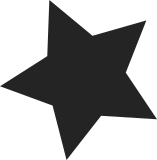
The CLOCKSOURCE_OF_DECLARE macro is used widely for the timers to declare the clocksource at early stage. However, this macro is also used to initialize the clockevent if any, or the clockevent only. It was originally suggested to declare another macro to initialize a clockevent, so in order to separate the two entities even they belong to the same IP. This was not accepted because of the impact on the DT where splitting a clocksource/clockevent definition does not make sense as it is a Linux concept not a hardware description. On the other side, the clocksource has not interrupt declared while the clockevent has, so it is easy from the driver to know if the description is for a clockevent or a clocksource, IOW it could be implemented at the driver level. So instead of dealing with a named clocksource macro, let's use a more generic one: TIMER_OF_DECLARE. The patch has not functional changes. Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org> Acked-by: Heiko Stuebner <heiko@sntech.de> Acked-by: Neil Armstrong <narmstrong@baylibre.com> Acked-by: Arnd Bergmann <arnd@arndb.de> Acked-by: Matthias Brugger <matthias.bgg@gmail.com> Reviewed-by: Linus Walleij <linus.walleij@linaro.org>
91 lines
2.5 KiB
C
91 lines
2.5 KiB
C
/*
|
|
* Copyright (C) ST-Ericsson SA 2011
|
|
*
|
|
* License Terms: GNU General Public License v2
|
|
* Author: Mattias Wallin <mattias.wallin@stericsson.com> for ST-Ericsson
|
|
* Author: Sundar Iyer for ST-Ericsson
|
|
* sched_clock implementation is based on:
|
|
* plat-nomadik/timer.c Linus Walleij <linus.walleij@stericsson.com>
|
|
*
|
|
* DBx500-PRCMU Timer
|
|
* The PRCMU has 5 timers which are available in a always-on
|
|
* power domain. We use the Timer 4 for our always-on clock
|
|
* source on DB8500.
|
|
*/
|
|
#include <linux/of.h>
|
|
#include <linux/of_address.h>
|
|
#include <linux/clockchips.h>
|
|
#include <linux/sched_clock.h>
|
|
|
|
#define RATE_32K 32768
|
|
|
|
#define TIMER_MODE_CONTINOUS 0x1
|
|
#define TIMER_DOWNCOUNT_VAL 0xffffffff
|
|
|
|
#define PRCMU_TIMER_REF 0
|
|
#define PRCMU_TIMER_DOWNCOUNT 0x4
|
|
#define PRCMU_TIMER_MODE 0x8
|
|
|
|
#define SCHED_CLOCK_MIN_WRAP 131072 /* 2^32 / 32768 */
|
|
|
|
static void __iomem *clksrc_dbx500_timer_base;
|
|
|
|
static u64 notrace clksrc_dbx500_prcmu_read(struct clocksource *cs)
|
|
{
|
|
void __iomem *base = clksrc_dbx500_timer_base;
|
|
u32 count, count2;
|
|
|
|
do {
|
|
count = readl_relaxed(base + PRCMU_TIMER_DOWNCOUNT);
|
|
count2 = readl_relaxed(base + PRCMU_TIMER_DOWNCOUNT);
|
|
} while (count2 != count);
|
|
|
|
/* Negate because the timer is a decrementing counter */
|
|
return ~count;
|
|
}
|
|
|
|
static struct clocksource clocksource_dbx500_prcmu = {
|
|
.name = "dbx500-prcmu-timer",
|
|
.rating = 300,
|
|
.read = clksrc_dbx500_prcmu_read,
|
|
.mask = CLOCKSOURCE_MASK(32),
|
|
.flags = CLOCK_SOURCE_IS_CONTINUOUS,
|
|
};
|
|
|
|
#ifdef CONFIG_CLKSRC_DBX500_PRCMU_SCHED_CLOCK
|
|
|
|
static u64 notrace dbx500_prcmu_sched_clock_read(void)
|
|
{
|
|
if (unlikely(!clksrc_dbx500_timer_base))
|
|
return 0;
|
|
|
|
return clksrc_dbx500_prcmu_read(&clocksource_dbx500_prcmu);
|
|
}
|
|
|
|
#endif
|
|
|
|
static int __init clksrc_dbx500_prcmu_init(struct device_node *node)
|
|
{
|
|
clksrc_dbx500_timer_base = of_iomap(node, 0);
|
|
|
|
/*
|
|
* The A9 sub system expects the timer to be configured as
|
|
* a continous looping timer.
|
|
* The PRCMU should configure it but if it for some reason
|
|
* don't we do it here.
|
|
*/
|
|
if (readl(clksrc_dbx500_timer_base + PRCMU_TIMER_MODE) !=
|
|
TIMER_MODE_CONTINOUS) {
|
|
writel(TIMER_MODE_CONTINOUS,
|
|
clksrc_dbx500_timer_base + PRCMU_TIMER_MODE);
|
|
writel(TIMER_DOWNCOUNT_VAL,
|
|
clksrc_dbx500_timer_base + PRCMU_TIMER_REF);
|
|
}
|
|
#ifdef CONFIG_CLKSRC_DBX500_PRCMU_SCHED_CLOCK
|
|
sched_clock_register(dbx500_prcmu_sched_clock_read, 32, RATE_32K);
|
|
#endif
|
|
return clocksource_register_hz(&clocksource_dbx500_prcmu, RATE_32K);
|
|
}
|
|
TIMER_OF_DECLARE(dbx500_prcmu, "stericsson,db8500-prcmu-timer-4",
|
|
clksrc_dbx500_prcmu_init);
|