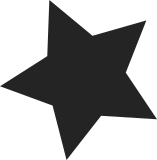
Xiaohui Xin and some other folks at Intel have been looking into what's
behind the performance hit of paravirt_ops when running native.
It appears that the hit is entirely due to the paravirtualized
spinlocks introduced by:
| commit 8efcbab674
| Date: Mon Jul 7 12:07:51 2008 -0700
|
| paravirt: introduce a "lock-byte" spinlock implementation
The extra call/return in the spinlock path is somehow
causing an increase in the cycles/instruction of somewhere around 2-7%
(seems to vary quite a lot from test to test). The working theory is
that the CPU's pipeline is getting upset about the
call->call->locked-op->return->return, and seems to be failing to
speculate (though I haven't seen anything definitive about the precise
reasons). This doesn't entirely make sense, because the performance
hit is also visible on unlock and other operations which don't involve
locked instructions. But spinlock operations clearly swamp all the
other pvops operations, even though I can't imagine that they're
nearly as common (there's only a .05% increase in instructions
executed).
If I disable just the pv-spinlock calls, my tests show that pvops is
identical to non-pvops performance on native (my measurements show that
it is actually about .1% faster, but Xiaohui shows a .05% slowdown).
Summary of results, averaging 10 runs of the "mmperf" test, using a
no-pvops build as baseline:
nopv Pv-nospin Pv-spin
CPU cycles 100.00% 99.89% 102.18%
instructions 100.00% 100.10% 100.15%
CPI 100.00% 99.79% 102.03%
cache ref 100.00% 100.84% 100.28%
cache miss 100.00% 90.47% 88.56%
cache miss rate 100.00% 89.72% 88.31%
branches 100.00% 99.93% 100.04%
branch miss 100.00% 103.66% 107.72%
branch miss rt 100.00% 103.73% 107.67%
wallclock 100.00% 99.90% 102.20%
The clear effect here is that the 2% increase in CPI is
directly reflected in the final wallclock time.
(The other interesting effect is that the more ops are
out of line calls via pvops, the lower the cache access
and miss rates. Not too surprising, but it suggests that
the non-pvops kernel is over-inlined. On the flipside,
the branch misses go up correspondingly...)
So, what's the fix?
Paravirt patching turns all the pvops calls into direct calls, so
_spin_lock etc do end up having direct calls. For example, the compiler
generated code for paravirtualized _spin_lock is:
<_spin_lock+0>: mov %gs:0xb4c8,%rax
<_spin_lock+9>: incl 0xffffffffffffe044(%rax)
<_spin_lock+15>: callq *0xffffffff805a5b30
<_spin_lock+22>: retq
The indirect call will get patched to:
<_spin_lock+0>: mov %gs:0xb4c8,%rax
<_spin_lock+9>: incl 0xffffffffffffe044(%rax)
<_spin_lock+15>: callq <__ticket_spin_lock>
<_spin_lock+20>: nop; nop /* or whatever 2-byte nop */
<_spin_lock+22>: retq
One possibility is to inline _spin_lock, etc, when building an
optimised kernel (ie, when there's no spinlock/preempt
instrumentation/debugging enabled). That will remove the outer
call/return pair, returning the instruction stream to a single
call/return, which will presumably execute the same as the non-pvops
case. The downsides arel 1) it will replicate the
preempt_disable/enable code at eack lock/unlock callsite; this code is
fairly small, but not nothing; and 2) the spinlock definitions are
already a very heavily tangled mass of #ifdefs and other preprocessor
magic, and making any changes will be non-trivial.
The other obvious answer is to disable pv-spinlocks. Making them a
separate config option is fairly easy, and it would be trivial to
enable them only when Xen is enabled (as the only non-default user).
But it doesn't really address the common case of a distro build which
is going to have Xen support enabled, and leaves the open question of
whether the native performance cost of pv-spinlocks is worth the
performance improvement on a loaded Xen system (10% saving of overall
system CPU when guests block rather than spin). Still it is a
reasonable short-term workaround.
[ Impact: fix pvops performance regression when running native ]
Analysed-by: "Xin Xiaohui" <xiaohui.xin@intel.com>
Analysed-by: "Li Xin" <xin.li@intel.com>
Analysed-by: "Nakajima Jun" <jun.nakajima@intel.com>
Signed-off-by: Jeremy Fitzhardinge <jeremy.fitzhardinge@citrix.com>
Acked-by: H. Peter Anvin <hpa@zytor.com>
Cc: Nick Piggin <npiggin@suse.de>
Cc: Xen-devel <xen-devel@lists.xensource.com>
LKML-Reference: <4A0B62F7.5030802@goop.org>
[ fixed the help text ]
Signed-off-by: Ingo Molnar <mingo@elte.hu>
306 lines
7.5 KiB
C
306 lines
7.5 KiB
C
#ifndef _ASM_X86_SPINLOCK_H
|
|
#define _ASM_X86_SPINLOCK_H
|
|
|
|
#include <asm/atomic.h>
|
|
#include <asm/rwlock.h>
|
|
#include <asm/page.h>
|
|
#include <asm/processor.h>
|
|
#include <linux/compiler.h>
|
|
#include <asm/paravirt.h>
|
|
/*
|
|
* Your basic SMP spinlocks, allowing only a single CPU anywhere
|
|
*
|
|
* Simple spin lock operations. There are two variants, one clears IRQ's
|
|
* on the local processor, one does not.
|
|
*
|
|
* These are fair FIFO ticket locks, which are currently limited to 256
|
|
* CPUs.
|
|
*
|
|
* (the type definitions are in asm/spinlock_types.h)
|
|
*/
|
|
|
|
#ifdef CONFIG_X86_32
|
|
# define LOCK_PTR_REG "a"
|
|
# define REG_PTR_MODE "k"
|
|
#else
|
|
# define LOCK_PTR_REG "D"
|
|
# define REG_PTR_MODE "q"
|
|
#endif
|
|
|
|
#if defined(CONFIG_X86_32) && \
|
|
(defined(CONFIG_X86_OOSTORE) || defined(CONFIG_X86_PPRO_FENCE))
|
|
/*
|
|
* On PPro SMP or if we are using OOSTORE, we use a locked operation to unlock
|
|
* (PPro errata 66, 92)
|
|
*/
|
|
# define UNLOCK_LOCK_PREFIX LOCK_PREFIX
|
|
#else
|
|
# define UNLOCK_LOCK_PREFIX
|
|
#endif
|
|
|
|
/*
|
|
* Ticket locks are conceptually two parts, one indicating the current head of
|
|
* the queue, and the other indicating the current tail. The lock is acquired
|
|
* by atomically noting the tail and incrementing it by one (thus adding
|
|
* ourself to the queue and noting our position), then waiting until the head
|
|
* becomes equal to the the initial value of the tail.
|
|
*
|
|
* We use an xadd covering *both* parts of the lock, to increment the tail and
|
|
* also load the position of the head, which takes care of memory ordering
|
|
* issues and should be optimal for the uncontended case. Note the tail must be
|
|
* in the high part, because a wide xadd increment of the low part would carry
|
|
* up and contaminate the high part.
|
|
*
|
|
* With fewer than 2^8 possible CPUs, we can use x86's partial registers to
|
|
* save some instructions and make the code more elegant. There really isn't
|
|
* much between them in performance though, especially as locks are out of line.
|
|
*/
|
|
#if (NR_CPUS < 256)
|
|
#define TICKET_SHIFT 8
|
|
|
|
static __always_inline void __ticket_spin_lock(raw_spinlock_t *lock)
|
|
{
|
|
short inc = 0x0100;
|
|
|
|
asm volatile (
|
|
LOCK_PREFIX "xaddw %w0, %1\n"
|
|
"1:\t"
|
|
"cmpb %h0, %b0\n\t"
|
|
"je 2f\n\t"
|
|
"rep ; nop\n\t"
|
|
"movb %1, %b0\n\t"
|
|
/* don't need lfence here, because loads are in-order */
|
|
"jmp 1b\n"
|
|
"2:"
|
|
: "+Q" (inc), "+m" (lock->slock)
|
|
:
|
|
: "memory", "cc");
|
|
}
|
|
|
|
static __always_inline int __ticket_spin_trylock(raw_spinlock_t *lock)
|
|
{
|
|
int tmp, new;
|
|
|
|
asm volatile("movzwl %2, %0\n\t"
|
|
"cmpb %h0,%b0\n\t"
|
|
"leal 0x100(%" REG_PTR_MODE "0), %1\n\t"
|
|
"jne 1f\n\t"
|
|
LOCK_PREFIX "cmpxchgw %w1,%2\n\t"
|
|
"1:"
|
|
"sete %b1\n\t"
|
|
"movzbl %b1,%0\n\t"
|
|
: "=&a" (tmp), "=&q" (new), "+m" (lock->slock)
|
|
:
|
|
: "memory", "cc");
|
|
|
|
return tmp;
|
|
}
|
|
|
|
static __always_inline void __ticket_spin_unlock(raw_spinlock_t *lock)
|
|
{
|
|
asm volatile(UNLOCK_LOCK_PREFIX "incb %0"
|
|
: "+m" (lock->slock)
|
|
:
|
|
: "memory", "cc");
|
|
}
|
|
#else
|
|
#define TICKET_SHIFT 16
|
|
|
|
static __always_inline void __ticket_spin_lock(raw_spinlock_t *lock)
|
|
{
|
|
int inc = 0x00010000;
|
|
int tmp;
|
|
|
|
asm volatile(LOCK_PREFIX "xaddl %0, %1\n"
|
|
"movzwl %w0, %2\n\t"
|
|
"shrl $16, %0\n\t"
|
|
"1:\t"
|
|
"cmpl %0, %2\n\t"
|
|
"je 2f\n\t"
|
|
"rep ; nop\n\t"
|
|
"movzwl %1, %2\n\t"
|
|
/* don't need lfence here, because loads are in-order */
|
|
"jmp 1b\n"
|
|
"2:"
|
|
: "+r" (inc), "+m" (lock->slock), "=&r" (tmp)
|
|
:
|
|
: "memory", "cc");
|
|
}
|
|
|
|
static __always_inline int __ticket_spin_trylock(raw_spinlock_t *lock)
|
|
{
|
|
int tmp;
|
|
int new;
|
|
|
|
asm volatile("movl %2,%0\n\t"
|
|
"movl %0,%1\n\t"
|
|
"roll $16, %0\n\t"
|
|
"cmpl %0,%1\n\t"
|
|
"leal 0x00010000(%" REG_PTR_MODE "0), %1\n\t"
|
|
"jne 1f\n\t"
|
|
LOCK_PREFIX "cmpxchgl %1,%2\n\t"
|
|
"1:"
|
|
"sete %b1\n\t"
|
|
"movzbl %b1,%0\n\t"
|
|
: "=&a" (tmp), "=&q" (new), "+m" (lock->slock)
|
|
:
|
|
: "memory", "cc");
|
|
|
|
return tmp;
|
|
}
|
|
|
|
static __always_inline void __ticket_spin_unlock(raw_spinlock_t *lock)
|
|
{
|
|
asm volatile(UNLOCK_LOCK_PREFIX "incw %0"
|
|
: "+m" (lock->slock)
|
|
:
|
|
: "memory", "cc");
|
|
}
|
|
#endif
|
|
|
|
static inline int __ticket_spin_is_locked(raw_spinlock_t *lock)
|
|
{
|
|
int tmp = ACCESS_ONCE(lock->slock);
|
|
|
|
return !!(((tmp >> TICKET_SHIFT) ^ tmp) & ((1 << TICKET_SHIFT) - 1));
|
|
}
|
|
|
|
static inline int __ticket_spin_is_contended(raw_spinlock_t *lock)
|
|
{
|
|
int tmp = ACCESS_ONCE(lock->slock);
|
|
|
|
return (((tmp >> TICKET_SHIFT) - tmp) & ((1 << TICKET_SHIFT) - 1)) > 1;
|
|
}
|
|
|
|
#ifndef CONFIG_PARAVIRT_SPINLOCKS
|
|
|
|
static inline int __raw_spin_is_locked(raw_spinlock_t *lock)
|
|
{
|
|
return __ticket_spin_is_locked(lock);
|
|
}
|
|
|
|
static inline int __raw_spin_is_contended(raw_spinlock_t *lock)
|
|
{
|
|
return __ticket_spin_is_contended(lock);
|
|
}
|
|
#define __raw_spin_is_contended __raw_spin_is_contended
|
|
|
|
static __always_inline void __raw_spin_lock(raw_spinlock_t *lock)
|
|
{
|
|
__ticket_spin_lock(lock);
|
|
}
|
|
|
|
static __always_inline int __raw_spin_trylock(raw_spinlock_t *lock)
|
|
{
|
|
return __ticket_spin_trylock(lock);
|
|
}
|
|
|
|
static __always_inline void __raw_spin_unlock(raw_spinlock_t *lock)
|
|
{
|
|
__ticket_spin_unlock(lock);
|
|
}
|
|
|
|
static __always_inline void __raw_spin_lock_flags(raw_spinlock_t *lock,
|
|
unsigned long flags)
|
|
{
|
|
__raw_spin_lock(lock);
|
|
}
|
|
|
|
#endif /* CONFIG_PARAVIRT_SPINLOCKS */
|
|
|
|
static inline void __raw_spin_unlock_wait(raw_spinlock_t *lock)
|
|
{
|
|
while (__raw_spin_is_locked(lock))
|
|
cpu_relax();
|
|
}
|
|
|
|
/*
|
|
* Read-write spinlocks, allowing multiple readers
|
|
* but only one writer.
|
|
*
|
|
* NOTE! it is quite common to have readers in interrupts
|
|
* but no interrupt writers. For those circumstances we
|
|
* can "mix" irq-safe locks - any writer needs to get a
|
|
* irq-safe write-lock, but readers can get non-irqsafe
|
|
* read-locks.
|
|
*
|
|
* On x86, we implement read-write locks as a 32-bit counter
|
|
* with the high bit (sign) being the "contended" bit.
|
|
*/
|
|
|
|
/**
|
|
* read_can_lock - would read_trylock() succeed?
|
|
* @lock: the rwlock in question.
|
|
*/
|
|
static inline int __raw_read_can_lock(raw_rwlock_t *lock)
|
|
{
|
|
return (int)(lock)->lock > 0;
|
|
}
|
|
|
|
/**
|
|
* write_can_lock - would write_trylock() succeed?
|
|
* @lock: the rwlock in question.
|
|
*/
|
|
static inline int __raw_write_can_lock(raw_rwlock_t *lock)
|
|
{
|
|
return (lock)->lock == RW_LOCK_BIAS;
|
|
}
|
|
|
|
static inline void __raw_read_lock(raw_rwlock_t *rw)
|
|
{
|
|
asm volatile(LOCK_PREFIX " subl $1,(%0)\n\t"
|
|
"jns 1f\n"
|
|
"call __read_lock_failed\n\t"
|
|
"1:\n"
|
|
::LOCK_PTR_REG (rw) : "memory");
|
|
}
|
|
|
|
static inline void __raw_write_lock(raw_rwlock_t *rw)
|
|
{
|
|
asm volatile(LOCK_PREFIX " subl %1,(%0)\n\t"
|
|
"jz 1f\n"
|
|
"call __write_lock_failed\n\t"
|
|
"1:\n"
|
|
::LOCK_PTR_REG (rw), "i" (RW_LOCK_BIAS) : "memory");
|
|
}
|
|
|
|
static inline int __raw_read_trylock(raw_rwlock_t *lock)
|
|
{
|
|
atomic_t *count = (atomic_t *)lock;
|
|
|
|
if (atomic_dec_return(count) >= 0)
|
|
return 1;
|
|
atomic_inc(count);
|
|
return 0;
|
|
}
|
|
|
|
static inline int __raw_write_trylock(raw_rwlock_t *lock)
|
|
{
|
|
atomic_t *count = (atomic_t *)lock;
|
|
|
|
if (atomic_sub_and_test(RW_LOCK_BIAS, count))
|
|
return 1;
|
|
atomic_add(RW_LOCK_BIAS, count);
|
|
return 0;
|
|
}
|
|
|
|
static inline void __raw_read_unlock(raw_rwlock_t *rw)
|
|
{
|
|
asm volatile(LOCK_PREFIX "incl %0" :"+m" (rw->lock) : : "memory");
|
|
}
|
|
|
|
static inline void __raw_write_unlock(raw_rwlock_t *rw)
|
|
{
|
|
asm volatile(LOCK_PREFIX "addl %1, %0"
|
|
: "+m" (rw->lock) : "i" (RW_LOCK_BIAS) : "memory");
|
|
}
|
|
|
|
#define __raw_read_lock_flags(lock, flags) __raw_read_lock(lock)
|
|
#define __raw_write_lock_flags(lock, flags) __raw_write_lock(lock)
|
|
|
|
#define _raw_spin_relax(lock) cpu_relax()
|
|
#define _raw_read_relax(lock) cpu_relax()
|
|
#define _raw_write_relax(lock) cpu_relax()
|
|
|
|
#endif /* _ASM_X86_SPINLOCK_H */
|