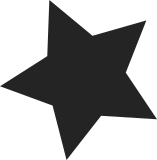
Wire up the existing arm64 support for SMBIOS tables (aka DMI) for ARM as well, by moving the arm64 init code to drivers/firmware/efi/arm-runtime.c (which is shared between ARM and arm64), and adding a asm/dmi.h header to ARM that defines the mapping routines for the firmware tables. This allows userspace to access these tables to discover system information exposed by the firmware. It also sets the hardware name used in crash dumps, e.g.: Unable to handle kernel NULL pointer dereference at virtual address 00000000 pgd = ed3c0000 [00000000] *pgd=bf1f3835 Internal error: Oops: 817 [#1] SMP THUMB2 Modules linked in: CPU: 0 PID: 759 Comm: bash Not tainted 4.10.0-09601-g0e8f38792120-dirty #112 Hardware name: QEMU KVM Virtual Machine, BIOS 0.0.0 02/06/2015 ^^^ NOTE: This does *NOT* enable or encourage the use of DMI quirks, i.e., the the practice of identifying the platform via DMI to decide whether certain workarounds for buggy hardware and/or firmware need to be enabled. This would require the DMI subsystem to be enabled much earlier than we do on ARM, which is non-trivial. Signed-off-by: Ard Biesheuvel <ard.biesheuvel@linaro.org> Acked-by: Russell King <rmk+kernel@armlinux.org.uk> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Matt Fleming <matt@codeblueprint.co.uk> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: linux-efi@vger.kernel.org Link: http://lkml.kernel.org/r/20170602135207.21708-14-ard.biesheuvel@linaro.org Signed-off-by: Ingo Molnar <mingo@kernel.org>
185 lines
4.3 KiB
C
185 lines
4.3 KiB
C
/*
|
|
* Extensible Firmware Interface
|
|
*
|
|
* Based on Extensible Firmware Interface Specification version 2.4
|
|
*
|
|
* Copyright (C) 2013, 2014 Linaro Ltd.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
*/
|
|
|
|
#include <linux/dmi.h>
|
|
#include <linux/efi.h>
|
|
#include <linux/io.h>
|
|
#include <linux/memblock.h>
|
|
#include <linux/mm_types.h>
|
|
#include <linux/preempt.h>
|
|
#include <linux/rbtree.h>
|
|
#include <linux/rwsem.h>
|
|
#include <linux/sched.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/spinlock.h>
|
|
|
|
#include <asm/cacheflush.h>
|
|
#include <asm/efi.h>
|
|
#include <asm/mmu.h>
|
|
#include <asm/pgalloc.h>
|
|
#include <asm/pgtable.h>
|
|
|
|
extern u64 efi_system_table;
|
|
|
|
static struct mm_struct efi_mm = {
|
|
.mm_rb = RB_ROOT,
|
|
.mm_users = ATOMIC_INIT(2),
|
|
.mm_count = ATOMIC_INIT(1),
|
|
.mmap_sem = __RWSEM_INITIALIZER(efi_mm.mmap_sem),
|
|
.page_table_lock = __SPIN_LOCK_UNLOCKED(efi_mm.page_table_lock),
|
|
.mmlist = LIST_HEAD_INIT(efi_mm.mmlist),
|
|
};
|
|
|
|
#ifdef CONFIG_ARM64_PTDUMP_DEBUGFS
|
|
#include <asm/ptdump.h>
|
|
|
|
static struct ptdump_info efi_ptdump_info = {
|
|
.mm = &efi_mm,
|
|
.markers = (struct addr_marker[]){
|
|
{ 0, "UEFI runtime start" },
|
|
{ TASK_SIZE_64, "UEFI runtime end" }
|
|
},
|
|
.base_addr = 0,
|
|
};
|
|
|
|
static int __init ptdump_init(void)
|
|
{
|
|
return ptdump_debugfs_register(&efi_ptdump_info, "efi_page_tables");
|
|
}
|
|
device_initcall(ptdump_init);
|
|
|
|
#endif
|
|
|
|
static bool __init efi_virtmap_init(void)
|
|
{
|
|
efi_memory_desc_t *md;
|
|
bool systab_found;
|
|
|
|
efi_mm.pgd = pgd_alloc(&efi_mm);
|
|
mm_init_cpumask(&efi_mm);
|
|
init_new_context(NULL, &efi_mm);
|
|
|
|
systab_found = false;
|
|
for_each_efi_memory_desc(md) {
|
|
phys_addr_t phys = md->phys_addr;
|
|
int ret;
|
|
|
|
if (!(md->attribute & EFI_MEMORY_RUNTIME))
|
|
continue;
|
|
if (md->virt_addr == 0)
|
|
return false;
|
|
|
|
ret = efi_create_mapping(&efi_mm, md);
|
|
if (!ret) {
|
|
pr_info(" EFI remap %pa => %p\n",
|
|
&phys, (void *)(unsigned long)md->virt_addr);
|
|
} else {
|
|
pr_warn(" EFI remap %pa: failed to create mapping (%d)\n",
|
|
&phys, ret);
|
|
return false;
|
|
}
|
|
/*
|
|
* If this entry covers the address of the UEFI system table,
|
|
* calculate and record its virtual address.
|
|
*/
|
|
if (efi_system_table >= phys &&
|
|
efi_system_table < phys + (md->num_pages * EFI_PAGE_SIZE)) {
|
|
efi.systab = (void *)(unsigned long)(efi_system_table -
|
|
phys + md->virt_addr);
|
|
systab_found = true;
|
|
}
|
|
}
|
|
if (!systab_found) {
|
|
pr_err("No virtual mapping found for the UEFI System Table\n");
|
|
return false;
|
|
}
|
|
|
|
if (efi_memattr_apply_permissions(&efi_mm, efi_set_mapping_permissions))
|
|
return false;
|
|
|
|
return true;
|
|
}
|
|
|
|
/*
|
|
* Enable the UEFI Runtime Services if all prerequisites are in place, i.e.,
|
|
* non-early mapping of the UEFI system table and virtual mappings for all
|
|
* EFI_MEMORY_RUNTIME regions.
|
|
*/
|
|
static int __init arm_enable_runtime_services(void)
|
|
{
|
|
u64 mapsize;
|
|
|
|
if (!efi_enabled(EFI_BOOT)) {
|
|
pr_info("EFI services will not be available.\n");
|
|
return 0;
|
|
}
|
|
|
|
if (efi_runtime_disabled()) {
|
|
pr_info("EFI runtime services will be disabled.\n");
|
|
return 0;
|
|
}
|
|
|
|
if (efi_enabled(EFI_RUNTIME_SERVICES)) {
|
|
pr_info("EFI runtime services access via paravirt.\n");
|
|
return 0;
|
|
}
|
|
|
|
pr_info("Remapping and enabling EFI services.\n");
|
|
|
|
mapsize = efi.memmap.desc_size * efi.memmap.nr_map;
|
|
|
|
if (efi_memmap_init_late(efi.memmap.phys_map, mapsize)) {
|
|
pr_err("Failed to remap EFI memory map\n");
|
|
return -ENOMEM;
|
|
}
|
|
|
|
if (!efi_virtmap_init()) {
|
|
pr_err("UEFI virtual mapping missing or invalid -- runtime services will not be available\n");
|
|
return -ENOMEM;
|
|
}
|
|
|
|
/* Set up runtime services function pointers */
|
|
efi_native_runtime_setup();
|
|
set_bit(EFI_RUNTIME_SERVICES, &efi.flags);
|
|
|
|
return 0;
|
|
}
|
|
early_initcall(arm_enable_runtime_services);
|
|
|
|
void efi_virtmap_load(void)
|
|
{
|
|
preempt_disable();
|
|
efi_set_pgd(&efi_mm);
|
|
}
|
|
|
|
void efi_virtmap_unload(void)
|
|
{
|
|
efi_set_pgd(current->active_mm);
|
|
preempt_enable();
|
|
}
|
|
|
|
|
|
static int __init arm_dmi_init(void)
|
|
{
|
|
/*
|
|
* On arm64/ARM, DMI depends on UEFI, and dmi_scan_machine() needs to
|
|
* be called early because dmi_id_init(), which is an arch_initcall
|
|
* itself, depends on dmi_scan_machine() having been called already.
|
|
*/
|
|
dmi_scan_machine();
|
|
if (dmi_available)
|
|
dmi_set_dump_stack_arch_desc();
|
|
return 0;
|
|
}
|
|
core_initcall(arm_dmi_init);
|