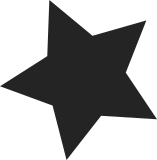
DECLARE_MUTEX_LOCKED was used for semaphores used as completions and we've got rid of them. Well, except for one in libusual that the maintainer explicitly wants to keep as semaphore. So convert that useage to an explicit sema_init and kill of DECLARE_MUTEX_LOCKED so that new code is reminded to use a completion. Signed-off-by: Christoph Hellwig <hch@lst.de> Acked-by: "Satyam Sharma" <satyam.sharma@gmail.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
116 lines
2.6 KiB
C
116 lines
2.6 KiB
C
#ifndef __ASM_SH_SEMAPHORE_H
|
|
#define __ASM_SH_SEMAPHORE_H
|
|
|
|
#include <linux/linkage.h>
|
|
|
|
#ifdef __KERNEL__
|
|
/*
|
|
* SMP- and interrupt-safe semaphores.
|
|
*
|
|
* (C) Copyright 1996 Linus Torvalds
|
|
*
|
|
* SuperH verison by Niibe Yutaka
|
|
* (Currently no asm implementation but generic C code...)
|
|
*/
|
|
|
|
#include <linux/spinlock.h>
|
|
#include <linux/rwsem.h>
|
|
#include <linux/wait.h>
|
|
|
|
#include <asm/system.h>
|
|
#include <asm/atomic.h>
|
|
|
|
struct semaphore {
|
|
atomic_t count;
|
|
int sleepers;
|
|
wait_queue_head_t wait;
|
|
};
|
|
|
|
#define __SEMAPHORE_INITIALIZER(name, n) \
|
|
{ \
|
|
.count = ATOMIC_INIT(n), \
|
|
.sleepers = 0, \
|
|
.wait = __WAIT_QUEUE_HEAD_INITIALIZER((name).wait) \
|
|
}
|
|
|
|
#define __DECLARE_SEMAPHORE_GENERIC(name,count) \
|
|
struct semaphore name = __SEMAPHORE_INITIALIZER(name,count)
|
|
|
|
#define DECLARE_MUTEX(name) __DECLARE_SEMAPHORE_GENERIC(name,1)
|
|
|
|
static inline void sema_init (struct semaphore *sem, int val)
|
|
{
|
|
/*
|
|
* *sem = (struct semaphore)__SEMAPHORE_INITIALIZER((*sem),val);
|
|
*
|
|
* i'd rather use the more flexible initialization above, but sadly
|
|
* GCC 2.7.2.3 emits a bogus warning. EGCS doesn't. Oh well.
|
|
*/
|
|
atomic_set(&sem->count, val);
|
|
sem->sleepers = 0;
|
|
init_waitqueue_head(&sem->wait);
|
|
}
|
|
|
|
static inline void init_MUTEX (struct semaphore *sem)
|
|
{
|
|
sema_init(sem, 1);
|
|
}
|
|
|
|
static inline void init_MUTEX_LOCKED (struct semaphore *sem)
|
|
{
|
|
sema_init(sem, 0);
|
|
}
|
|
|
|
#if 0
|
|
asmlinkage void __down_failed(void /* special register calling convention */);
|
|
asmlinkage int __down_failed_interruptible(void /* params in registers */);
|
|
asmlinkage int __down_failed_trylock(void /* params in registers */);
|
|
asmlinkage void __up_wakeup(void /* special register calling convention */);
|
|
#endif
|
|
|
|
asmlinkage void __down(struct semaphore * sem);
|
|
asmlinkage int __down_interruptible(struct semaphore * sem);
|
|
asmlinkage int __down_trylock(struct semaphore * sem);
|
|
asmlinkage void __up(struct semaphore * sem);
|
|
|
|
extern spinlock_t semaphore_wake_lock;
|
|
|
|
static inline void down(struct semaphore * sem)
|
|
{
|
|
might_sleep();
|
|
if (atomic_dec_return(&sem->count) < 0)
|
|
__down(sem);
|
|
}
|
|
|
|
static inline int down_interruptible(struct semaphore * sem)
|
|
{
|
|
int ret = 0;
|
|
|
|
might_sleep();
|
|
if (atomic_dec_return(&sem->count) < 0)
|
|
ret = __down_interruptible(sem);
|
|
return ret;
|
|
}
|
|
|
|
static inline int down_trylock(struct semaphore * sem)
|
|
{
|
|
int ret = 0;
|
|
|
|
if (atomic_dec_return(&sem->count) < 0)
|
|
ret = __down_trylock(sem);
|
|
return ret;
|
|
}
|
|
|
|
/*
|
|
* Note! This is subtle. We jump to wake people up only if
|
|
* the semaphore was negative (== somebody was waiting on it).
|
|
*/
|
|
static inline void up(struct semaphore * sem)
|
|
{
|
|
if (atomic_inc_return(&sem->count) <= 0)
|
|
__up(sem);
|
|
}
|
|
|
|
#endif
|
|
#endif /* __ASM_SH_SEMAPHORE_H */
|