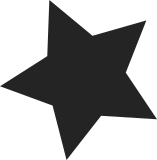
renesas_usbhs implements ->pullup() method, switches over to devm_request_irq(), adds support for DMA Engine and got a few miscelaneous cleanups. The NCM gadget got an endianness fix and the Ethernet gadget a frame size fix. We're finally removing the g_file_storage gadget and sticking to g_mass_storage and the new tcm_usb_gadget gadgets since that was a huge duplicaton of effort anyway. While removing g_file_storage, we also had to fix a bunch of defconfigs which were still pointing to the old gadget. There's a big series getting us closer to being able to introduce our configfs interface. The series converts functions into loadable modules which will, eventually, be registered to the configfs interface. Other than that there's the usual typo fixes and miscelaneous cleanups all over the place. -----BEGIN PGP SIGNATURE----- Version: GnuPG v1.4.12 (GNU/Linux) iQIcBAABAgAGBQJQnXPMAAoJEIaOsuA1yqREkygQALIuhY6veRPZoZJltuADeAOV h7lBkuseJxvlJsbMLnjqP5tw4W/haE1deGR+ee1ZItkPrERCX1++jkQ6hmm7e00R mvr8rI+n3eBHSKUO89tUfCaz5UBsTl0cowPWdTwxRrV4VRJ1wVBw/oII9sfyss03 jDo+11DSjTGTB+Bz72p2NTkRiv9my2Kz+ihhqFR5VSl5FyoutG53RNKRmciJKGB+ i+RptOI+prdW1uOURHbie5FAI0xOBrE1Up2XdNiZ9blT6zcsK754Lc8erFJEZXX5 7s8Ys/HJZLQCF/fRt4WAw8e1lSPELD2xuDMqV+WKu93aXOiAWL1SbzqK3Y+PaUDg Red07jOxgPqgq0F1mAp3+0Rs1RnshSvKREtQhZqsttg7suXhDB0q7h61CX8uQbRA hBZh8eFexRjqOZxveeV+h4ATz00c2nlEa8cJscr5zLf4R/LSxJWT7LV5227BDkBV 9NUMA3dunDYZLqnxBv5lS2gQzmYO6G11wzdpgjnABL2WlM8Pv1lUDhY+erwvTRzd BM+9qMd7K40BuI1JyUsbBdmuEpJAD/yWE77pT2aBrr4767x0CYjBPZqQAxXFcWi8 5NG1BzqWmH9HhwxKyWueWgNgY253cRcAzFlUN80NRA2UuNkMAeOAeJjvK48isAqJ T1MUkQgIFNvSecpRPrEl =umtl -----END PGP SIGNATURE----- Merge tag 'gadget-for-v3.8' of git://git.kernel.org/pub/scm/linux/kernel/git/balbi/usb into usb-next USB gadget patches from Felipe: "usb: gadget: patches for v3.8 renesas_usbhs implements ->pullup() method, switches over to devm_request_irq(), adds support for DMA Engine and got a few miscelaneous cleanups. The NCM gadget got an endianness fix and the Ethernet gadget a frame size fix. We're finally removing the g_file_storage gadget and sticking to g_mass_storage and the new tcm_usb_gadget gadgets since that was a huge duplicaton of effort anyway. While removing g_file_storage, we also had to fix a bunch of defconfigs which were still pointing to the old gadget. There's a big series getting us closer to being able to introduce our configfs interface. The series converts functions into loadable modules which will, eventually, be registered to the configfs interface. Other than that there's the usual typo fixes and miscelaneous cleanups all over the place."
117 lines
3.6 KiB
C
117 lines
3.6 KiB
C
/*
|
|
* Renesas USB driver
|
|
*
|
|
* Copyright (C) 2011 Renesas Solutions Corp.
|
|
* Kuninori Morimoto <kuninori.morimoto.gx@renesas.com>
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*
|
|
*/
|
|
#ifndef RENESAS_USB_PIPE_H
|
|
#define RENESAS_USB_PIPE_H
|
|
|
|
#include "common.h"
|
|
#include "fifo.h"
|
|
|
|
/*
|
|
* struct
|
|
*/
|
|
struct usbhs_pipe {
|
|
u32 pipe_type; /* USB_ENDPOINT_XFER_xxx */
|
|
|
|
struct usbhs_priv *priv;
|
|
struct usbhs_fifo *fifo;
|
|
struct list_head list;
|
|
|
|
int maxp;
|
|
|
|
u32 flags;
|
|
#define USBHS_PIPE_FLAGS_IS_USED (1 << 0)
|
|
#define USBHS_PIPE_FLAGS_IS_DIR_IN (1 << 1)
|
|
#define USBHS_PIPE_FLAGS_IS_DIR_HOST (1 << 2)
|
|
|
|
struct usbhs_pkt_handle *handler;
|
|
|
|
void *mod_private;
|
|
};
|
|
|
|
struct usbhs_pipe_info {
|
|
struct usbhs_pipe *pipe;
|
|
int size; /* array size of "pipe" */
|
|
int bufnmb_last; /* FIXME : driver needs good allocator */
|
|
|
|
int (*dma_map_ctrl)(struct usbhs_pkt *pkt, int map);
|
|
};
|
|
|
|
/*
|
|
* pipe list
|
|
*/
|
|
#define __usbhs_for_each_pipe(start, pos, info, i) \
|
|
for (i = start, pos = (info)->pipe + i; \
|
|
i < (info)->size; \
|
|
i++, pos = (info)->pipe + i)
|
|
|
|
#define usbhs_for_each_pipe(pos, priv, i) \
|
|
__usbhs_for_each_pipe(1, pos, &((priv)->pipe_info), i)
|
|
|
|
#define usbhs_for_each_pipe_with_dcp(pos, priv, i) \
|
|
__usbhs_for_each_pipe(0, pos, &((priv)->pipe_info), i)
|
|
|
|
/*
|
|
* data
|
|
*/
|
|
#define usbhs_priv_to_pipeinfo(pr) (&(pr)->pipe_info)
|
|
|
|
/*
|
|
* pipe control
|
|
*/
|
|
char *usbhs_pipe_name(struct usbhs_pipe *pipe);
|
|
struct usbhs_pipe
|
|
*usbhs_pipe_malloc(struct usbhs_priv *priv, int endpoint_type, int dir_in);
|
|
int usbhs_pipe_probe(struct usbhs_priv *priv);
|
|
void usbhs_pipe_remove(struct usbhs_priv *priv);
|
|
int usbhs_pipe_is_dir_in(struct usbhs_pipe *pipe);
|
|
int usbhs_pipe_is_dir_host(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_init(struct usbhs_priv *priv,
|
|
int (*dma_map_ctrl)(struct usbhs_pkt *pkt, int map));
|
|
int usbhs_pipe_get_maxpacket(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_clear(struct usbhs_pipe *pipe);
|
|
int usbhs_pipe_is_accessible(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_enable(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_disable(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_stall(struct usbhs_pipe *pipe);
|
|
int usbhs_pipe_is_stall(struct usbhs_pipe *pipe);
|
|
void usbhs_pipe_set_trans_count_if_bulk(struct usbhs_pipe *pipe, int len);
|
|
void usbhs_pipe_select_fifo(struct usbhs_pipe *pipe, struct usbhs_fifo *fifo);
|
|
void usbhs_pipe_config_update(struct usbhs_pipe *pipe, u16 devsel,
|
|
u16 epnum, u16 maxp);
|
|
|
|
#define usbhs_pipe_sequence_data0(pipe) usbhs_pipe_data_sequence(pipe, 0)
|
|
#define usbhs_pipe_sequence_data1(pipe) usbhs_pipe_data_sequence(pipe, 1)
|
|
void usbhs_pipe_data_sequence(struct usbhs_pipe *pipe, int data);
|
|
|
|
#define usbhs_pipe_to_priv(p) ((p)->priv)
|
|
#define usbhs_pipe_number(p) (int)((p) - (p)->priv->pipe_info.pipe)
|
|
#define usbhs_pipe_is_dcp(p) ((p)->priv->pipe_info.pipe == (p))
|
|
#define usbhs_pipe_to_fifo(p) ((p)->fifo)
|
|
#define usbhs_pipe_is_busy(p) usbhs_pipe_to_fifo(p)
|
|
|
|
#define usbhs_pipe_type(p) ((p)->pipe_type)
|
|
#define usbhs_pipe_type_is(p, t) ((p)->pipe_type == t)
|
|
|
|
/*
|
|
* dcp control
|
|
*/
|
|
struct usbhs_pipe *usbhs_dcp_malloc(struct usbhs_priv *priv);
|
|
void usbhs_dcp_control_transfer_done(struct usbhs_pipe *pipe);
|
|
void usbhs_dcp_dir_for_host(struct usbhs_pipe *pipe, int dir_out);
|
|
|
|
#endif /* RENESAS_USB_PIPE_H */
|