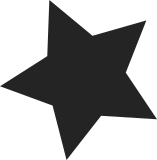
By moving these client drivers to use RPMSG instead of the direct SMD API we can reuse them ontop of the newly added GLINK wire-protocol support found in the 820 and 835 Qualcomm platforms. As the new (RPMSG-based) and old SMD implementations are mutually exclusive we have to change all client drivers in one commit, to make sure we have a working system before and after this transition. Acked-by: Andy Gross <andy.gross@linaro.org> Acked-by: Kalle Valo <kvalo@codeaurora.org> Acked-by: Marcel Holtmann <marcel@holtmann.org> Signed-off-by: Bjorn Andersson <bjorn.andersson@linaro.org> Signed-off-by: David S. Miller <davem@davemloft.net>
119 lines
2.7 KiB
C
119 lines
2.7 KiB
C
/*
|
|
* Copyright (c) 2015, Sony Mobile Communications Inc.
|
|
* Copyright (c) 2013, The Linux Foundation. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 and
|
|
* only version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*/
|
|
|
|
#include <linux/module.h>
|
|
#include <linux/skbuff.h>
|
|
#include <linux/rpmsg.h>
|
|
|
|
#include "qrtr.h"
|
|
|
|
struct qrtr_smd_dev {
|
|
struct qrtr_endpoint ep;
|
|
struct rpmsg_endpoint *channel;
|
|
struct device *dev;
|
|
};
|
|
|
|
/* from smd to qrtr */
|
|
static int qcom_smd_qrtr_callback(struct rpmsg_device *rpdev,
|
|
void *data, int len, void *priv, u32 addr)
|
|
{
|
|
struct qrtr_smd_dev *qdev = dev_get_drvdata(&rpdev->dev);
|
|
int rc;
|
|
|
|
if (!qdev)
|
|
return -EAGAIN;
|
|
|
|
rc = qrtr_endpoint_post(&qdev->ep, data, len);
|
|
if (rc == -EINVAL) {
|
|
dev_err(qdev->dev, "invalid ipcrouter packet\n");
|
|
/* return 0 to let smd drop the packet */
|
|
rc = 0;
|
|
}
|
|
|
|
return rc;
|
|
}
|
|
|
|
/* from qrtr to smd */
|
|
static int qcom_smd_qrtr_send(struct qrtr_endpoint *ep, struct sk_buff *skb)
|
|
{
|
|
struct qrtr_smd_dev *qdev = container_of(ep, struct qrtr_smd_dev, ep);
|
|
int rc;
|
|
|
|
rc = skb_linearize(skb);
|
|
if (rc)
|
|
goto out;
|
|
|
|
rc = rpmsg_send(qdev->channel, skb->data, skb->len);
|
|
|
|
out:
|
|
if (rc)
|
|
kfree_skb(skb);
|
|
else
|
|
consume_skb(skb);
|
|
return rc;
|
|
}
|
|
|
|
static int qcom_smd_qrtr_probe(struct rpmsg_device *rpdev)
|
|
{
|
|
struct qrtr_smd_dev *qdev;
|
|
int rc;
|
|
|
|
qdev = devm_kzalloc(&rpdev->dev, sizeof(*qdev), GFP_KERNEL);
|
|
if (!qdev)
|
|
return -ENOMEM;
|
|
|
|
qdev->channel = rpdev->ept;
|
|
qdev->dev = &rpdev->dev;
|
|
qdev->ep.xmit = qcom_smd_qrtr_send;
|
|
|
|
rc = qrtr_endpoint_register(&qdev->ep, QRTR_EP_NID_AUTO);
|
|
if (rc)
|
|
return rc;
|
|
|
|
dev_set_drvdata(&rpdev->dev, qdev);
|
|
|
|
dev_dbg(&rpdev->dev, "Qualcomm SMD QRTR driver probed\n");
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void qcom_smd_qrtr_remove(struct rpmsg_device *rpdev)
|
|
{
|
|
struct qrtr_smd_dev *qdev = dev_get_drvdata(&rpdev->dev);
|
|
|
|
qrtr_endpoint_unregister(&qdev->ep);
|
|
|
|
dev_set_drvdata(&rpdev->dev, NULL);
|
|
}
|
|
|
|
static const struct rpmsg_device_id qcom_smd_qrtr_smd_match[] = {
|
|
{ "IPCRTR" },
|
|
{}
|
|
};
|
|
|
|
static struct rpmsg_driver qcom_smd_qrtr_driver = {
|
|
.probe = qcom_smd_qrtr_probe,
|
|
.remove = qcom_smd_qrtr_remove,
|
|
.callback = qcom_smd_qrtr_callback,
|
|
.id_table = qcom_smd_qrtr_smd_match,
|
|
.drv = {
|
|
.name = "qcom_smd_qrtr",
|
|
},
|
|
};
|
|
|
|
module_rpmsg_driver(qcom_smd_qrtr_driver);
|
|
|
|
MODULE_DESCRIPTION("Qualcomm IPC-Router SMD interface driver");
|
|
MODULE_LICENSE("GPL v2");
|