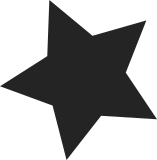
Overlapping overlay layers are not supported and can cause unexpected behavior, but overlayfs does not currently check or warn about these configurations. User is not supposed to specify the same directory for upper and lower dirs or for different lower layers and user is not supposed to specify directories that are descendants of each other for overlay layers, but that is exactly what this zysbot repro did: https://syzkaller.appspot.com/x/repro.syz?x=12c7a94f400000 Moving layer root directories into other layers while overlayfs is mounted could also result in unexpected behavior. This commit places "traps" in the overlay inode hash table. Those traps are dummy overlay inodes that are hashed by the layers root inodes. On mount, the hash table trap entries are used to verify that overlay layers are not overlapping. While at it, we also verify that overlay layers are not overlapping with directories "in-use" by other overlay instances as upperdir/workdir. On lookup, the trap entries are used to verify that overlay layers root inodes have not been moved into other layers after mount. Some examples: $ ./run --ov --samefs -s ... ( mkdir -p base/upper/0/u base/upper/0/w base/lower lower upper mnt mount -o bind base/lower lower mount -o bind base/upper upper mount -t overlay none mnt ... -o lowerdir=lower,upperdir=upper/0/u,workdir=upper/0/w) $ umount mnt $ mount -t overlay none mnt ... -o lowerdir=base,upperdir=upper/0/u,workdir=upper/0/w [ 94.434900] overlayfs: overlapping upperdir path mount: mount overlay on mnt failed: Too many levels of symbolic links $ mount -t overlay none mnt ... -o lowerdir=upper/0/u,upperdir=upper/0/u,workdir=upper/0/w [ 151.350132] overlayfs: conflicting lowerdir path mount: none is already mounted or mnt busy $ mount -t overlay none mnt ... -o lowerdir=lower:lower/a,upperdir=upper/0/u,workdir=upper/0/w [ 201.205045] overlayfs: overlapping lowerdir path mount: mount overlay on mnt failed: Too many levels of symbolic links $ mount -t overlay none mnt ... -o lowerdir=lower,upperdir=upper/0/u,workdir=upper/0/w $ mv base/upper/0/ base/lower/ $ find mnt/0 mnt/0 mnt/0/w find: 'mnt/0/w/work': Too many levels of symbolic links find: 'mnt/0/u': Too many levels of symbolic links Reported-by: syzbot+9c69c282adc4edd2b540@syzkaller.appspotmail.com Signed-off-by: Amir Goldstein <amir73il@gmail.com> Signed-off-by: Miklos Szeredi <mszeredi@redhat.com>
122 lines
2.8 KiB
C
122 lines
2.8 KiB
C
/*
|
|
*
|
|
* Copyright (C) 2011 Novell Inc.
|
|
* Copyright (C) 2016 Red Hat, Inc.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms of the GNU General Public License version 2 as published by
|
|
* the Free Software Foundation.
|
|
*/
|
|
|
|
struct ovl_config {
|
|
char *lowerdir;
|
|
char *upperdir;
|
|
char *workdir;
|
|
bool default_permissions;
|
|
bool redirect_dir;
|
|
bool redirect_follow;
|
|
const char *redirect_mode;
|
|
bool index;
|
|
bool nfs_export;
|
|
int xino;
|
|
bool metacopy;
|
|
};
|
|
|
|
struct ovl_sb {
|
|
struct super_block *sb;
|
|
dev_t pseudo_dev;
|
|
};
|
|
|
|
struct ovl_layer {
|
|
struct vfsmount *mnt;
|
|
/* Trap in ovl inode cache */
|
|
struct inode *trap;
|
|
struct ovl_sb *fs;
|
|
/* Index of this layer in fs root (upper idx == 0) */
|
|
int idx;
|
|
/* One fsid per unique underlying sb (upper fsid == 0) */
|
|
int fsid;
|
|
};
|
|
|
|
struct ovl_path {
|
|
struct ovl_layer *layer;
|
|
struct dentry *dentry;
|
|
};
|
|
|
|
/* private information held for overlayfs's superblock */
|
|
struct ovl_fs {
|
|
struct vfsmount *upper_mnt;
|
|
unsigned int numlower;
|
|
/* Number of unique lower sb that differ from upper sb */
|
|
unsigned int numlowerfs;
|
|
struct ovl_layer *lower_layers;
|
|
struct ovl_sb *lower_fs;
|
|
/* workbasedir is the path at workdir= mount option */
|
|
struct dentry *workbasedir;
|
|
/* workdir is the 'work' directory under workbasedir */
|
|
struct dentry *workdir;
|
|
/* index directory listing overlay inodes by origin file handle */
|
|
struct dentry *indexdir;
|
|
long namelen;
|
|
/* pathnames of lower and upper dirs, for show_options */
|
|
struct ovl_config config;
|
|
/* creds of process who forced instantiation of super block */
|
|
const struct cred *creator_cred;
|
|
bool tmpfile;
|
|
bool noxattr;
|
|
/* Did we take the inuse lock? */
|
|
bool upperdir_locked;
|
|
bool workdir_locked;
|
|
/* Traps in ovl inode cache */
|
|
struct inode *upperdir_trap;
|
|
struct inode *workdir_trap;
|
|
struct inode *indexdir_trap;
|
|
/* Inode numbers in all layers do not use the high xino_bits */
|
|
unsigned int xino_bits;
|
|
};
|
|
|
|
/* private information held for every overlayfs dentry */
|
|
struct ovl_entry {
|
|
union {
|
|
struct {
|
|
unsigned long flags;
|
|
};
|
|
struct rcu_head rcu;
|
|
};
|
|
unsigned numlower;
|
|
struct ovl_path lowerstack[];
|
|
};
|
|
|
|
struct ovl_entry *ovl_alloc_entry(unsigned int numlower);
|
|
|
|
static inline struct ovl_entry *OVL_E(struct dentry *dentry)
|
|
{
|
|
return (struct ovl_entry *) dentry->d_fsdata;
|
|
}
|
|
|
|
struct ovl_inode {
|
|
union {
|
|
struct ovl_dir_cache *cache; /* directory */
|
|
struct inode *lowerdata; /* regular file */
|
|
};
|
|
const char *redirect;
|
|
u64 version;
|
|
unsigned long flags;
|
|
struct inode vfs_inode;
|
|
struct dentry *__upperdentry;
|
|
struct inode *lower;
|
|
|
|
/* synchronize copy up and more */
|
|
struct mutex lock;
|
|
};
|
|
|
|
static inline struct ovl_inode *OVL_I(struct inode *inode)
|
|
{
|
|
return container_of(inode, struct ovl_inode, vfs_inode);
|
|
}
|
|
|
|
static inline struct dentry *ovl_upperdentry_dereference(struct ovl_inode *oi)
|
|
{
|
|
return READ_ONCE(oi->__upperdentry);
|
|
}
|