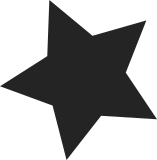
This patch moves the definition of struct rnd_state and the inline __seed() function to linux/random.h. It renames the static __random32() function to prandom32() and exports it for use in modules. prandom32() is useful as a privately-seeded pseudo random number generator that can give the same result every time it is initialized. For FCoE FC-BB-6 VN2VN mode self-selected unique FC address generation, we need an pseudo-random number generator seeded with the 64-bit world-wide port name. A truly random generator or one seeded with randomness won't do because the same sequence of numbers should be generated each time we boot or the link comes up. A prandom32_seed() inline function is added to the header file. It is inlined not for speed, but so the function won't be expanded in the base kernel, but only in the module that uses it. Signed-off-by: Joe Eykholt <jeykholt@cisco.com> Acked-by: Matt Mackall <mpm@selenic.com> Cc: Theodore Ts'o <tytso@mit.edu> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
108 lines
2.7 KiB
C
108 lines
2.7 KiB
C
/*
|
|
* include/linux/random.h
|
|
*
|
|
* Include file for the random number generator.
|
|
*/
|
|
|
|
#ifndef _LINUX_RANDOM_H
|
|
#define _LINUX_RANDOM_H
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/ioctl.h>
|
|
#include <linux/irqnr.h>
|
|
|
|
/* ioctl()'s for the random number generator */
|
|
|
|
/* Get the entropy count. */
|
|
#define RNDGETENTCNT _IOR( 'R', 0x00, int )
|
|
|
|
/* Add to (or subtract from) the entropy count. (Superuser only.) */
|
|
#define RNDADDTOENTCNT _IOW( 'R', 0x01, int )
|
|
|
|
/* Get the contents of the entropy pool. (Superuser only.) */
|
|
#define RNDGETPOOL _IOR( 'R', 0x02, int [2] )
|
|
|
|
/*
|
|
* Write bytes into the entropy pool and add to the entropy count.
|
|
* (Superuser only.)
|
|
*/
|
|
#define RNDADDENTROPY _IOW( 'R', 0x03, int [2] )
|
|
|
|
/* Clear entropy count to 0. (Superuser only.) */
|
|
#define RNDZAPENTCNT _IO( 'R', 0x04 )
|
|
|
|
/* Clear the entropy pool and associated counters. (Superuser only.) */
|
|
#define RNDCLEARPOOL _IO( 'R', 0x06 )
|
|
|
|
struct rand_pool_info {
|
|
int entropy_count;
|
|
int buf_size;
|
|
__u32 buf[0];
|
|
};
|
|
|
|
struct rnd_state {
|
|
__u32 s1, s2, s3;
|
|
};
|
|
|
|
/* Exported functions */
|
|
|
|
#ifdef __KERNEL__
|
|
|
|
extern void rand_initialize_irq(int irq);
|
|
|
|
extern void add_input_randomness(unsigned int type, unsigned int code,
|
|
unsigned int value);
|
|
extern void add_interrupt_randomness(int irq);
|
|
|
|
extern void get_random_bytes(void *buf, int nbytes);
|
|
void generate_random_uuid(unsigned char uuid_out[16]);
|
|
|
|
extern __u32 secure_ip_id(__be32 daddr);
|
|
extern u32 secure_ipv4_port_ephemeral(__be32 saddr, __be32 daddr, __be16 dport);
|
|
extern u32 secure_ipv6_port_ephemeral(const __be32 *saddr, const __be32 *daddr,
|
|
__be16 dport);
|
|
extern __u32 secure_tcp_sequence_number(__be32 saddr, __be32 daddr,
|
|
__be16 sport, __be16 dport);
|
|
extern __u32 secure_tcpv6_sequence_number(__be32 *saddr, __be32 *daddr,
|
|
__be16 sport, __be16 dport);
|
|
extern u64 secure_dccp_sequence_number(__be32 saddr, __be32 daddr,
|
|
__be16 sport, __be16 dport);
|
|
|
|
#ifndef MODULE
|
|
extern const struct file_operations random_fops, urandom_fops;
|
|
#endif
|
|
|
|
unsigned int get_random_int(void);
|
|
unsigned long randomize_range(unsigned long start, unsigned long end, unsigned long len);
|
|
|
|
u32 random32(void);
|
|
void srandom32(u32 seed);
|
|
|
|
u32 prandom32(struct rnd_state *);
|
|
|
|
/*
|
|
* Handle minimum values for seeds
|
|
*/
|
|
static inline u32 __seed(u32 x, u32 m)
|
|
{
|
|
return (x < m) ? x + m : x;
|
|
}
|
|
|
|
/**
|
|
* prandom32_seed - set seed for prandom32().
|
|
* @state: pointer to state structure to receive the seed.
|
|
* @seed: arbitrary 64-bit value to use as a seed.
|
|
*/
|
|
static inline void prandom32_seed(struct rnd_state *state, u64 seed)
|
|
{
|
|
u32 i = (seed >> 32) ^ (seed << 10) ^ seed;
|
|
|
|
state->s1 = __seed(i, 1);
|
|
state->s2 = __seed(i, 7);
|
|
state->s3 = __seed(i, 15);
|
|
}
|
|
|
|
#endif /* __KERNEL___ */
|
|
|
|
#endif /* _LINUX_RANDOM_H */
|