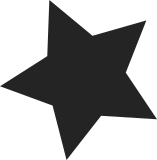
There is still some more work to do to disentangle map creation from DSO loading, but this happens only for the kernel, and for the early adopters of perf diff, where this disentanglement matters most, we'll be testing different kernels, so no problem here. Further clarification: right now we create the kernel maps for the various modules and discontiguous kernel text maps when loading the DSO, we should do it as a two step process, first creating the maps, for multiple mappings with the same DSO store, then doing the dso load just once, for the first hit on one of the maps sharing this DSO backing store. Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com> Cc: Frédéric Weisbecker <fweisbec@gmail.com> Cc: Mike Galbraith <efault@gmx.de> Cc: Peter Zijlstra <a.p.zijlstra@chello.nl> Cc: Paul Mackerras <paulus@samba.org> LKML-Reference: <1260741029-4430-6-git-send-email-acme@infradead.org> Signed-off-by: Ingo Molnar <mingo@elte.hu>
77 lines
1.8 KiB
C
77 lines
1.8 KiB
C
/*
|
|
* builtin-buildid-list.c
|
|
*
|
|
* Builtin buildid-list command: list buildids in perf.data
|
|
*
|
|
* Copyright (C) 2009, Red Hat Inc.
|
|
* Copyright (C) 2009, Arnaldo Carvalho de Melo <acme@redhat.com>
|
|
*/
|
|
#include "builtin.h"
|
|
#include "perf.h"
|
|
#include "util/cache.h"
|
|
#include "util/debug.h"
|
|
#include "util/parse-options.h"
|
|
#include "util/session.h"
|
|
#include "util/symbol.h"
|
|
|
|
static char const *input_name = "perf.data";
|
|
static int force;
|
|
|
|
static const char *const buildid_list_usage[] = {
|
|
"perf buildid-list [<options>]",
|
|
NULL
|
|
};
|
|
|
|
static const struct option options[] = {
|
|
OPT_STRING('i', "input", &input_name, "file",
|
|
"input file name"),
|
|
OPT_BOOLEAN('f', "force", &force, "don't complain, do it"),
|
|
OPT_BOOLEAN('v', "verbose", &verbose,
|
|
"be more verbose"),
|
|
OPT_END()
|
|
};
|
|
|
|
static int perf_file_section__process_buildids(struct perf_file_section *self,
|
|
int feat, int fd)
|
|
{
|
|
if (feat != HEADER_BUILD_ID)
|
|
return 0;
|
|
|
|
if (lseek(fd, self->offset, SEEK_SET) < 0) {
|
|
pr_warning("Failed to lseek to %Ld offset for buildids!\n",
|
|
self->offset);
|
|
return -1;
|
|
}
|
|
|
|
if (perf_header__read_build_ids(fd, self->offset, self->size)) {
|
|
pr_warning("Failed to read buildids!\n");
|
|
return -1;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int __cmd_buildid_list(void)
|
|
{
|
|
int err = -1;
|
|
struct perf_session *session = perf_session__new(input_name, O_RDONLY,
|
|
force, NULL);
|
|
if (session == NULL)
|
|
return -1;
|
|
|
|
err = perf_header__process_sections(&session->header, session->fd,
|
|
perf_file_section__process_buildids);
|
|
if (err >= 0)
|
|
dsos__fprintf_buildid(stdout);
|
|
|
|
perf_session__delete(session);
|
|
return err;
|
|
}
|
|
|
|
int cmd_buildid_list(int argc, const char **argv, const char *prefix __used)
|
|
{
|
|
argc = parse_options(argc, argv, options, buildid_list_usage, 0);
|
|
setup_pager();
|
|
return __cmd_buildid_list();
|
|
}
|