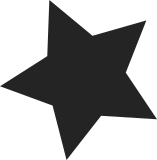
This patch benefits from newly introduced switchdev notifier and uses it to propagate fdb learn events from rocker driver to bridge. That avoids direct function calls and possible use by other listeners (ovs). Suggested-by: Thomas Graf <tgraf@suug.ch> Signed-off-by: Jiri Pirko <jiri@resnulli.us> Signed-off-by: Scott Feldman <sfeldma@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
77 lines
1.9 KiB
C
77 lines
1.9 KiB
C
/*
|
|
* Linux ethernet bridge
|
|
*
|
|
* Authors:
|
|
* Lennert Buytenhek <buytenh@gnu.org>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
#ifndef _LINUX_IF_BRIDGE_H
|
|
#define _LINUX_IF_BRIDGE_H
|
|
|
|
|
|
#include <linux/netdevice.h>
|
|
#include <uapi/linux/if_bridge.h>
|
|
#include <linux/bitops.h>
|
|
|
|
struct br_ip {
|
|
union {
|
|
__be32 ip4;
|
|
#if IS_ENABLED(CONFIG_IPV6)
|
|
struct in6_addr ip6;
|
|
#endif
|
|
} u;
|
|
__be16 proto;
|
|
__u16 vid;
|
|
};
|
|
|
|
struct br_ip_list {
|
|
struct list_head list;
|
|
struct br_ip addr;
|
|
};
|
|
|
|
#define BR_HAIRPIN_MODE BIT(0)
|
|
#define BR_BPDU_GUARD BIT(1)
|
|
#define BR_ROOT_BLOCK BIT(2)
|
|
#define BR_MULTICAST_FAST_LEAVE BIT(3)
|
|
#define BR_ADMIN_COST BIT(4)
|
|
#define BR_LEARNING BIT(5)
|
|
#define BR_FLOOD BIT(6)
|
|
#define BR_AUTO_MASK (BR_FLOOD | BR_LEARNING)
|
|
#define BR_PROMISC BIT(7)
|
|
#define BR_PROXYARP BIT(8)
|
|
#define BR_LEARNING_SYNC BIT(9)
|
|
|
|
extern void brioctl_set(int (*ioctl_hook)(struct net *, unsigned int, void __user *));
|
|
|
|
typedef int br_should_route_hook_t(struct sk_buff *skb);
|
|
extern br_should_route_hook_t __rcu *br_should_route_hook;
|
|
|
|
#if IS_ENABLED(CONFIG_BRIDGE) && IS_ENABLED(CONFIG_BRIDGE_IGMP_SNOOPING)
|
|
int br_multicast_list_adjacent(struct net_device *dev,
|
|
struct list_head *br_ip_list);
|
|
bool br_multicast_has_querier_anywhere(struct net_device *dev, int proto);
|
|
bool br_multicast_has_querier_adjacent(struct net_device *dev, int proto);
|
|
#else
|
|
static inline int br_multicast_list_adjacent(struct net_device *dev,
|
|
struct list_head *br_ip_list)
|
|
{
|
|
return 0;
|
|
}
|
|
static inline bool br_multicast_has_querier_anywhere(struct net_device *dev,
|
|
int proto)
|
|
{
|
|
return false;
|
|
}
|
|
static inline bool br_multicast_has_querier_adjacent(struct net_device *dev,
|
|
int proto)
|
|
{
|
|
return false;
|
|
}
|
|
#endif
|
|
|
|
#endif
|