
DECLARE_MUTEX_LOCKED was used for semaphores used as completions and we've got rid of them. Well, except for one in libusual that the maintainer explicitly wants to keep as semaphore. So convert that useage to an explicit sema_init and kill of DECLARE_MUTEX_LOCKED so that new code is reminded to use a completion. Signed-off-by: Christoph Hellwig <hch@lst.de> Acked-by: "Satyam Sharma" <satyam.sharma@gmail.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
100 lines
2.1 KiB
C
100 lines
2.1 KiB
C
#ifndef _ASM_IA64_SEMAPHORE_H
|
|
#define _ASM_IA64_SEMAPHORE_H
|
|
|
|
/*
|
|
* Copyright (C) 1998-2000 Hewlett-Packard Co
|
|
* Copyright (C) 1998-2000 David Mosberger-Tang <davidm@hpl.hp.com>
|
|
*/
|
|
|
|
#include <linux/wait.h>
|
|
#include <linux/rwsem.h>
|
|
|
|
#include <asm/atomic.h>
|
|
|
|
struct semaphore {
|
|
atomic_t count;
|
|
int sleepers;
|
|
wait_queue_head_t wait;
|
|
};
|
|
|
|
#define __SEMAPHORE_INITIALIZER(name, n) \
|
|
{ \
|
|
.count = ATOMIC_INIT(n), \
|
|
.sleepers = 0, \
|
|
.wait = __WAIT_QUEUE_HEAD_INITIALIZER((name).wait) \
|
|
}
|
|
|
|
#define __DECLARE_SEMAPHORE_GENERIC(name,count) \
|
|
struct semaphore name = __SEMAPHORE_INITIALIZER(name, count)
|
|
|
|
#define DECLARE_MUTEX(name) __DECLARE_SEMAPHORE_GENERIC(name, 1)
|
|
|
|
static inline void
|
|
sema_init (struct semaphore *sem, int val)
|
|
{
|
|
*sem = (struct semaphore) __SEMAPHORE_INITIALIZER(*sem, val);
|
|
}
|
|
|
|
static inline void
|
|
init_MUTEX (struct semaphore *sem)
|
|
{
|
|
sema_init(sem, 1);
|
|
}
|
|
|
|
static inline void
|
|
init_MUTEX_LOCKED (struct semaphore *sem)
|
|
{
|
|
sema_init(sem, 0);
|
|
}
|
|
|
|
extern void __down (struct semaphore * sem);
|
|
extern int __down_interruptible (struct semaphore * sem);
|
|
extern int __down_trylock (struct semaphore * sem);
|
|
extern void __up (struct semaphore * sem);
|
|
|
|
/*
|
|
* Atomically decrement the semaphore's count. If it goes negative,
|
|
* block the calling thread in the TASK_UNINTERRUPTIBLE state.
|
|
*/
|
|
static inline void
|
|
down (struct semaphore *sem)
|
|
{
|
|
might_sleep();
|
|
if (ia64_fetchadd(-1, &sem->count.counter, acq) < 1)
|
|
__down(sem);
|
|
}
|
|
|
|
/*
|
|
* Atomically decrement the semaphore's count. If it goes negative,
|
|
* block the calling thread in the TASK_INTERRUPTIBLE state.
|
|
*/
|
|
static inline int
|
|
down_interruptible (struct semaphore * sem)
|
|
{
|
|
int ret = 0;
|
|
|
|
might_sleep();
|
|
if (ia64_fetchadd(-1, &sem->count.counter, acq) < 1)
|
|
ret = __down_interruptible(sem);
|
|
return ret;
|
|
}
|
|
|
|
static inline int
|
|
down_trylock (struct semaphore *sem)
|
|
{
|
|
int ret = 0;
|
|
|
|
if (ia64_fetchadd(-1, &sem->count.counter, acq) < 1)
|
|
ret = __down_trylock(sem);
|
|
return ret;
|
|
}
|
|
|
|
static inline void
|
|
up (struct semaphore * sem)
|
|
{
|
|
if (ia64_fetchadd(1, &sem->count.counter, rel) <= -1)
|
|
__up(sem);
|
|
}
|
|
|
|
#endif /* _ASM_IA64_SEMAPHORE_H */
|