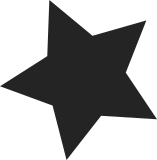
Rename the following functions, which are shorter and more in line with common naming practice in the network subsystem. tipc_bclink_send_msg->tipc_bclink_xmit tipc_bclink_recv_pkt->tipc_bclink_rcv tipc_disc_recv_msg->tipc_disc_rcv tipc_link_send_proto_msg->tipc_link_proto_xmit link_recv_proto_msg->tipc_link_proto_rcv link_send_sections_long->tipc_link_iovec_long_xmit tipc_link_send_sections_fast->tipc_link_iovec_xmit_fast tipc_link_send_sync->tipc_link_sync_xmit tipc_link_recv_sync->tipc_link_sync_rcv tipc_link_send_buf->__tipc_link_xmit tipc_link_send->tipc_link_xmit tipc_link_send_names->tipc_link_names_xmit tipc_named_recv->tipc_named_rcv tipc_link_recv_bundle->tipc_link_bundle_rcv tipc_link_dup_send_queue->tipc_link_dup_queue_xmit link_send_long_buf->tipc_link_frag_xmit tipc_multicast->tipc_port_mcast_xmit tipc_port_recv_mcast->tipc_port_mcast_rcv tipc_port_reject_sections->tipc_port_iovec_reject tipc_port_recv_proto_msg->tipc_port_proto_rcv tipc_connect->tipc_port_connect __tipc_connect->__tipc_port_connect __tipc_disconnect->__tipc_port_disconnect tipc_disconnect->tipc_port_disconnect tipc_shutdown->tipc_port_shutdown tipc_port_recv_msg->tipc_port_rcv tipc_port_recv_sections->tipc_port_iovec_rcv release->tipc_release accept->tipc_accept bind->tipc_bind get_name->tipc_getname poll->tipc_poll send_msg->tipc_sendmsg send_packet->tipc_send_packet send_stream->tipc_send_stream recv_msg->tipc_recvmsg recv_stream->tipc_recv_stream connect->tipc_connect listen->tipc_listen shutdown->tipc_shutdown setsockopt->tipc_setsockopt getsockopt->tipc_getsockopt Above changes have no impact on current users of the functions. Signed-off-by: Ying Xue <ying.xue@windriver.com> Reviewed-by: Jon Maloy <jon.maloy@ericsson.com> Signed-off-by: David S. Miller <davem@davemloft.net>
104 lines
3.6 KiB
C
104 lines
3.6 KiB
C
/*
|
|
* net/tipc/bcast.h: Include file for TIPC broadcast code
|
|
*
|
|
* Copyright (c) 2003-2006, Ericsson AB
|
|
* Copyright (c) 2005, 2010-2011, Wind River Systems
|
|
* All rights reserved.
|
|
*
|
|
* Redistribution and use in source and binary forms, with or without
|
|
* modification, are permitted provided that the following conditions are met:
|
|
*
|
|
* 1. Redistributions of source code must retain the above copyright
|
|
* notice, this list of conditions and the following disclaimer.
|
|
* 2. Redistributions in binary form must reproduce the above copyright
|
|
* notice, this list of conditions and the following disclaimer in the
|
|
* documentation and/or other materials provided with the distribution.
|
|
* 3. Neither the names of the copyright holders nor the names of its
|
|
* contributors may be used to endorse or promote products derived from
|
|
* this software without specific prior written permission.
|
|
*
|
|
* Alternatively, this software may be distributed under the terms of the
|
|
* GNU General Public License ("GPL") version 2 as published by the Free
|
|
* Software Foundation.
|
|
*
|
|
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
|
|
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
|
|
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
|
|
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
|
|
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
|
|
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
|
|
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
|
|
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
|
|
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
|
|
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
|
|
* POSSIBILITY OF SUCH DAMAGE.
|
|
*/
|
|
|
|
#ifndef _TIPC_BCAST_H
|
|
#define _TIPC_BCAST_H
|
|
|
|
#define MAX_NODES 4096
|
|
#define WSIZE 32
|
|
|
|
/**
|
|
* struct tipc_node_map - set of node identifiers
|
|
* @count: # of nodes in set
|
|
* @map: bitmap of node identifiers that are in the set
|
|
*/
|
|
struct tipc_node_map {
|
|
u32 count;
|
|
u32 map[MAX_NODES / WSIZE];
|
|
};
|
|
|
|
#define PLSIZE 32
|
|
|
|
/**
|
|
* struct tipc_port_list - set of node local destination ports
|
|
* @count: # of ports in set (only valid for first entry in list)
|
|
* @next: pointer to next entry in list
|
|
* @ports: array of port references
|
|
*/
|
|
struct tipc_port_list {
|
|
int count;
|
|
struct tipc_port_list *next;
|
|
u32 ports[PLSIZE];
|
|
};
|
|
|
|
|
|
struct tipc_node;
|
|
|
|
extern const char tipc_bclink_name[];
|
|
|
|
void tipc_nmap_add(struct tipc_node_map *nm_ptr, u32 node);
|
|
void tipc_nmap_remove(struct tipc_node_map *nm_ptr, u32 node);
|
|
|
|
/**
|
|
* tipc_nmap_equal - test for equality of node maps
|
|
*/
|
|
static inline int tipc_nmap_equal(struct tipc_node_map *nm_a,
|
|
struct tipc_node_map *nm_b)
|
|
{
|
|
return !memcmp(nm_a, nm_b, sizeof(*nm_a));
|
|
}
|
|
|
|
void tipc_port_list_add(struct tipc_port_list *pl_ptr, u32 port);
|
|
void tipc_port_list_free(struct tipc_port_list *pl_ptr);
|
|
|
|
void tipc_bclink_init(void);
|
|
void tipc_bclink_stop(void);
|
|
void tipc_bclink_add_node(u32 addr);
|
|
void tipc_bclink_remove_node(u32 addr);
|
|
struct tipc_node *tipc_bclink_retransmit_to(void);
|
|
void tipc_bclink_acknowledge(struct tipc_node *n_ptr, u32 acked);
|
|
int tipc_bclink_xmit(struct sk_buff *buf);
|
|
void tipc_bclink_rcv(struct sk_buff *buf);
|
|
u32 tipc_bclink_get_last_sent(void);
|
|
u32 tipc_bclink_acks_missing(struct tipc_node *n_ptr);
|
|
void tipc_bclink_update_link_state(struct tipc_node *n_ptr, u32 last_sent);
|
|
int tipc_bclink_stats(char *stats_buf, const u32 buf_size);
|
|
int tipc_bclink_reset_stats(void);
|
|
int tipc_bclink_set_queue_limits(u32 limit);
|
|
void tipc_bcbearer_sort(void);
|
|
|
|
#endif
|