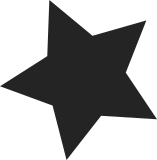
Right now we use the 'readlock' both for protecting some of the af_unix
IO path and for making the bind be single-threaded.
The two are independent, but using the same lock makes for a nasty
deadlock due to ordering with regards to filesystem locking. The bind
locking would want to nest outside the VSF pathname locking, but the IO
locking wants to nest inside some of those same locks.
We tried to fix this earlier with commit c845acb324
("af_unix: Fix
splice-bind deadlock") which moved the readlock inside the vfs locks,
but that caused problems with overlayfs that will then call back into
filesystem routines that take the lock in the wrong order anyway.
Splitting the locks means that we can go back to having the bind lock be
the outermost lock, and we don't have any deadlocks with lock ordering.
Acked-by: Rainer Weikusat <rweikusat@cyberadapt.com>
Acked-by: Al Viro <viro@zeniv.linux.org.uk>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
Acked-by: Hannes Frederic Sowa <hannes@stressinduktion.org>
Signed-off-by: David S. Miller <davem@davemloft.net>
86 lines
2.1 KiB
C
86 lines
2.1 KiB
C
#ifndef __LINUX_NET_AFUNIX_H
|
|
#define __LINUX_NET_AFUNIX_H
|
|
|
|
#include <linux/socket.h>
|
|
#include <linux/un.h>
|
|
#include <linux/mutex.h>
|
|
#include <net/sock.h>
|
|
|
|
void unix_inflight(struct user_struct *user, struct file *fp);
|
|
void unix_notinflight(struct user_struct *user, struct file *fp);
|
|
void unix_gc(void);
|
|
void wait_for_unix_gc(void);
|
|
struct sock *unix_get_socket(struct file *filp);
|
|
struct sock *unix_peer_get(struct sock *);
|
|
|
|
#define UNIX_HASH_SIZE 256
|
|
#define UNIX_HASH_BITS 8
|
|
|
|
extern unsigned int unix_tot_inflight;
|
|
extern spinlock_t unix_table_lock;
|
|
extern struct hlist_head unix_socket_table[2 * UNIX_HASH_SIZE];
|
|
|
|
struct unix_address {
|
|
atomic_t refcnt;
|
|
int len;
|
|
unsigned int hash;
|
|
struct sockaddr_un name[0];
|
|
};
|
|
|
|
struct unix_skb_parms {
|
|
struct pid *pid; /* Skb credentials */
|
|
kuid_t uid;
|
|
kgid_t gid;
|
|
struct scm_fp_list *fp; /* Passed files */
|
|
#ifdef CONFIG_SECURITY_NETWORK
|
|
u32 secid; /* Security ID */
|
|
#endif
|
|
u32 consumed;
|
|
};
|
|
|
|
#define UNIXCB(skb) (*(struct unix_skb_parms *)&((skb)->cb))
|
|
|
|
#define unix_state_lock(s) spin_lock(&unix_sk(s)->lock)
|
|
#define unix_state_unlock(s) spin_unlock(&unix_sk(s)->lock)
|
|
#define unix_state_lock_nested(s) \
|
|
spin_lock_nested(&unix_sk(s)->lock, \
|
|
SINGLE_DEPTH_NESTING)
|
|
|
|
/* The AF_UNIX socket */
|
|
struct unix_sock {
|
|
/* WARNING: sk has to be the first member */
|
|
struct sock sk;
|
|
struct unix_address *addr;
|
|
struct path path;
|
|
struct mutex iolock, bindlock;
|
|
struct sock *peer;
|
|
struct list_head link;
|
|
atomic_long_t inflight;
|
|
spinlock_t lock;
|
|
unsigned char recursion_level;
|
|
unsigned long gc_flags;
|
|
#define UNIX_GC_CANDIDATE 0
|
|
#define UNIX_GC_MAYBE_CYCLE 1
|
|
struct socket_wq peer_wq;
|
|
wait_queue_t peer_wake;
|
|
};
|
|
|
|
static inline struct unix_sock *unix_sk(const struct sock *sk)
|
|
{
|
|
return (struct unix_sock *)sk;
|
|
}
|
|
|
|
#define peer_wait peer_wq.wait
|
|
|
|
long unix_inq_len(struct sock *sk);
|
|
long unix_outq_len(struct sock *sk);
|
|
|
|
#ifdef CONFIG_SYSCTL
|
|
int unix_sysctl_register(struct net *net);
|
|
void unix_sysctl_unregister(struct net *net);
|
|
#else
|
|
static inline int unix_sysctl_register(struct net *net) { return 0; }
|
|
static inline void unix_sysctl_unregister(struct net *net) {}
|
|
#endif
|
|
#endif
|