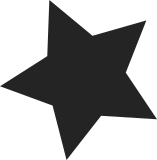
When events occurs while no one is listening to the node (hid->open == 0 and usb_kill_urb() called) some events are still stacked somewhere in the USB (kernel or device?) stack. When the node gets reopened, these events are drained, and this results in spurious touch down/up, or mouse button clicks. The problem was spotted with touchscreens in fdo bug #81781 [1], but it actually occurs with any mouse using hid-generic or touchscreen. A way to reproduce it is to call: $ xinput disable 9 ; sleep 5 ; xinput enable 9 With 9 being the device ID for the touchscreen/mouse. During the "sleep", produce some touch events or click events. When "xinput enable" is called, at least one click is generated. This patch tries to fix this by draining the queue for 50 msec and during this time frame, not forwarding these old events to the hid layer. Hans completed the explanation: """ Devices like mice (basically any hid device) will have a fifo on the device side, when we stop submitting urbs to get hid reports from it, that fifo will fill up, and when we resume we will get whatever is there in that fifo. """ [1] https://bugs.freedesktop.org/show_bug.cgi?id=81781 Signed-off-by: Benjamin Tissoires <benjamin.tissoires@redhat.com> Signed-off-by: Jiri Kosina <jkosina@suse.cz>
103 lines
4.1 KiB
C
103 lines
4.1 KiB
C
#ifndef __USBHID_H
|
|
#define __USBHID_H
|
|
|
|
/*
|
|
* Copyright (c) 1999 Andreas Gal
|
|
* Copyright (c) 2000-2001 Vojtech Pavlik
|
|
* Copyright (c) 2006 Jiri Kosina
|
|
*/
|
|
|
|
/*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/list.h>
|
|
#include <linux/mutex.h>
|
|
#include <linux/timer.h>
|
|
#include <linux/wait.h>
|
|
#include <linux/workqueue.h>
|
|
#include <linux/input.h>
|
|
|
|
/* API provided by hid-core.c for USB HID drivers */
|
|
void usbhid_close(struct hid_device *hid);
|
|
int usbhid_open(struct hid_device *hid);
|
|
void usbhid_init_reports(struct hid_device *hid);
|
|
int usbhid_get_power(struct hid_device *hid);
|
|
void usbhid_put_power(struct hid_device *hid);
|
|
struct usb_interface *usbhid_find_interface(int minor);
|
|
|
|
/* iofl flags */
|
|
#define HID_CTRL_RUNNING 1
|
|
#define HID_OUT_RUNNING 2
|
|
#define HID_IN_RUNNING 3
|
|
#define HID_RESET_PENDING 4
|
|
#define HID_SUSPENDED 5
|
|
#define HID_CLEAR_HALT 6
|
|
#define HID_DISCONNECTED 7
|
|
#define HID_STARTED 8
|
|
#define HID_KEYS_PRESSED 10
|
|
#define HID_NO_BANDWIDTH 11
|
|
#define HID_RESUME_RUNNING 12
|
|
|
|
/*
|
|
* USB-specific HID struct, to be pointed to
|
|
* from struct hid_device->driver_data
|
|
*/
|
|
|
|
struct usbhid_device {
|
|
struct hid_device *hid; /* pointer to corresponding HID dev */
|
|
|
|
struct usb_interface *intf; /* USB interface */
|
|
int ifnum; /* USB interface number */
|
|
|
|
unsigned int bufsize; /* URB buffer size */
|
|
|
|
struct urb *urbin; /* Input URB */
|
|
char *inbuf; /* Input buffer */
|
|
dma_addr_t inbuf_dma; /* Input buffer dma */
|
|
|
|
struct urb *urbctrl; /* Control URB */
|
|
struct usb_ctrlrequest *cr; /* Control request struct */
|
|
struct hid_control_fifo ctrl[HID_CONTROL_FIFO_SIZE]; /* Control fifo */
|
|
unsigned char ctrlhead, ctrltail; /* Control fifo head & tail */
|
|
char *ctrlbuf; /* Control buffer */
|
|
dma_addr_t ctrlbuf_dma; /* Control buffer dma */
|
|
unsigned long last_ctrl; /* record of last output for timeouts */
|
|
|
|
struct urb *urbout; /* Output URB */
|
|
struct hid_output_fifo out[HID_CONTROL_FIFO_SIZE]; /* Output pipe fifo */
|
|
unsigned char outhead, outtail; /* Output pipe fifo head & tail */
|
|
char *outbuf; /* Output buffer */
|
|
dma_addr_t outbuf_dma; /* Output buffer dma */
|
|
unsigned long last_out; /* record of last output for timeouts */
|
|
|
|
spinlock_t lock; /* fifo spinlock */
|
|
unsigned long iofl; /* I/O flags (CTRL_RUNNING, OUT_RUNNING) */
|
|
struct timer_list io_retry; /* Retry timer */
|
|
unsigned long stop_retry; /* Time to give up, in jiffies */
|
|
unsigned int retry_delay; /* Delay length in ms */
|
|
struct work_struct reset_work; /* Task context for resets */
|
|
wait_queue_head_t wait; /* For sleeping */
|
|
};
|
|
|
|
#define hid_to_usb_dev(hid_dev) \
|
|
container_of(hid_dev->dev.parent->parent, struct usb_device, dev)
|
|
|
|
#endif
|
|
|