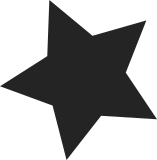
To define the outgoing port and to discover the incoming port a regular VLAN tag is used by the LAN9303. But its VID meaning is 'special'. This tag handler/filter depends on some hardware features which must be enabled in the device to provide and make use of this special VLAN tag to control the destination and the source of an ethernet packet. Signed-off-by: Juergen Borleis <jbe@pengutronix.de> Reviewed-by: Florian Fainelli <f.fainelli@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
100 lines
2.8 KiB
C
100 lines
2.8 KiB
C
/*
|
|
* net/dsa/dsa_priv.h - Hardware switch handling
|
|
* Copyright (c) 2008-2009 Marvell Semiconductor
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*/
|
|
|
|
#ifndef __DSA_PRIV_H
|
|
#define __DSA_PRIV_H
|
|
|
|
#include <linux/phy.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/netpoll.h>
|
|
|
|
struct dsa_device_ops {
|
|
struct sk_buff *(*xmit)(struct sk_buff *skb, struct net_device *dev);
|
|
struct sk_buff *(*rcv)(struct sk_buff *skb, struct net_device *dev,
|
|
struct packet_type *pt,
|
|
struct net_device *orig_dev);
|
|
};
|
|
|
|
struct dsa_slave_priv {
|
|
struct sk_buff * (*xmit)(struct sk_buff *skb,
|
|
struct net_device *dev);
|
|
|
|
/* DSA port data, such as switch, port index, etc. */
|
|
struct dsa_port *dp;
|
|
|
|
/*
|
|
* The phylib phy_device pointer for the PHY connected
|
|
* to this port.
|
|
*/
|
|
struct phy_device *phy;
|
|
phy_interface_t phy_interface;
|
|
int old_link;
|
|
int old_pause;
|
|
int old_duplex;
|
|
|
|
#ifdef CONFIG_NET_POLL_CONTROLLER
|
|
struct netpoll *netpoll;
|
|
#endif
|
|
|
|
/* TC context */
|
|
struct list_head mall_tc_list;
|
|
};
|
|
|
|
/* dsa.c */
|
|
int dsa_cpu_dsa_setup(struct dsa_switch *ds, struct device *dev,
|
|
struct dsa_port *dport, int port);
|
|
void dsa_cpu_dsa_destroy(struct dsa_port *dport);
|
|
const struct dsa_device_ops *dsa_resolve_tag_protocol(int tag_protocol);
|
|
int dsa_cpu_port_ethtool_setup(struct dsa_switch *ds);
|
|
void dsa_cpu_port_ethtool_restore(struct dsa_switch *ds);
|
|
|
|
/* legacy.c */
|
|
int dsa_legacy_register(void);
|
|
void dsa_legacy_unregister(void);
|
|
|
|
/* slave.c */
|
|
extern const struct dsa_device_ops notag_netdev_ops;
|
|
void dsa_slave_mii_bus_init(struct dsa_switch *ds);
|
|
void dsa_cpu_port_ethtool_init(struct ethtool_ops *ops);
|
|
int dsa_slave_create(struct dsa_switch *ds, struct device *parent,
|
|
int port, const char *name);
|
|
void dsa_slave_destroy(struct net_device *slave_dev);
|
|
int dsa_slave_suspend(struct net_device *slave_dev);
|
|
int dsa_slave_resume(struct net_device *slave_dev);
|
|
int dsa_slave_register_notifier(void);
|
|
void dsa_slave_unregister_notifier(void);
|
|
|
|
/* switch.c */
|
|
int dsa_switch_register_notifier(struct dsa_switch *ds);
|
|
void dsa_switch_unregister_notifier(struct dsa_switch *ds);
|
|
|
|
/* tag_dsa.c */
|
|
extern const struct dsa_device_ops dsa_netdev_ops;
|
|
|
|
/* tag_edsa.c */
|
|
extern const struct dsa_device_ops edsa_netdev_ops;
|
|
|
|
/* tag_trailer.c */
|
|
extern const struct dsa_device_ops trailer_netdev_ops;
|
|
|
|
/* tag_brcm.c */
|
|
extern const struct dsa_device_ops brcm_netdev_ops;
|
|
|
|
/* tag_qca.c */
|
|
extern const struct dsa_device_ops qca_netdev_ops;
|
|
|
|
/* tag_mtk.c */
|
|
extern const struct dsa_device_ops mtk_netdev_ops;
|
|
|
|
/* tag_lan9303.c */
|
|
extern const struct dsa_device_ops lan9303_netdev_ops;
|
|
|
|
#endif
|