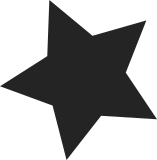
The NAT range to nlattr conversation callbacks and helpers are entirely dead code and are also useless since there are no NAT ranges in conntrack context, they are only used for initially selecting a tuple. The final NAT information is contained in the selected tuples of the conntrack entry. Signed-off-by: Patrick McHardy <kaber@trash.net> Signed-off-by: Pablo Neira Ayuso <pablo@netfilter.org>
92 lines
2.6 KiB
C
92 lines
2.6 KiB
C
/* (C) 1999-2001 Paul `Rusty' Russell
|
|
* (C) 2002-2006 Netfilter Core Team <coreteam@netfilter.org>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 as
|
|
* published by the Free Software Foundation.
|
|
*/
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/init.h>
|
|
#include <linux/export.h>
|
|
#include <linux/ip.h>
|
|
#include <linux/tcp.h>
|
|
|
|
#include <linux/netfilter.h>
|
|
#include <linux/netfilter/nfnetlink_conntrack.h>
|
|
#include <net/netfilter/nf_nat.h>
|
|
#include <net/netfilter/nf_nat_rule.h>
|
|
#include <net/netfilter/nf_nat_protocol.h>
|
|
#include <net/netfilter/nf_nat_core.h>
|
|
|
|
static u_int16_t tcp_port_rover;
|
|
|
|
static void
|
|
tcp_unique_tuple(struct nf_conntrack_tuple *tuple,
|
|
const struct nf_nat_ipv4_range *range,
|
|
enum nf_nat_manip_type maniptype,
|
|
const struct nf_conn *ct)
|
|
{
|
|
nf_nat_proto_unique_tuple(tuple, range, maniptype, ct, &tcp_port_rover);
|
|
}
|
|
|
|
static bool
|
|
tcp_manip_pkt(struct sk_buff *skb,
|
|
unsigned int iphdroff,
|
|
const struct nf_conntrack_tuple *tuple,
|
|
enum nf_nat_manip_type maniptype)
|
|
{
|
|
const struct iphdr *iph = (struct iphdr *)(skb->data + iphdroff);
|
|
struct tcphdr *hdr;
|
|
unsigned int hdroff = iphdroff + iph->ihl*4;
|
|
__be32 oldip, newip;
|
|
__be16 *portptr, newport, oldport;
|
|
int hdrsize = 8; /* TCP connection tracking guarantees this much */
|
|
|
|
/* this could be a inner header returned in icmp packet; in such
|
|
cases we cannot update the checksum field since it is outside of
|
|
the 8 bytes of transport layer headers we are guaranteed */
|
|
if (skb->len >= hdroff + sizeof(struct tcphdr))
|
|
hdrsize = sizeof(struct tcphdr);
|
|
|
|
if (!skb_make_writable(skb, hdroff + hdrsize))
|
|
return false;
|
|
|
|
iph = (struct iphdr *)(skb->data + iphdroff);
|
|
hdr = (struct tcphdr *)(skb->data + hdroff);
|
|
|
|
if (maniptype == NF_NAT_MANIP_SRC) {
|
|
/* Get rid of src ip and src pt */
|
|
oldip = iph->saddr;
|
|
newip = tuple->src.u3.ip;
|
|
newport = tuple->src.u.tcp.port;
|
|
portptr = &hdr->source;
|
|
} else {
|
|
/* Get rid of dst ip and dst pt */
|
|
oldip = iph->daddr;
|
|
newip = tuple->dst.u3.ip;
|
|
newport = tuple->dst.u.tcp.port;
|
|
portptr = &hdr->dest;
|
|
}
|
|
|
|
oldport = *portptr;
|
|
*portptr = newport;
|
|
|
|
if (hdrsize < sizeof(*hdr))
|
|
return true;
|
|
|
|
inet_proto_csum_replace4(&hdr->check, skb, oldip, newip, 1);
|
|
inet_proto_csum_replace2(&hdr->check, skb, oldport, newport, 0);
|
|
return true;
|
|
}
|
|
|
|
const struct nf_nat_protocol nf_nat_protocol_tcp = {
|
|
.protonum = IPPROTO_TCP,
|
|
.manip_pkt = tcp_manip_pkt,
|
|
.in_range = nf_nat_proto_in_range,
|
|
.unique_tuple = tcp_unique_tuple,
|
|
#if defined(CONFIG_NF_CT_NETLINK) || defined(CONFIG_NF_CT_NETLINK_MODULE)
|
|
.nlattr_to_range = nf_nat_proto_nlattr_to_range,
|
|
#endif
|
|
};
|