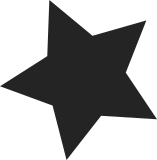
Impact: build fix for Sparc and s390
Stephen Rothwell reported that the Sparc build broke:
In file included from kernel/panic.c:12:
include/linux/debug_locks.h: In function '__debug_locks_off':
include/linux/debug_locks.h:15: error: implicit declaration of function 'xchg'
due to:
9eeba61
: lockdep: warn about lockdep disabling after kernel taint
There is some inconsistency between architectures about where exactly
xchg() is defined.
The traditional place is in system.h but the more logical point for it
is in atomic.h - where most architectures (especially new ones) have
it defined. These architecture also still offer it via system.h.
Some, such as Sparc or s390 only have it in asm/system.h and not available
via asm/atomic.h at all.
Use the widest set of headers in debug_locks.h and also include asm/system.h.
Reported-by: Stephen Rothwell <sfr@canb.auug.org.au>
Cc: Frederic Weisbecker <fweisbec@gmail.com>
Cc: "David S. Miller" <davem@davemloft.net>
Cc: Linus Torvalds <torvalds@linux-foundation.org>
LKML-Reference: <20090414144317.026498df.sfr@canb.auug.org.au>
Signed-off-by: Ingo Molnar <mingo@elte.hu>
81 lines
1.6 KiB
C
81 lines
1.6 KiB
C
#ifndef __LINUX_DEBUG_LOCKING_H
|
|
#define __LINUX_DEBUG_LOCKING_H
|
|
|
|
#include <linux/kernel.h>
|
|
#include <asm/atomic.h>
|
|
#include <asm/system.h>
|
|
|
|
struct task_struct;
|
|
|
|
extern int debug_locks;
|
|
extern int debug_locks_silent;
|
|
|
|
|
|
static inline int __debug_locks_off(void)
|
|
{
|
|
return xchg(&debug_locks, 0);
|
|
}
|
|
|
|
/*
|
|
* Generic 'turn off all lock debugging' function:
|
|
*/
|
|
extern int debug_locks_off(void);
|
|
|
|
#define DEBUG_LOCKS_WARN_ON(c) \
|
|
({ \
|
|
int __ret = 0; \
|
|
\
|
|
if (!oops_in_progress && unlikely(c)) { \
|
|
if (debug_locks_off() && !debug_locks_silent) \
|
|
WARN_ON(1); \
|
|
__ret = 1; \
|
|
} \
|
|
__ret; \
|
|
})
|
|
|
|
#ifdef CONFIG_SMP
|
|
# define SMP_DEBUG_LOCKS_WARN_ON(c) DEBUG_LOCKS_WARN_ON(c)
|
|
#else
|
|
# define SMP_DEBUG_LOCKS_WARN_ON(c) do { } while (0)
|
|
#endif
|
|
|
|
#ifdef CONFIG_DEBUG_LOCKING_API_SELFTESTS
|
|
extern void locking_selftest(void);
|
|
#else
|
|
# define locking_selftest() do { } while (0)
|
|
#endif
|
|
|
|
struct task_struct;
|
|
|
|
#ifdef CONFIG_LOCKDEP
|
|
extern void debug_show_all_locks(void);
|
|
extern void __debug_show_held_locks(struct task_struct *task);
|
|
extern void debug_show_held_locks(struct task_struct *task);
|
|
extern void debug_check_no_locks_freed(const void *from, unsigned long len);
|
|
extern void debug_check_no_locks_held(struct task_struct *task);
|
|
#else
|
|
static inline void debug_show_all_locks(void)
|
|
{
|
|
}
|
|
|
|
static inline void __debug_show_held_locks(struct task_struct *task)
|
|
{
|
|
}
|
|
|
|
static inline void debug_show_held_locks(struct task_struct *task)
|
|
{
|
|
}
|
|
|
|
static inline void
|
|
debug_check_no_locks_freed(const void *from, unsigned long len)
|
|
{
|
|
}
|
|
|
|
static inline void
|
|
debug_check_no_locks_held(struct task_struct *task)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
#endif
|