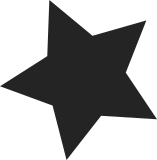
This patch modifies the GRO stack to avoid the use of "network_header" and associated macros like ip_hdr() and ipv6_hdr() in order to allow an arbitary number of IP hdrs (v4 or v6) to be used in the encapsulation chain. This lays the foundation for various IP tunneling support (IP-in-IP, GRE, VXLAN, SIT,...) to be added later. With this patch, the GRO stack traversing now is mostly based on skb_gro_offset rather than special hdr offsets saved in skb (e.g., skb->network_header). As a result all but the top layer (i.e., the the transport layer) must have hdrs of the same length in order for a pkt to be considered for aggregation. Therefore when adding a new encap layer (e.g., for tunneling), one must check and skip flows (e.g., by setting NAPI_GRO_CB(p)->same_flow to 0) that have a different hdr length. Note that unlike the network header, the transport header can and will continue to be set by the GRO code since there will be at most one "transport layer" in the encap chain. Signed-off-by: H.K. Jerry Chu <hkchu@google.com> Suggested-by: Eric Dumazet <edumazet@google.com> Reviewed-by: Eric Dumazet <edumazet@google.com> Signed-off-by: David S. Miller <davem@davemloft.net>
94 lines
2.1 KiB
C
94 lines
2.1 KiB
C
/*
|
|
* IPV6 GSO/GRO offload support
|
|
* Linux INET6 implementation
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*
|
|
* TCPv6 GSO/GRO support
|
|
*/
|
|
#include <linux/skbuff.h>
|
|
#include <net/protocol.h>
|
|
#include <net/tcp.h>
|
|
#include <net/ip6_checksum.h>
|
|
#include "ip6_offload.h"
|
|
|
|
static int tcp_v6_gso_send_check(struct sk_buff *skb)
|
|
{
|
|
const struct ipv6hdr *ipv6h;
|
|
struct tcphdr *th;
|
|
|
|
if (!pskb_may_pull(skb, sizeof(*th)))
|
|
return -EINVAL;
|
|
|
|
ipv6h = ipv6_hdr(skb);
|
|
th = tcp_hdr(skb);
|
|
|
|
th->check = 0;
|
|
skb->ip_summed = CHECKSUM_PARTIAL;
|
|
__tcp_v6_send_check(skb, &ipv6h->saddr, &ipv6h->daddr);
|
|
return 0;
|
|
}
|
|
|
|
static struct sk_buff **tcp6_gro_receive(struct sk_buff **head,
|
|
struct sk_buff *skb)
|
|
{
|
|
const struct ipv6hdr *iph = skb_gro_network_header(skb);
|
|
__wsum wsum;
|
|
|
|
/* Don't bother verifying checksum if we're going to flush anyway. */
|
|
if (NAPI_GRO_CB(skb)->flush)
|
|
goto skip_csum;
|
|
|
|
wsum = skb->csum;
|
|
|
|
switch (skb->ip_summed) {
|
|
case CHECKSUM_NONE:
|
|
wsum = skb_checksum(skb, skb_gro_offset(skb), skb_gro_len(skb),
|
|
wsum);
|
|
|
|
/* fall through */
|
|
|
|
case CHECKSUM_COMPLETE:
|
|
if (!tcp_v6_check(skb_gro_len(skb), &iph->saddr, &iph->daddr,
|
|
wsum)) {
|
|
skb->ip_summed = CHECKSUM_UNNECESSARY;
|
|
break;
|
|
}
|
|
|
|
NAPI_GRO_CB(skb)->flush = 1;
|
|
return NULL;
|
|
}
|
|
|
|
skip_csum:
|
|
return tcp_gro_receive(head, skb);
|
|
}
|
|
|
|
static int tcp6_gro_complete(struct sk_buff *skb, int thoff)
|
|
{
|
|
const struct ipv6hdr *iph = ipv6_hdr(skb);
|
|
struct tcphdr *th = tcp_hdr(skb);
|
|
|
|
th->check = ~tcp_v6_check(skb->len - thoff, &iph->saddr,
|
|
&iph->daddr, 0);
|
|
skb_shinfo(skb)->gso_type = SKB_GSO_TCPV6;
|
|
|
|
return tcp_gro_complete(skb);
|
|
}
|
|
|
|
static const struct net_offload tcpv6_offload = {
|
|
.callbacks = {
|
|
.gso_send_check = tcp_v6_gso_send_check,
|
|
.gso_segment = tcp_gso_segment,
|
|
.gro_receive = tcp6_gro_receive,
|
|
.gro_complete = tcp6_gro_complete,
|
|
},
|
|
};
|
|
|
|
int __init tcpv6_offload_init(void)
|
|
{
|
|
return inet6_add_offload(&tcpv6_offload, IPPROTO_TCP);
|
|
}
|