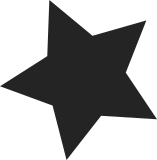
On MIPv6 usage, XFRM sub policy is enabled. When main (IPsec) and sub (MIPv6) policy selectors have the same address set but different upper layer information (i.e. protocol number and its ports or type/code), multiple bundle should be created. However, currently we have issue to use the same bundle created for the first time with all flows covered by the case. It is useful for the bundle to have the upper layer information to be restructured correctly if it does not match with the flow. 1. Bundle was created by two policies Selector from another policy is added to xfrm_dst. If the flow does not match the selector, it goes to slow path to restructure new bundle by single policy. 2. Bundle was created by one policy Flow cache is added to xfrm_dst as originated one. If the flow does not match the cache, it goes to slow path to try searching another policy. Signed-off-by: Masahide NAKAMURA <nakam@linux-ipv6.org> Signed-off-by: David S. Miller <davem@davemloft.net>
107 lines
2 KiB
C
107 lines
2 KiB
C
/*
|
|
*
|
|
* Generic internet FLOW.
|
|
*
|
|
*/
|
|
|
|
#ifndef _NET_FLOW_H
|
|
#define _NET_FLOW_H
|
|
|
|
#include <linux/in6.h>
|
|
#include <asm/atomic.h>
|
|
|
|
struct flowi {
|
|
int oif;
|
|
int iif;
|
|
__u32 mark;
|
|
|
|
union {
|
|
struct {
|
|
__be32 daddr;
|
|
__be32 saddr;
|
|
__u8 tos;
|
|
__u8 scope;
|
|
} ip4_u;
|
|
|
|
struct {
|
|
struct in6_addr daddr;
|
|
struct in6_addr saddr;
|
|
__be32 flowlabel;
|
|
} ip6_u;
|
|
|
|
struct {
|
|
__le16 daddr;
|
|
__le16 saddr;
|
|
__u8 scope;
|
|
} dn_u;
|
|
} nl_u;
|
|
#define fld_dst nl_u.dn_u.daddr
|
|
#define fld_src nl_u.dn_u.saddr
|
|
#define fld_scope nl_u.dn_u.scope
|
|
#define fl6_dst nl_u.ip6_u.daddr
|
|
#define fl6_src nl_u.ip6_u.saddr
|
|
#define fl6_flowlabel nl_u.ip6_u.flowlabel
|
|
#define fl4_dst nl_u.ip4_u.daddr
|
|
#define fl4_src nl_u.ip4_u.saddr
|
|
#define fl4_tos nl_u.ip4_u.tos
|
|
#define fl4_scope nl_u.ip4_u.scope
|
|
|
|
__u8 proto;
|
|
__u8 flags;
|
|
#define FLOWI_FLAG_MULTIPATHOLDROUTE 0x01
|
|
union {
|
|
struct {
|
|
__be16 sport;
|
|
__be16 dport;
|
|
} ports;
|
|
|
|
struct {
|
|
__u8 type;
|
|
__u8 code;
|
|
} icmpt;
|
|
|
|
struct {
|
|
__le16 sport;
|
|
__le16 dport;
|
|
} dnports;
|
|
|
|
__be32 spi;
|
|
|
|
#ifdef CONFIG_IPV6_MIP6
|
|
struct {
|
|
__u8 type;
|
|
} mht;
|
|
#endif
|
|
} uli_u;
|
|
#define fl_ip_sport uli_u.ports.sport
|
|
#define fl_ip_dport uli_u.ports.dport
|
|
#define fl_icmp_type uli_u.icmpt.type
|
|
#define fl_icmp_code uli_u.icmpt.code
|
|
#define fl_ipsec_spi uli_u.spi
|
|
#ifdef CONFIG_IPV6_MIP6
|
|
#define fl_mh_type uli_u.mht.type
|
|
#endif
|
|
__u32 secid; /* used by xfrm; see secid.txt */
|
|
} __attribute__((__aligned__(BITS_PER_LONG/8)));
|
|
|
|
#define FLOW_DIR_IN 0
|
|
#define FLOW_DIR_OUT 1
|
|
#define FLOW_DIR_FWD 2
|
|
|
|
struct sock;
|
|
typedef int (*flow_resolve_t)(struct flowi *key, u16 family, u8 dir,
|
|
void **objp, atomic_t **obj_refp);
|
|
|
|
extern void *flow_cache_lookup(struct flowi *key, u16 family, u8 dir,
|
|
flow_resolve_t resolver);
|
|
extern void flow_cache_flush(void);
|
|
extern atomic_t flow_cache_genid;
|
|
|
|
static inline int flow_cache_uli_match(struct flowi *fl1, struct flowi *fl2)
|
|
{
|
|
return (fl1->proto == fl2->proto &&
|
|
!memcmp(&fl1->uli_u, &fl2->uli_u, sizeof(fl1->uli_u)));
|
|
}
|
|
|
|
#endif
|