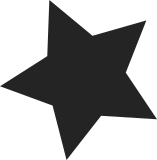
Backlight class attributes are currently easy to implement incorrectly. Moving certain handling into the backlight core prevents this whilst at the same time makes the drivers simpler and consistent. The following changes are included: The brightness attribute only sets and reads the brightness variable in the backlight_properties structure. The power attribute only sets and reads the power variable in the backlight_properties structure. Any framebuffer blanking events change a variable fb_blank in the backlight_properties structure. The backlight driver has only two functions to implement. One function is called when any of the above properties change (to update the backlight brightness), the second is called to return the current backlight brightness value. A new attribute "actual_brightness" is added to return this brightness as determined by the driver having combined all the above factors (and any driver/device specific factors). Additionally, the backlight core takes care of checking the maximum brightness is not exceeded and of turning off the backlight before device removal. The corgi backlight driver is updated to reflect these changes. Signed-off-by: Richard Purdie <rpurdie@rpsys.net> Signed-off-by: Antonino Daplas <adaplas@pol.net> Signed-off-by: Andrew Morton <akpm@osdl.org> Signed-off-by: Linus Torvalds <torvalds@osdl.org>
63 lines
1.9 KiB
C
63 lines
1.9 KiB
C
/*
|
|
* Backlight Lowlevel Control Abstraction
|
|
*
|
|
* Copyright (C) 2003,2004 Hewlett-Packard Company
|
|
*
|
|
*/
|
|
|
|
#ifndef _LINUX_BACKLIGHT_H
|
|
#define _LINUX_BACKLIGHT_H
|
|
|
|
#include <linux/device.h>
|
|
#include <linux/notifier.h>
|
|
|
|
struct backlight_device;
|
|
struct fb_info;
|
|
|
|
/* This structure defines all the properties of a backlight
|
|
(usually attached to a LCD). */
|
|
struct backlight_properties {
|
|
/* Owner module */
|
|
struct module *owner;
|
|
|
|
/* Notify the backlight driver some property has changed */
|
|
int (*update_status)(struct backlight_device *);
|
|
/* Return the current backlight brightness (accounting for power,
|
|
fb_blank etc.) */
|
|
int (*get_brightness)(struct backlight_device *);
|
|
/* Check if given framebuffer device is the one bound to this backlight;
|
|
return 0 if not, !=0 if it is. If NULL, backlight always matches the fb. */
|
|
int (*check_fb)(struct fb_info *);
|
|
|
|
/* Current User requested brightness (0 - max_brightness) */
|
|
int brightness;
|
|
/* Maximal value for brightness (read-only) */
|
|
int max_brightness;
|
|
/* Current FB Power mode (0: full on, 1..3: power saving
|
|
modes; 4: full off), see FB_BLANK_XXX */
|
|
int power;
|
|
/* FB Blanking active? (values as for power) */
|
|
int fb_blank;
|
|
};
|
|
|
|
struct backlight_device {
|
|
/* This protects the 'props' field. If 'props' is NULL, the driver that
|
|
registered this device has been unloaded, and if class_get_devdata()
|
|
points to something in the body of that driver, it is also invalid. */
|
|
struct semaphore sem;
|
|
/* If this is NULL, the backing module is unloaded */
|
|
struct backlight_properties *props;
|
|
/* The framebuffer notifier block */
|
|
struct notifier_block fb_notif;
|
|
/* The class device structure */
|
|
struct class_device class_dev;
|
|
};
|
|
|
|
extern struct backlight_device *backlight_device_register(const char *name,
|
|
void *devdata, struct backlight_properties *bp);
|
|
extern void backlight_device_unregister(struct backlight_device *bd);
|
|
|
|
#define to_backlight_device(obj) container_of(obj, struct backlight_device, class_dev)
|
|
|
|
#endif
|