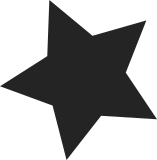
The best place to register the CPU cooling device is from the cpufreq driver as we would know if all the resources are already available or not. That's what is done for the cpufreq-dt.c driver as well. The cpu-cooling driver for dbx500 platform was just (un)registering with the thermal framework and that can be handled easily by the cpufreq driver as well and in proper sequence as well. Get rid of the cooling driver and its its users and manage everything from the cpufreq driver instead. Signed-off-by: Viresh Kumar <viresh.kumar@linaro.org> Tested-by: Linus Walleij <linus.walleij@linaro.org> Signed-off-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com>
104 lines
2.7 KiB
C
104 lines
2.7 KiB
C
/*
|
|
* Copyright (C) STMicroelectronics 2009
|
|
* Copyright (C) ST-Ericsson SA 2010-2012
|
|
*
|
|
* License Terms: GNU General Public License v2
|
|
* Author: Sundar Iyer <sundar.iyer@stericsson.com>
|
|
* Author: Martin Persson <martin.persson@stericsson.com>
|
|
* Author: Jonas Aaberg <jonas.aberg@stericsson.com>
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/cpufreq.h>
|
|
#include <linux/cpu_cooling.h>
|
|
#include <linux/delay.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/platform_device.h>
|
|
#include <linux/clk.h>
|
|
|
|
static struct cpufreq_frequency_table *freq_table;
|
|
static struct clk *armss_clk;
|
|
static struct thermal_cooling_device *cdev;
|
|
|
|
static int dbx500_cpufreq_target(struct cpufreq_policy *policy,
|
|
unsigned int index)
|
|
{
|
|
/* update armss clk frequency */
|
|
return clk_set_rate(armss_clk, freq_table[index].frequency * 1000);
|
|
}
|
|
|
|
static int dbx500_cpufreq_init(struct cpufreq_policy *policy)
|
|
{
|
|
policy->clk = armss_clk;
|
|
return cpufreq_generic_init(policy, freq_table, 20 * 1000);
|
|
}
|
|
|
|
static int dbx500_cpufreq_exit(struct cpufreq_policy *policy)
|
|
{
|
|
if (!IS_ERR(cdev))
|
|
cpufreq_cooling_unregister(cdev);
|
|
return 0;
|
|
}
|
|
|
|
static void dbx500_cpufreq_ready(struct cpufreq_policy *policy)
|
|
{
|
|
cdev = cpufreq_cooling_register(policy->cpus);
|
|
if (IS_ERR(cdev))
|
|
pr_err("Failed to register cooling device %ld\n", PTR_ERR(cdev));
|
|
else
|
|
pr_info("Cooling device registered: %s\n", cdev->type);
|
|
}
|
|
|
|
static struct cpufreq_driver dbx500_cpufreq_driver = {
|
|
.flags = CPUFREQ_STICKY | CPUFREQ_CONST_LOOPS |
|
|
CPUFREQ_NEED_INITIAL_FREQ_CHECK,
|
|
.verify = cpufreq_generic_frequency_table_verify,
|
|
.target_index = dbx500_cpufreq_target,
|
|
.get = cpufreq_generic_get,
|
|
.init = dbx500_cpufreq_init,
|
|
.exit = dbx500_cpufreq_exit,
|
|
.ready = dbx500_cpufreq_ready,
|
|
.name = "DBX500",
|
|
.attr = cpufreq_generic_attr,
|
|
};
|
|
|
|
static int dbx500_cpufreq_probe(struct platform_device *pdev)
|
|
{
|
|
struct cpufreq_frequency_table *pos;
|
|
|
|
freq_table = dev_get_platdata(&pdev->dev);
|
|
if (!freq_table) {
|
|
pr_err("dbx500-cpufreq: Failed to fetch cpufreq table\n");
|
|
return -ENODEV;
|
|
}
|
|
|
|
armss_clk = clk_get(&pdev->dev, "armss");
|
|
if (IS_ERR(armss_clk)) {
|
|
pr_err("dbx500-cpufreq: Failed to get armss clk\n");
|
|
return PTR_ERR(armss_clk);
|
|
}
|
|
|
|
pr_info("dbx500-cpufreq: Available frequencies:\n");
|
|
cpufreq_for_each_entry(pos, freq_table)
|
|
pr_info(" %d Mhz\n", pos->frequency / 1000);
|
|
|
|
return cpufreq_register_driver(&dbx500_cpufreq_driver);
|
|
}
|
|
|
|
static struct platform_driver dbx500_cpufreq_plat_driver = {
|
|
.driver = {
|
|
.name = "cpufreq-ux500",
|
|
},
|
|
.probe = dbx500_cpufreq_probe,
|
|
};
|
|
|
|
static int __init dbx500_cpufreq_register(void)
|
|
{
|
|
return platform_driver_register(&dbx500_cpufreq_plat_driver);
|
|
}
|
|
device_initcall(dbx500_cpufreq_register);
|
|
|
|
MODULE_LICENSE("GPL v2");
|
|
MODULE_DESCRIPTION("cpufreq driver for DBX500");
|