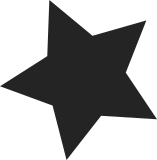
In the case of a device tree config the code uses the device ID from the DT entry to check which codec is required but when storing the ID into struct arizona it was always using the non-DT SPI device table to get an ID. This patch changes the code to store the correct ID into struct arizona. Signed-off-by: Richard Fitzgerald <rf@opensource.wolfsonmicro.com> Acked-by: Lee Jones <lee.jones@linaro.org> Signed-off-by: Charles Keepax <ckeepax@opensource.wolfsonmicro.com> Signed-off-by: Lee Jones <lee.jones@linaro.org>
122 lines
2.8 KiB
C
122 lines
2.8 KiB
C
/*
|
|
* Arizona-i2c.c -- Arizona I2C bus interface
|
|
*
|
|
* Copyright 2012 Wolfson Microelectronics plc
|
|
*
|
|
* Author: Mark Brown <broonie@opensource.wolfsonmicro.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/err.h>
|
|
#include <linux/i2c.h>
|
|
#include <linux/module.h>
|
|
#include <linux/pm_runtime.h>
|
|
#include <linux/regmap.h>
|
|
#include <linux/regulator/consumer.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/of.h>
|
|
|
|
#include <linux/mfd/arizona/core.h>
|
|
|
|
#include "arizona.h"
|
|
|
|
static int arizona_i2c_probe(struct i2c_client *i2c,
|
|
const struct i2c_device_id *id)
|
|
{
|
|
struct arizona *arizona;
|
|
const struct regmap_config *regmap_config = NULL;
|
|
unsigned long type;
|
|
int ret;
|
|
|
|
if (i2c->dev.of_node)
|
|
type = arizona_of_get_type(&i2c->dev);
|
|
else
|
|
type = id->driver_data;
|
|
|
|
switch (type) {
|
|
case WM5102:
|
|
if (IS_ENABLED(CONFIG_MFD_WM5102))
|
|
regmap_config = &wm5102_i2c_regmap;
|
|
break;
|
|
case WM5110:
|
|
case WM8280:
|
|
if (IS_ENABLED(CONFIG_MFD_WM5110))
|
|
regmap_config = &wm5110_i2c_regmap;
|
|
break;
|
|
case WM8997:
|
|
if (IS_ENABLED(CONFIG_MFD_WM8997))
|
|
regmap_config = &wm8997_i2c_regmap;
|
|
break;
|
|
case WM8998:
|
|
case WM1814:
|
|
if (IS_ENABLED(CONFIG_MFD_WM8998))
|
|
regmap_config = &wm8998_i2c_regmap;
|
|
break;
|
|
default:
|
|
dev_err(&i2c->dev, "Unknown device type %ld\n", type);
|
|
return -EINVAL;
|
|
}
|
|
|
|
if (!regmap_config) {
|
|
dev_err(&i2c->dev,
|
|
"No kernel support for device type %ld\n", type);
|
|
return -EINVAL;
|
|
}
|
|
|
|
arizona = devm_kzalloc(&i2c->dev, sizeof(*arizona), GFP_KERNEL);
|
|
if (arizona == NULL)
|
|
return -ENOMEM;
|
|
|
|
arizona->regmap = devm_regmap_init_i2c(i2c, regmap_config);
|
|
if (IS_ERR(arizona->regmap)) {
|
|
ret = PTR_ERR(arizona->regmap);
|
|
dev_err(&i2c->dev, "Failed to allocate register map: %d\n",
|
|
ret);
|
|
return ret;
|
|
}
|
|
|
|
arizona->type = type;
|
|
arizona->dev = &i2c->dev;
|
|
arizona->irq = i2c->irq;
|
|
|
|
return arizona_dev_init(arizona);
|
|
}
|
|
|
|
static int arizona_i2c_remove(struct i2c_client *i2c)
|
|
{
|
|
struct arizona *arizona = dev_get_drvdata(&i2c->dev);
|
|
arizona_dev_exit(arizona);
|
|
return 0;
|
|
}
|
|
|
|
static const struct i2c_device_id arizona_i2c_id[] = {
|
|
{ "wm5102", WM5102 },
|
|
{ "wm5110", WM5110 },
|
|
{ "wm8280", WM8280 },
|
|
{ "wm8997", WM8997 },
|
|
{ "wm8998", WM8998 },
|
|
{ "wm1814", WM1814 },
|
|
{ }
|
|
};
|
|
MODULE_DEVICE_TABLE(i2c, arizona_i2c_id);
|
|
|
|
static struct i2c_driver arizona_i2c_driver = {
|
|
.driver = {
|
|
.name = "arizona",
|
|
.pm = &arizona_pm_ops,
|
|
.of_match_table = of_match_ptr(arizona_of_match),
|
|
},
|
|
.probe = arizona_i2c_probe,
|
|
.remove = arizona_i2c_remove,
|
|
.id_table = arizona_i2c_id,
|
|
};
|
|
|
|
module_i2c_driver(arizona_i2c_driver);
|
|
|
|
MODULE_DESCRIPTION("Arizona I2C bus interface");
|
|
MODULE_AUTHOR("Mark Brown <broonie@opensource.wolfsonmicro.com>");
|
|
MODULE_LICENSE("GPL");
|