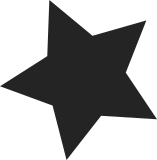
The DesignWare I2C controller has high count (HCNT) and low count (LCNT) registers for each of the I2C speed modes (standard and fast). These registers are programmed based on the input clock speed in the driver. The current code calculates these values based on the input clock speed and tries hard to meet the I2C bus timing requirements. This could result non-optimal values with regarding to the bus speed. For example on Intel BayTrail we get bus speed of 315.41kHz which is ~20% slower than we would expect (400kHz) in fast mode (even though the timing requirements are met). This patch makes it possible for the platform code to pass more optimal HCNT/LCNT values to the core driver if they are known beforehand. If these are not set we use the calculated and more conservative values. Signed-off-by: Mika Westerberg <mika.westerberg@linux.intel.com> Acked-by: Shinya Kuribayashi <skuribay@pobox.com> Signed-off-by: Wolfram Sang <wsa@the-dreams.de>
124 lines
4.2 KiB
C
124 lines
4.2 KiB
C
/*
|
|
* Synopsys DesignWare I2C adapter driver (master only).
|
|
*
|
|
* Based on the TI DAVINCI I2C adapter driver.
|
|
*
|
|
* Copyright (C) 2006 Texas Instruments.
|
|
* Copyright (C) 2007 MontaVista Software Inc.
|
|
* Copyright (C) 2009 Provigent Ltd.
|
|
*
|
|
* ----------------------------------------------------------------------------
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
* ----------------------------------------------------------------------------
|
|
*
|
|
*/
|
|
|
|
|
|
#define DW_IC_CON_MASTER 0x1
|
|
#define DW_IC_CON_SPEED_STD 0x2
|
|
#define DW_IC_CON_SPEED_FAST 0x4
|
|
#define DW_IC_CON_10BITADDR_MASTER 0x10
|
|
#define DW_IC_CON_RESTART_EN 0x20
|
|
#define DW_IC_CON_SLAVE_DISABLE 0x40
|
|
|
|
|
|
/**
|
|
* struct dw_i2c_dev - private i2c-designware data
|
|
* @dev: driver model device node
|
|
* @base: IO registers pointer
|
|
* @cmd_complete: tx completion indicator
|
|
* @lock: protect this struct and IO registers
|
|
* @clk: input reference clock
|
|
* @cmd_err: run time hadware error code
|
|
* @msgs: points to an array of messages currently being transfered
|
|
* @msgs_num: the number of elements in msgs
|
|
* @msg_write_idx: the element index of the current tx message in the msgs
|
|
* array
|
|
* @tx_buf_len: the length of the current tx buffer
|
|
* @tx_buf: the current tx buffer
|
|
* @msg_read_idx: the element index of the current rx message in the msgs
|
|
* array
|
|
* @rx_buf_len: the length of the current rx buffer
|
|
* @rx_buf: the current rx buffer
|
|
* @msg_err: error status of the current transfer
|
|
* @status: i2c master status, one of STATUS_*
|
|
* @abort_source: copy of the TX_ABRT_SOURCE register
|
|
* @irq: interrupt number for the i2c master
|
|
* @adapter: i2c subsystem adapter node
|
|
* @tx_fifo_depth: depth of the hardware tx fifo
|
|
* @rx_fifo_depth: depth of the hardware rx fifo
|
|
* @rx_outstanding: current master-rx elements in tx fifo
|
|
* @ss_hcnt: standard speed HCNT value
|
|
* @ss_lcnt: standard speed LCNT value
|
|
* @fs_hcnt: fast speed HCNT value
|
|
* @fs_lcnt: fast speed LCNT value
|
|
*
|
|
* HCNT and LCNT parameters can be used if the platform knows more accurate
|
|
* values than the one computed based only on the input clock frequency.
|
|
* Leave them to be %0 if not used.
|
|
*/
|
|
struct dw_i2c_dev {
|
|
struct device *dev;
|
|
void __iomem *base;
|
|
struct completion cmd_complete;
|
|
struct mutex lock;
|
|
struct clk *clk;
|
|
u32 (*get_clk_rate_khz) (struct dw_i2c_dev *dev);
|
|
struct dw_pci_controller *controller;
|
|
int cmd_err;
|
|
struct i2c_msg *msgs;
|
|
int msgs_num;
|
|
int msg_write_idx;
|
|
u32 tx_buf_len;
|
|
u8 *tx_buf;
|
|
int msg_read_idx;
|
|
u32 rx_buf_len;
|
|
u8 *rx_buf;
|
|
int msg_err;
|
|
unsigned int status;
|
|
u32 abort_source;
|
|
int irq;
|
|
u32 accessor_flags;
|
|
struct i2c_adapter adapter;
|
|
u32 functionality;
|
|
u32 master_cfg;
|
|
unsigned int tx_fifo_depth;
|
|
unsigned int rx_fifo_depth;
|
|
int rx_outstanding;
|
|
u32 sda_hold_time;
|
|
u16 ss_hcnt;
|
|
u16 ss_lcnt;
|
|
u16 fs_hcnt;
|
|
u16 fs_lcnt;
|
|
};
|
|
|
|
#define ACCESS_SWAP 0x00000001
|
|
#define ACCESS_16BIT 0x00000002
|
|
|
|
extern u32 dw_readl(struct dw_i2c_dev *dev, int offset);
|
|
extern void dw_writel(struct dw_i2c_dev *dev, u32 b, int offset);
|
|
extern int i2c_dw_init(struct dw_i2c_dev *dev);
|
|
extern int i2c_dw_xfer(struct i2c_adapter *adap, struct i2c_msg msgs[],
|
|
int num);
|
|
extern u32 i2c_dw_func(struct i2c_adapter *adap);
|
|
extern irqreturn_t i2c_dw_isr(int this_irq, void *dev_id);
|
|
extern void i2c_dw_enable(struct dw_i2c_dev *dev);
|
|
extern u32 i2c_dw_is_enabled(struct dw_i2c_dev *dev);
|
|
extern void i2c_dw_disable(struct dw_i2c_dev *dev);
|
|
extern void i2c_dw_clear_int(struct dw_i2c_dev *dev);
|
|
extern void i2c_dw_disable_int(struct dw_i2c_dev *dev);
|
|
extern u32 i2c_dw_read_comp_param(struct dw_i2c_dev *dev);
|