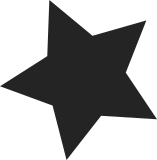
might_sleep() and smp_processor_id() checks are enabled after the boot process is done. That hides bugs in the SMP bringup and driver initialization code. Enable it right when the scheduler starts working, i.e. when init task and kthreadd have been created and right before the idle task enables preemption. Tested-by: Mark Rutland <mark.rutland@arm.com> Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Signed-off-by: Peter Zijlstra (Intel) <peterz@infradead.org> Acked-by: Mark Rutland <mark.rutland@arm.com> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Steven Rostedt <rostedt@goodmis.org> Link: http://lkml.kernel.org/r/20170516184736.272225698@linutronix.de Signed-off-by: Ingo Molnar <mingo@kernel.org>
65 lines
1.3 KiB
C
65 lines
1.3 KiB
C
/*
|
|
* lib/smp_processor_id.c
|
|
*
|
|
* DEBUG_PREEMPT variant of smp_processor_id().
|
|
*/
|
|
#include <linux/export.h>
|
|
#include <linux/kallsyms.h>
|
|
#include <linux/sched.h>
|
|
|
|
notrace static unsigned int check_preemption_disabled(const char *what1,
|
|
const char *what2)
|
|
{
|
|
int this_cpu = raw_smp_processor_id();
|
|
|
|
if (likely(preempt_count()))
|
|
goto out;
|
|
|
|
if (irqs_disabled())
|
|
goto out;
|
|
|
|
/*
|
|
* Kernel threads bound to a single CPU can safely use
|
|
* smp_processor_id():
|
|
*/
|
|
if (cpumask_equal(¤t->cpus_allowed, cpumask_of(this_cpu)))
|
|
goto out;
|
|
|
|
/*
|
|
* It is valid to assume CPU-locality during early bootup:
|
|
*/
|
|
if (system_state < SYSTEM_SCHEDULING)
|
|
goto out;
|
|
|
|
/*
|
|
* Avoid recursion:
|
|
*/
|
|
preempt_disable_notrace();
|
|
|
|
if (!printk_ratelimit())
|
|
goto out_enable;
|
|
|
|
printk(KERN_ERR "BUG: using %s%s() in preemptible [%08x] code: %s/%d\n",
|
|
what1, what2, preempt_count() - 1, current->comm, current->pid);
|
|
|
|
print_symbol("caller is %s\n", (long)__builtin_return_address(0));
|
|
dump_stack();
|
|
|
|
out_enable:
|
|
preempt_enable_no_resched_notrace();
|
|
out:
|
|
return this_cpu;
|
|
}
|
|
|
|
notrace unsigned int debug_smp_processor_id(void)
|
|
{
|
|
return check_preemption_disabled("smp_processor_id", "");
|
|
}
|
|
EXPORT_SYMBOL(debug_smp_processor_id);
|
|
|
|
notrace void __this_cpu_preempt_check(const char *op)
|
|
{
|
|
check_preemption_disabled("__this_cpu_", op);
|
|
}
|
|
EXPORT_SYMBOL(__this_cpu_preempt_check);
|