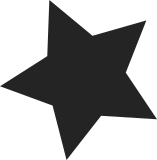
There is no need to have separate kthread for handling USB hub events. It is more elegant to use the workqueue framework. The workqueue is allocated as freezable because the original thread was freezable as well. Also it is allocated as ordered because the code is not ready for parallel processing of hub events, see choose_devnum(). struct usb_hub is passed via the work item. Therefore we do not need hub_event_list. Also hub_thread() is not longer needed. It would call only hub_event(). The rest of the code did manipulate the kthread and it is handled by the workqueue framework now. kick_khubd is renamed to kick_hub_wq() to make the function clear. And the protection against races is done another way, see below. hub_event_lock has been removed. It cannot longer be used to protect struct usb_hub between hub_event() and hub_disconnect(). Instead we need to get hub->kref already in kick_hub_wq(). The lock is not really needed for the other scenarios as well. queue_work() returns whether it succeeded. We could revert the needed operations accordingly. This is enough to avoid duplicity and inconsistencies. Yes, the removed lock causes that there is not longer such a strong synchronization between scheduling the work and manipulating hub->disconnected. But kick_hub_wq() must never be called together with hub_disconnect() otherwise even the original code would have failed. Any callers are responsible for this. Therefore the only problem is that hub_disconnect() could be called in parallel with hub_event(). But this was possible even in the past. struct usb_hub is still guarded by hub->kref and released in hub_events() when needed. Note that the source file is still full of the obsolete "khubd" strings. Let's remove them in a follow up patch. This patch already is complex enough. Thanks a lot Alan Stern <stern@rowland.harvard.edu> for code review, many useful tips and guidance. Also thanks to Tejun Heo <tj@kernel.org> for hints how to allocate the workqueue. Signed-off-by: Petr Mladek <pmladek@suse.cz> Acked-by: Alan Stern <stern@rowland.harvard.edu> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
159 lines
4.7 KiB
C
159 lines
4.7 KiB
C
/*
|
|
* usb hub driver head file
|
|
*
|
|
* Copyright (C) 1999 Linus Torvalds
|
|
* Copyright (C) 1999 Johannes Erdfelt
|
|
* Copyright (C) 1999 Gregory P. Smith
|
|
* Copyright (C) 2001 Brad Hards (bhards@bigpond.net.au)
|
|
* Copyright (C) 2012 Intel Corp (tianyu.lan@intel.com)
|
|
*
|
|
* move struct usb_hub to this file.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
|
|
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License
|
|
* for more details.
|
|
*/
|
|
|
|
#include <linux/usb.h>
|
|
#include <linux/usb/ch11.h>
|
|
#include <linux/usb/hcd.h>
|
|
#include "usb.h"
|
|
|
|
struct usb_hub {
|
|
struct device *intfdev; /* the "interface" device */
|
|
struct usb_device *hdev;
|
|
struct kref kref;
|
|
struct urb *urb; /* for interrupt polling pipe */
|
|
|
|
/* buffer for urb ... with extra space in case of babble */
|
|
u8 (*buffer)[8];
|
|
union {
|
|
struct usb_hub_status hub;
|
|
struct usb_port_status port;
|
|
} *status; /* buffer for status reports */
|
|
struct mutex status_mutex; /* for the status buffer */
|
|
|
|
int error; /* last reported error */
|
|
int nerrors; /* track consecutive errors */
|
|
|
|
unsigned long event_bits[1]; /* status change bitmask */
|
|
unsigned long change_bits[1]; /* ports with logical connect
|
|
status change */
|
|
unsigned long removed_bits[1]; /* ports with a "removed"
|
|
device present */
|
|
unsigned long wakeup_bits[1]; /* ports that have signaled
|
|
remote wakeup */
|
|
unsigned long power_bits[1]; /* ports that are powered */
|
|
unsigned long child_usage_bits[1]; /* ports powered on for
|
|
children */
|
|
unsigned long warm_reset_bits[1]; /* ports requesting warm
|
|
reset recovery */
|
|
#if USB_MAXCHILDREN > 31 /* 8*sizeof(unsigned long) - 1 */
|
|
#error event_bits[] is too short!
|
|
#endif
|
|
|
|
struct usb_hub_descriptor *descriptor; /* class descriptor */
|
|
struct usb_tt tt; /* Transaction Translator */
|
|
|
|
unsigned mA_per_port; /* current for each child */
|
|
#ifdef CONFIG_PM
|
|
unsigned wakeup_enabled_descendants;
|
|
#endif
|
|
|
|
unsigned limited_power:1;
|
|
unsigned quiescing:1;
|
|
unsigned disconnected:1;
|
|
unsigned in_reset:1;
|
|
|
|
unsigned quirk_check_port_auto_suspend:1;
|
|
|
|
unsigned has_indicators:1;
|
|
u8 indicator[USB_MAXCHILDREN];
|
|
struct delayed_work leds;
|
|
struct delayed_work init_work;
|
|
struct work_struct events;
|
|
struct usb_port **ports;
|
|
};
|
|
|
|
/**
|
|
* struct usb port - kernel's representation of a usb port
|
|
* @child: usb device attached to the port
|
|
* @dev: generic device interface
|
|
* @port_owner: port's owner
|
|
* @peer: related usb2 and usb3 ports (share the same connector)
|
|
* @req: default pm qos request for hubs without port power control
|
|
* @connect_type: port's connect type
|
|
* @location: opaque representation of platform connector location
|
|
* @status_lock: synchronize port_event() vs usb_port_{suspend|resume}
|
|
* @portnum: port index num based one
|
|
* @is_superspeed cache super-speed status
|
|
*/
|
|
struct usb_port {
|
|
struct usb_device *child;
|
|
struct device dev;
|
|
struct usb_dev_state *port_owner;
|
|
struct usb_port *peer;
|
|
struct dev_pm_qos_request *req;
|
|
enum usb_port_connect_type connect_type;
|
|
usb_port_location_t location;
|
|
struct mutex status_lock;
|
|
u8 portnum;
|
|
unsigned int is_superspeed:1;
|
|
};
|
|
|
|
#define to_usb_port(_dev) \
|
|
container_of(_dev, struct usb_port, dev)
|
|
|
|
extern int usb_hub_create_port_device(struct usb_hub *hub,
|
|
int port1);
|
|
extern void usb_hub_remove_port_device(struct usb_hub *hub,
|
|
int port1);
|
|
extern int usb_hub_set_port_power(struct usb_device *hdev, struct usb_hub *hub,
|
|
int port1, bool set);
|
|
extern struct usb_hub *usb_hub_to_struct_hub(struct usb_device *hdev);
|
|
extern int hub_port_debounce(struct usb_hub *hub, int port1,
|
|
bool must_be_connected);
|
|
extern int usb_clear_port_feature(struct usb_device *hdev,
|
|
int port1, int feature);
|
|
|
|
static inline bool hub_is_port_power_switchable(struct usb_hub *hub)
|
|
{
|
|
__le16 hcs;
|
|
|
|
if (!hub)
|
|
return false;
|
|
hcs = hub->descriptor->wHubCharacteristics;
|
|
return (le16_to_cpu(hcs) & HUB_CHAR_LPSM) < HUB_CHAR_NO_LPSM;
|
|
}
|
|
|
|
static inline int hub_is_superspeed(struct usb_device *hdev)
|
|
{
|
|
return hdev->descriptor.bDeviceProtocol == USB_HUB_PR_SS;
|
|
}
|
|
|
|
static inline unsigned hub_power_on_good_delay(struct usb_hub *hub)
|
|
{
|
|
unsigned delay = hub->descriptor->bPwrOn2PwrGood * 2;
|
|
|
|
/* Wait at least 100 msec for power to become stable */
|
|
return max(delay, 100U);
|
|
}
|
|
|
|
static inline int hub_port_debounce_be_connected(struct usb_hub *hub,
|
|
int port1)
|
|
{
|
|
return hub_port_debounce(hub, port1, true);
|
|
}
|
|
|
|
static inline int hub_port_debounce_be_stable(struct usb_hub *hub,
|
|
int port1)
|
|
{
|
|
return hub_port_debounce(hub, port1, false);
|
|
}
|
|
|